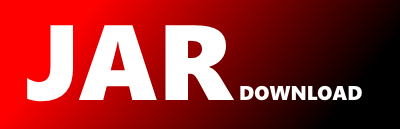
com.pulumi.azurenative.servicebus.outputs.GetTopicResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.servicebus.outputs;
import com.pulumi.azurenative.servicebus.outputs.MessageCountDetailsResponse;
import com.pulumi.azurenative.servicebus.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetTopicResult {
/**
* @return Last time the message was sent, or a request was received, for this topic.
*
*/
private String accessedAt;
/**
* @return ISO 8601 timespan idle interval after which the topic is automatically deleted. The minimum duration is 5 minutes.
*
*/
private @Nullable String autoDeleteOnIdle;
/**
* @return Message count details
*
*/
private MessageCountDetailsResponse countDetails;
/**
* @return Exact time the message was created.
*
*/
private String createdAt;
/**
* @return ISO 8601 Default message timespan to live value. This is the duration after which the message expires, starting from when the message is sent to Service Bus. This is the default value used when TimeToLive is not set on a message itself.
*
*/
private @Nullable String defaultMessageTimeToLive;
/**
* @return ISO8601 timespan structure that defines the duration of the duplicate detection history. The default value is 10 minutes.
*
*/
private @Nullable String duplicateDetectionHistoryTimeWindow;
/**
* @return Value that indicates whether server-side batched operations are enabled.
*
*/
private @Nullable Boolean enableBatchedOperations;
/**
* @return Value that indicates whether Express Entities are enabled. An express topic holds a message in memory temporarily before writing it to persistent storage.
*
*/
private @Nullable Boolean enableExpress;
/**
* @return Value that indicates whether the topic to be partitioned across multiple message brokers is enabled.
*
*/
private @Nullable Boolean enablePartitioning;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return Maximum size (in KB) of the message payload that can be accepted by the topic. This property is only used in Premium today and default is 1024.
*
*/
private @Nullable Double maxMessageSizeInKilobytes;
/**
* @return Maximum size of the topic in megabytes, which is the size of the memory allocated for the topic. Default is 1024.
*
*/
private @Nullable Integer maxSizeInMegabytes;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Value indicating if this topic requires duplicate detection.
*
*/
private @Nullable Boolean requiresDuplicateDetection;
/**
* @return Size of the topic, in bytes.
*
*/
private Double sizeInBytes;
/**
* @return Enumerates the possible values for the status of a messaging entity.
*
*/
private @Nullable String status;
/**
* @return Number of subscriptions.
*
*/
private Integer subscriptionCount;
/**
* @return Value that indicates whether the topic supports ordering.
*
*/
private @Nullable Boolean supportOrdering;
/**
* @return The system meta data relating to this resource.
*
*/
private SystemDataResponse systemData;
/**
* @return The type of the resource. E.g. "Microsoft.EventHub/Namespaces" or "Microsoft.EventHub/Namespaces/EventHubs"
*
*/
private String type;
/**
* @return The exact time the message was updated.
*
*/
private String updatedAt;
private GetTopicResult() {}
/**
* @return Last time the message was sent, or a request was received, for this topic.
*
*/
public String accessedAt() {
return this.accessedAt;
}
/**
* @return ISO 8601 timespan idle interval after which the topic is automatically deleted. The minimum duration is 5 minutes.
*
*/
public Optional autoDeleteOnIdle() {
return Optional.ofNullable(this.autoDeleteOnIdle);
}
/**
* @return Message count details
*
*/
public MessageCountDetailsResponse countDetails() {
return this.countDetails;
}
/**
* @return Exact time the message was created.
*
*/
public String createdAt() {
return this.createdAt;
}
/**
* @return ISO 8601 Default message timespan to live value. This is the duration after which the message expires, starting from when the message is sent to Service Bus. This is the default value used when TimeToLive is not set on a message itself.
*
*/
public Optional defaultMessageTimeToLive() {
return Optional.ofNullable(this.defaultMessageTimeToLive);
}
/**
* @return ISO8601 timespan structure that defines the duration of the duplicate detection history. The default value is 10 minutes.
*
*/
public Optional duplicateDetectionHistoryTimeWindow() {
return Optional.ofNullable(this.duplicateDetectionHistoryTimeWindow);
}
/**
* @return Value that indicates whether server-side batched operations are enabled.
*
*/
public Optional enableBatchedOperations() {
return Optional.ofNullable(this.enableBatchedOperations);
}
/**
* @return Value that indicates whether Express Entities are enabled. An express topic holds a message in memory temporarily before writing it to persistent storage.
*
*/
public Optional enableExpress() {
return Optional.ofNullable(this.enableExpress);
}
/**
* @return Value that indicates whether the topic to be partitioned across multiple message brokers is enabled.
*
*/
public Optional enablePartitioning() {
return Optional.ofNullable(this.enablePartitioning);
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return Maximum size (in KB) of the message payload that can be accepted by the topic. This property is only used in Premium today and default is 1024.
*
*/
public Optional maxMessageSizeInKilobytes() {
return Optional.ofNullable(this.maxMessageSizeInKilobytes);
}
/**
* @return Maximum size of the topic in megabytes, which is the size of the memory allocated for the topic. Default is 1024.
*
*/
public Optional maxSizeInMegabytes() {
return Optional.ofNullable(this.maxSizeInMegabytes);
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Value indicating if this topic requires duplicate detection.
*
*/
public Optional requiresDuplicateDetection() {
return Optional.ofNullable(this.requiresDuplicateDetection);
}
/**
* @return Size of the topic, in bytes.
*
*/
public Double sizeInBytes() {
return this.sizeInBytes;
}
/**
* @return Enumerates the possible values for the status of a messaging entity.
*
*/
public Optional status() {
return Optional.ofNullable(this.status);
}
/**
* @return Number of subscriptions.
*
*/
public Integer subscriptionCount() {
return this.subscriptionCount;
}
/**
* @return Value that indicates whether the topic supports ordering.
*
*/
public Optional supportOrdering() {
return Optional.ofNullable(this.supportOrdering);
}
/**
* @return The system meta data relating to this resource.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The type of the resource. E.g. "Microsoft.EventHub/Namespaces" or "Microsoft.EventHub/Namespaces/EventHubs"
*
*/
public String type() {
return this.type;
}
/**
* @return The exact time the message was updated.
*
*/
public String updatedAt() {
return this.updatedAt;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetTopicResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String accessedAt;
private @Nullable String autoDeleteOnIdle;
private MessageCountDetailsResponse countDetails;
private String createdAt;
private @Nullable String defaultMessageTimeToLive;
private @Nullable String duplicateDetectionHistoryTimeWindow;
private @Nullable Boolean enableBatchedOperations;
private @Nullable Boolean enableExpress;
private @Nullable Boolean enablePartitioning;
private String id;
private String location;
private @Nullable Double maxMessageSizeInKilobytes;
private @Nullable Integer maxSizeInMegabytes;
private String name;
private @Nullable Boolean requiresDuplicateDetection;
private Double sizeInBytes;
private @Nullable String status;
private Integer subscriptionCount;
private @Nullable Boolean supportOrdering;
private SystemDataResponse systemData;
private String type;
private String updatedAt;
public Builder() {}
public Builder(GetTopicResult defaults) {
Objects.requireNonNull(defaults);
this.accessedAt = defaults.accessedAt;
this.autoDeleteOnIdle = defaults.autoDeleteOnIdle;
this.countDetails = defaults.countDetails;
this.createdAt = defaults.createdAt;
this.defaultMessageTimeToLive = defaults.defaultMessageTimeToLive;
this.duplicateDetectionHistoryTimeWindow = defaults.duplicateDetectionHistoryTimeWindow;
this.enableBatchedOperations = defaults.enableBatchedOperations;
this.enableExpress = defaults.enableExpress;
this.enablePartitioning = defaults.enablePartitioning;
this.id = defaults.id;
this.location = defaults.location;
this.maxMessageSizeInKilobytes = defaults.maxMessageSizeInKilobytes;
this.maxSizeInMegabytes = defaults.maxSizeInMegabytes;
this.name = defaults.name;
this.requiresDuplicateDetection = defaults.requiresDuplicateDetection;
this.sizeInBytes = defaults.sizeInBytes;
this.status = defaults.status;
this.subscriptionCount = defaults.subscriptionCount;
this.supportOrdering = defaults.supportOrdering;
this.systemData = defaults.systemData;
this.type = defaults.type;
this.updatedAt = defaults.updatedAt;
}
@CustomType.Setter
public Builder accessedAt(String accessedAt) {
if (accessedAt == null) {
throw new MissingRequiredPropertyException("GetTopicResult", "accessedAt");
}
this.accessedAt = accessedAt;
return this;
}
@CustomType.Setter
public Builder autoDeleteOnIdle(@Nullable String autoDeleteOnIdle) {
this.autoDeleteOnIdle = autoDeleteOnIdle;
return this;
}
@CustomType.Setter
public Builder countDetails(MessageCountDetailsResponse countDetails) {
if (countDetails == null) {
throw new MissingRequiredPropertyException("GetTopicResult", "countDetails");
}
this.countDetails = countDetails;
return this;
}
@CustomType.Setter
public Builder createdAt(String createdAt) {
if (createdAt == null) {
throw new MissingRequiredPropertyException("GetTopicResult", "createdAt");
}
this.createdAt = createdAt;
return this;
}
@CustomType.Setter
public Builder defaultMessageTimeToLive(@Nullable String defaultMessageTimeToLive) {
this.defaultMessageTimeToLive = defaultMessageTimeToLive;
return this;
}
@CustomType.Setter
public Builder duplicateDetectionHistoryTimeWindow(@Nullable String duplicateDetectionHistoryTimeWindow) {
this.duplicateDetectionHistoryTimeWindow = duplicateDetectionHistoryTimeWindow;
return this;
}
@CustomType.Setter
public Builder enableBatchedOperations(@Nullable Boolean enableBatchedOperations) {
this.enableBatchedOperations = enableBatchedOperations;
return this;
}
@CustomType.Setter
public Builder enableExpress(@Nullable Boolean enableExpress) {
this.enableExpress = enableExpress;
return this;
}
@CustomType.Setter
public Builder enablePartitioning(@Nullable Boolean enablePartitioning) {
this.enablePartitioning = enablePartitioning;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetTopicResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetTopicResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder maxMessageSizeInKilobytes(@Nullable Double maxMessageSizeInKilobytes) {
this.maxMessageSizeInKilobytes = maxMessageSizeInKilobytes;
return this;
}
@CustomType.Setter
public Builder maxSizeInMegabytes(@Nullable Integer maxSizeInMegabytes) {
this.maxSizeInMegabytes = maxSizeInMegabytes;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetTopicResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder requiresDuplicateDetection(@Nullable Boolean requiresDuplicateDetection) {
this.requiresDuplicateDetection = requiresDuplicateDetection;
return this;
}
@CustomType.Setter
public Builder sizeInBytes(Double sizeInBytes) {
if (sizeInBytes == null) {
throw new MissingRequiredPropertyException("GetTopicResult", "sizeInBytes");
}
this.sizeInBytes = sizeInBytes;
return this;
}
@CustomType.Setter
public Builder status(@Nullable String status) {
this.status = status;
return this;
}
@CustomType.Setter
public Builder subscriptionCount(Integer subscriptionCount) {
if (subscriptionCount == null) {
throw new MissingRequiredPropertyException("GetTopicResult", "subscriptionCount");
}
this.subscriptionCount = subscriptionCount;
return this;
}
@CustomType.Setter
public Builder supportOrdering(@Nullable Boolean supportOrdering) {
this.supportOrdering = supportOrdering;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetTopicResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetTopicResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder updatedAt(String updatedAt) {
if (updatedAt == null) {
throw new MissingRequiredPropertyException("GetTopicResult", "updatedAt");
}
this.updatedAt = updatedAt;
return this;
}
public GetTopicResult build() {
final var _resultValue = new GetTopicResult();
_resultValue.accessedAt = accessedAt;
_resultValue.autoDeleteOnIdle = autoDeleteOnIdle;
_resultValue.countDetails = countDetails;
_resultValue.createdAt = createdAt;
_resultValue.defaultMessageTimeToLive = defaultMessageTimeToLive;
_resultValue.duplicateDetectionHistoryTimeWindow = duplicateDetectionHistoryTimeWindow;
_resultValue.enableBatchedOperations = enableBatchedOperations;
_resultValue.enableExpress = enableExpress;
_resultValue.enablePartitioning = enablePartitioning;
_resultValue.id = id;
_resultValue.location = location;
_resultValue.maxMessageSizeInKilobytes = maxMessageSizeInKilobytes;
_resultValue.maxSizeInMegabytes = maxSizeInMegabytes;
_resultValue.name = name;
_resultValue.requiresDuplicateDetection = requiresDuplicateDetection;
_resultValue.sizeInBytes = sizeInBytes;
_resultValue.status = status;
_resultValue.subscriptionCount = subscriptionCount;
_resultValue.supportOrdering = supportOrdering;
_resultValue.systemData = systemData;
_resultValue.type = type;
_resultValue.updatedAt = updatedAt;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy