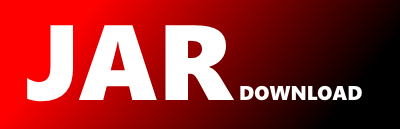
com.pulumi.azurenative.servicefabric.ManagedClusterArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.servicefabric;
import com.pulumi.azurenative.servicefabric.enums.ClusterUpgradeCadence;
import com.pulumi.azurenative.servicefabric.enums.ClusterUpgradeMode;
import com.pulumi.azurenative.servicefabric.enums.ManagedClusterAddOnFeature;
import com.pulumi.azurenative.servicefabric.enums.ZonalUpdateMode;
import com.pulumi.azurenative.servicefabric.inputs.ApplicationTypeVersionsCleanupPolicyArgs;
import com.pulumi.azurenative.servicefabric.inputs.AzureActiveDirectoryArgs;
import com.pulumi.azurenative.servicefabric.inputs.ClientCertificateArgs;
import com.pulumi.azurenative.servicefabric.inputs.IPTagArgs;
import com.pulumi.azurenative.servicefabric.inputs.LoadBalancingRuleArgs;
import com.pulumi.azurenative.servicefabric.inputs.NetworkSecurityRuleArgs;
import com.pulumi.azurenative.servicefabric.inputs.ServiceEndpointArgs;
import com.pulumi.azurenative.servicefabric.inputs.SettingsSectionDescriptionArgs;
import com.pulumi.azurenative.servicefabric.inputs.SkuArgs;
import com.pulumi.azurenative.servicefabric.inputs.SubnetArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ManagedClusterArgs extends com.pulumi.resources.ResourceArgs {
public static final ManagedClusterArgs Empty = new ManagedClusterArgs();
/**
* List of add-on features to enable on the cluster.
*
*/
@Import(name="addonFeatures")
private @Nullable Output>> addonFeatures;
/**
* @return List of add-on features to enable on the cluster.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy