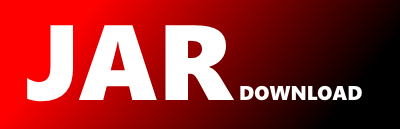
com.pulumi.azurenative.resources.inputs.DeploymentPropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.resources.inputs;
import com.pulumi.azurenative.resources.enums.DeploymentMode;
import com.pulumi.azurenative.resources.inputs.DebugSettingArgs;
import com.pulumi.azurenative.resources.inputs.DeploymentParameterArgs;
import com.pulumi.azurenative.resources.inputs.ExpressionEvaluationOptionsArgs;
import com.pulumi.azurenative.resources.inputs.OnErrorDeploymentArgs;
import com.pulumi.azurenative.resources.inputs.ParametersLinkArgs;
import com.pulumi.azurenative.resources.inputs.TemplateLinkArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Deployment properties.
*
*/
public final class DeploymentPropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final DeploymentPropertiesArgs Empty = new DeploymentPropertiesArgs();
/**
* The debug setting of the deployment.
*
*/
@Import(name="debugSetting")
private @Nullable Output debugSetting;
/**
* @return The debug setting of the deployment.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy