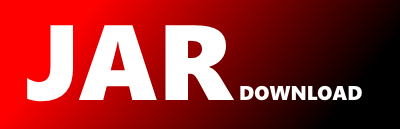
com.pulumi.azurenative.recoveryservices.inputs.AzureResourceProtectionIntentArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.inputs;
import com.pulumi.azurenative.recoveryservices.enums.BackupManagementType;
import com.pulumi.azurenative.recoveryservices.enums.ProtectionStatus;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* IaaS VM specific backup protection intent item.
*
*/
public final class AzureResourceProtectionIntentArgs extends com.pulumi.resources.ResourceArgs {
public static final AzureResourceProtectionIntentArgs Empty = new AzureResourceProtectionIntentArgs();
/**
* Type of backup management for the backed up item.
*
*/
@Import(name="backupManagementType")
private @Nullable Output> backupManagementType;
/**
* @return Type of backup management for the backed up item.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy