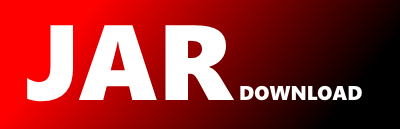
com.pulumi.azurenative.secretsynccontroller.SecretSync Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.secretsynccontroller;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.secretsynccontroller.SecretSyncArgs;
import com.pulumi.azurenative.secretsynccontroller.outputs.AzureResourceManagerCommonTypesExtendedLocationResponse;
import com.pulumi.azurenative.secretsynccontroller.outputs.KubernetesSecretObjectMappingResponse;
import com.pulumi.azurenative.secretsynccontroller.outputs.SecretSyncStatusResponse;
import com.pulumi.azurenative.secretsynccontroller.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The SecretSync resource.
* Azure REST API version: 2024-08-21-preview.
*
* ## Example Usage
* ### SecretSyncs_CreateOrUpdate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.secretsynccontroller.SecretSync;
* import com.pulumi.azurenative.secretsynccontroller.SecretSyncArgs;
* import com.pulumi.azurenative.secretsynccontroller.inputs.AzureResourceManagerCommonTypesExtendedLocationArgs;
* import com.pulumi.azurenative.secretsynccontroller.inputs.KubernetesSecretObjectMappingArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var secretSync = new SecretSync("secretSync", SecretSyncArgs.builder()
* .extendedLocation(AzureResourceManagerCommonTypesExtendedLocationArgs.builder()
* .name("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/rg-ssc-example/providers/Microsoft.ExtendedLocation/customLocations/example-custom-location")
* .type("CustomLocation")
* .build())
* .kubernetesSecretType("Opaque")
* .location("eastus")
* .objectSecretMapping(KubernetesSecretObjectMappingArgs.builder()
* .sourcePath("kv-secret-name/0")
* .targetKey("kv-secret-name/0")
* .build())
* .resourceGroupName("rg-ssc-example")
* .secretProviderClassName("akvspc-ssc-example")
* .secretSyncName("secretsync-ssc-example")
* .serviceAccountName("example-k8s-sa-name")
* .tags(Map.of("example-tag", "example-tag-value"))
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:secretsynccontroller:SecretSync secretsync-ssc-example /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.SecretSyncController/secretSyncs/{secretSyncName}
* ```
*
*/
@ResourceType(type="azure-native:secretsynccontroller:SecretSync")
public class SecretSync extends com.pulumi.resources.CustomResource {
/**
* The complex type of the extended location.
*
*/
@Export(name="extendedLocation", refs={AzureResourceManagerCommonTypesExtendedLocationResponse.class}, tree="[0]")
private Output* @Nullable */ AzureResourceManagerCommonTypesExtendedLocationResponse> extendedLocation;
/**
* @return The complex type of the extended location.
*
*/
public Output> extendedLocation() {
return Codegen.optional(this.extendedLocation);
}
/**
* ForceSynchronization can be used to force the secret synchronization. The secret synchronization is triggered by changing the value in this field. This field is not used to resolve synchronization conflicts.
*
*/
@Export(name="forceSynchronization", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> forceSynchronization;
/**
* @return ForceSynchronization can be used to force the secret synchronization. The secret synchronization is triggered by changing the value in this field. This field is not used to resolve synchronization conflicts.
*
*/
public Output> forceSynchronization() {
return Codegen.optional(this.forceSynchronization);
}
/**
* Type specifies the type of the Kubernetes secret object, e.g. "Opaque" or"kubernetes.io/tls". The controller must have permission to create secrets of the specified type.
*
*/
@Export(name="kubernetesSecretType", refs={String.class}, tree="[0]")
private Output kubernetesSecretType;
/**
* @return Type specifies the type of the Kubernetes secret object, e.g. "Opaque" or"kubernetes.io/tls". The controller must have permission to create secrets of the specified type.
*
*/
public Output kubernetesSecretType() {
return this.kubernetesSecretType;
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The geo-location where the resource lives
*
*/
public Output location() {
return this.location;
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* An array of SecretObjectData that maps secret data from the external secret provider to the Kubernetes secret. Each entry specifies the source secret in the external provider and the corresponding key in the Kubernetes secret.
*
*/
@Export(name="objectSecretMapping", refs={List.class,KubernetesSecretObjectMappingResponse.class}, tree="[0,1]")
private Output> objectSecretMapping;
/**
* @return An array of SecretObjectData that maps secret data from the external secret provider to the Kubernetes secret. Each entry specifies the source secret in the external provider and the corresponding key in the Kubernetes secret.
*
*/
public Output> objectSecretMapping() {
return this.objectSecretMapping;
}
/**
* Provisioning state of the SecretSync instance.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Provisioning state of the SecretSync instance.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* SecretProviderClassName specifies the name of the SecretProviderClass resource, which contains the information needed to access the cloud provider secret store.
*
*/
@Export(name="secretProviderClassName", refs={String.class}, tree="[0]")
private Output secretProviderClassName;
/**
* @return SecretProviderClassName specifies the name of the SecretProviderClass resource, which contains the information needed to access the cloud provider secret store.
*
*/
public Output secretProviderClassName() {
return this.secretProviderClassName;
}
/**
* ServiceAccountName specifies the name of the service account used to access the cloud provider secret store. The audience field in the service account token must be passed as parameter in the controller configuration. The audience is used when requesting a token from the API server for the service account; the supported audiences are defined by each provider.
*
*/
@Export(name="serviceAccountName", refs={String.class}, tree="[0]")
private Output serviceAccountName;
/**
* @return ServiceAccountName specifies the name of the service account used to access the cloud provider secret store. The audience field in the service account token must be passed as parameter in the controller configuration. The audience is used when requesting a token from the API server for the service account; the supported audiences are defined by each provider.
*
*/
public Output serviceAccountName() {
return this.serviceAccountName;
}
/**
* SecretSyncStatus defines the observed state of the secret synchronization process.
*
*/
@Export(name="status", refs={SecretSyncStatusResponse.class}, tree="[0]")
private Output status;
/**
* @return SecretSyncStatus defines the observed state of the secret synchronization process.
*
*/
public Output status() {
return this.status;
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public SecretSync(java.lang.String name) {
this(name, SecretSyncArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public SecretSync(java.lang.String name, SecretSyncArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public SecretSync(java.lang.String name, SecretSyncArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:secretsynccontroller:SecretSync", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private SecretSync(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:secretsynccontroller:SecretSync", name, null, makeResourceOptions(options, id), false);
}
private static SecretSyncArgs makeArgs(SecretSyncArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? SecretSyncArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:secretsynccontroller/v20240821preview:SecretSync").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static SecretSync get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new SecretSync(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy