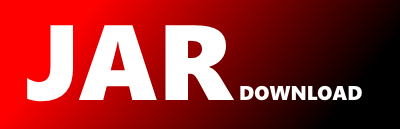
com.pulumi.azurenative.securityinsights.inputs.SapAgentConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.securityinsights.inputs;
import com.pulumi.azurenative.securityinsights.enums.KeyVaultAuthenticationMode;
import com.pulumi.azurenative.securityinsights.enums.SecretSource;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Describes the configuration of a SAP Docker agent.
*
*/
public final class SapAgentConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final SapAgentConfigurationArgs Empty = new SapAgentConfigurationArgs();
/**
* The name of the docker agent.
* only letters with numbers, underscores and hyphens are allowed
* example: "my-agent"
*
*/
@Import(name="agentContainerName")
private @Nullable Output agentContainerName;
/**
* @return The name of the docker agent.
* only letters with numbers, underscores and hyphens are allowed
* example: "my-agent"
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy