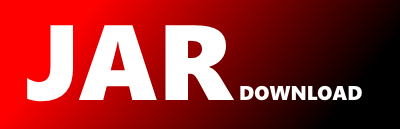
com.pulumi.azurenative.servicebus.QueueArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.servicebus;
import com.pulumi.azurenative.servicebus.enums.EntityStatus;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class QueueArgs extends com.pulumi.resources.ResourceArgs {
public static final QueueArgs Empty = new QueueArgs();
/**
* ISO 8061 timeSpan idle interval after which the queue is automatically deleted. The minimum duration is 5 minutes.
*
*/
@Import(name="autoDeleteOnIdle")
private @Nullable Output autoDeleteOnIdle;
/**
* @return ISO 8061 timeSpan idle interval after which the queue is automatically deleted. The minimum duration is 5 minutes.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy