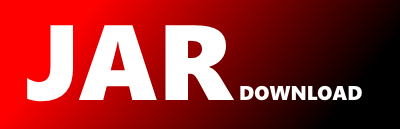
com.pulumi.azurenative.securityinsights.outputs.GetAnomalySecurityMLAnalyticsSettingsResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.securityinsights.outputs;
import com.pulumi.azurenative.securityinsights.outputs.SecurityMLAnalyticsSettingsDataSourceResponse;
import com.pulumi.azurenative.securityinsights.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetAnomalySecurityMLAnalyticsSettingsResult {
/**
* @return The anomaly settings version of the Anomaly security ml analytics settings that dictates whether job version gets updated or not.
*
*/
private @Nullable Integer anomalySettingsVersion;
/**
* @return The anomaly version of the AnomalySecurityMLAnalyticsSettings.
*
*/
private String anomalyVersion;
/**
* @return The customizable observations of the AnomalySecurityMLAnalyticsSettings.
*
*/
private @Nullable Object customizableObservations;
/**
* @return The description of the SecurityMLAnalyticsSettings.
*
*/
private @Nullable String description;
/**
* @return The display name for settings created by this SecurityMLAnalyticsSettings.
*
*/
private String displayName;
/**
* @return Determines whether this settings is enabled or disabled.
*
*/
private Boolean enabled;
/**
* @return Etag of the azure resource
*
*/
private @Nullable String etag;
/**
* @return The frequency that this SecurityMLAnalyticsSettings will be run.
*
*/
private String frequency;
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
private String id;
/**
* @return Determines whether this anomaly security ml analytics settings is a default settings
*
*/
private Boolean isDefaultSettings;
/**
* @return The kind of security ML analytics settings
* Expected value is 'Anomaly'.
*
*/
private String kind;
/**
* @return The last time that this SecurityMLAnalyticsSettings has been modified.
*
*/
private String lastModifiedUtc;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The required data sources for this SecurityMLAnalyticsSettings
*
*/
private @Nullable List requiredDataConnectors;
/**
* @return The anomaly settings definition Id
*
*/
private @Nullable String settingsDefinitionId;
/**
* @return The anomaly SecurityMLAnalyticsSettings status
*
*/
private String settingsStatus;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return The tactics of the SecurityMLAnalyticsSettings
*
*/
private @Nullable List tactics;
/**
* @return The techniques of the SecurityMLAnalyticsSettings
*
*/
private @Nullable List techniques;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetAnomalySecurityMLAnalyticsSettingsResult() {}
/**
* @return The anomaly settings version of the Anomaly security ml analytics settings that dictates whether job version gets updated or not.
*
*/
public Optional anomalySettingsVersion() {
return Optional.ofNullable(this.anomalySettingsVersion);
}
/**
* @return The anomaly version of the AnomalySecurityMLAnalyticsSettings.
*
*/
public String anomalyVersion() {
return this.anomalyVersion;
}
/**
* @return The customizable observations of the AnomalySecurityMLAnalyticsSettings.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy