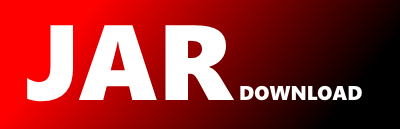
com.pulumi.azurenative.security.SecurityConnectorArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.security;
import com.pulumi.azurenative.security.enums.CloudName;
import com.pulumi.azurenative.security.inputs.AwsEnvironmentDataArgs;
import com.pulumi.azurenative.security.inputs.AzureDevOpsScopeEnvironmentDataArgs;
import com.pulumi.azurenative.security.inputs.CspmMonitorAwsOfferingArgs;
import com.pulumi.azurenative.security.inputs.CspmMonitorAzureDevOpsOfferingArgs;
import com.pulumi.azurenative.security.inputs.CspmMonitorGcpOfferingArgs;
import com.pulumi.azurenative.security.inputs.CspmMonitorGitLabOfferingArgs;
import com.pulumi.azurenative.security.inputs.CspmMonitorGithubOfferingArgs;
import com.pulumi.azurenative.security.inputs.DefenderCspmAwsOfferingArgs;
import com.pulumi.azurenative.security.inputs.DefenderCspmGcpOfferingArgs;
import com.pulumi.azurenative.security.inputs.DefenderFoDatabasesAwsOfferingArgs;
import com.pulumi.azurenative.security.inputs.DefenderForContainersAwsOfferingArgs;
import com.pulumi.azurenative.security.inputs.DefenderForContainersGcpOfferingArgs;
import com.pulumi.azurenative.security.inputs.DefenderForDatabasesGcpOfferingArgs;
import com.pulumi.azurenative.security.inputs.DefenderForDevOpsAzureDevOpsOfferingArgs;
import com.pulumi.azurenative.security.inputs.DefenderForDevOpsGitLabOfferingArgs;
import com.pulumi.azurenative.security.inputs.DefenderForDevOpsGithubOfferingArgs;
import com.pulumi.azurenative.security.inputs.DefenderForServersAwsOfferingArgs;
import com.pulumi.azurenative.security.inputs.DefenderForServersGcpOfferingArgs;
import com.pulumi.azurenative.security.inputs.GcpProjectEnvironmentDataArgs;
import com.pulumi.azurenative.security.inputs.GithubScopeEnvironmentDataArgs;
import com.pulumi.azurenative.security.inputs.GitlabScopeEnvironmentDataArgs;
import com.pulumi.azurenative.security.inputs.InformationProtectionAwsOfferingArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SecurityConnectorArgs extends com.pulumi.resources.ResourceArgs {
public static final SecurityConnectorArgs Empty = new SecurityConnectorArgs();
/**
* The security connector environment data.
*
*/
@Import(name="environmentData")
private @Nullable Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy