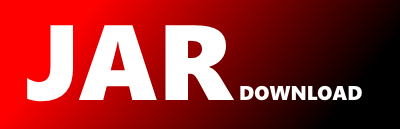
com.pulumi.azurenative.servicefabric.outputs.RollingUpgradeMonitoringPolicyResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.servicefabric.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class RollingUpgradeMonitoringPolicyResponse {
/**
* @return The compensating action to perform when a Monitored upgrade encounters monitoring policy or health policy violations. Invalid indicates the failure action is invalid. Rollback specifies that the upgrade will start rolling back automatically. Manual indicates that the upgrade will switch to UnmonitoredManual upgrade mode.
*
*/
private String failureAction;
/**
* @return The amount of time to retry health evaluation when the application or cluster is unhealthy before FailureAction is executed. It is interpreted as a string representing an ISO 8601 duration with following format "hh:mm:ss.fff".
*
*/
private String healthCheckRetryTimeout;
/**
* @return The amount of time that the application or cluster must remain healthy before the upgrade proceeds to the next upgrade domain. It is interpreted as a string representing an ISO 8601 duration with following format "hh:mm:ss.fff".
*
*/
private String healthCheckStableDuration;
/**
* @return The amount of time to wait after completing an upgrade domain before applying health policies. It is interpreted as a string representing an ISO 8601 duration with following format "hh:mm:ss.fff".
*
*/
private String healthCheckWaitDuration;
/**
* @return The amount of time each upgrade domain has to complete before FailureAction is executed. Cannot be larger than 12 hours. It is interpreted as a string representing an ISO 8601 duration with following format "hh:mm:ss.fff".
*
*/
private String upgradeDomainTimeout;
/**
* @return The amount of time the overall upgrade has to complete before FailureAction is executed. Cannot be larger than 12 hours. It is interpreted as a string representing an ISO 8601 duration with following format "hh:mm:ss.fff".
*
*/
private String upgradeTimeout;
private RollingUpgradeMonitoringPolicyResponse() {}
/**
* @return The compensating action to perform when a Monitored upgrade encounters monitoring policy or health policy violations. Invalid indicates the failure action is invalid. Rollback specifies that the upgrade will start rolling back automatically. Manual indicates that the upgrade will switch to UnmonitoredManual upgrade mode.
*
*/
public String failureAction() {
return this.failureAction;
}
/**
* @return The amount of time to retry health evaluation when the application or cluster is unhealthy before FailureAction is executed. It is interpreted as a string representing an ISO 8601 duration with following format "hh:mm:ss.fff".
*
*/
public String healthCheckRetryTimeout() {
return this.healthCheckRetryTimeout;
}
/**
* @return The amount of time that the application or cluster must remain healthy before the upgrade proceeds to the next upgrade domain. It is interpreted as a string representing an ISO 8601 duration with following format "hh:mm:ss.fff".
*
*/
public String healthCheckStableDuration() {
return this.healthCheckStableDuration;
}
/**
* @return The amount of time to wait after completing an upgrade domain before applying health policies. It is interpreted as a string representing an ISO 8601 duration with following format "hh:mm:ss.fff".
*
*/
public String healthCheckWaitDuration() {
return this.healthCheckWaitDuration;
}
/**
* @return The amount of time each upgrade domain has to complete before FailureAction is executed. Cannot be larger than 12 hours. It is interpreted as a string representing an ISO 8601 duration with following format "hh:mm:ss.fff".
*
*/
public String upgradeDomainTimeout() {
return this.upgradeDomainTimeout;
}
/**
* @return The amount of time the overall upgrade has to complete before FailureAction is executed. Cannot be larger than 12 hours. It is interpreted as a string representing an ISO 8601 duration with following format "hh:mm:ss.fff".
*
*/
public String upgradeTimeout() {
return this.upgradeTimeout;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(RollingUpgradeMonitoringPolicyResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String failureAction;
private String healthCheckRetryTimeout;
private String healthCheckStableDuration;
private String healthCheckWaitDuration;
private String upgradeDomainTimeout;
private String upgradeTimeout;
public Builder() {}
public Builder(RollingUpgradeMonitoringPolicyResponse defaults) {
Objects.requireNonNull(defaults);
this.failureAction = defaults.failureAction;
this.healthCheckRetryTimeout = defaults.healthCheckRetryTimeout;
this.healthCheckStableDuration = defaults.healthCheckStableDuration;
this.healthCheckWaitDuration = defaults.healthCheckWaitDuration;
this.upgradeDomainTimeout = defaults.upgradeDomainTimeout;
this.upgradeTimeout = defaults.upgradeTimeout;
}
@CustomType.Setter
public Builder failureAction(String failureAction) {
if (failureAction == null) {
throw new MissingRequiredPropertyException("RollingUpgradeMonitoringPolicyResponse", "failureAction");
}
this.failureAction = failureAction;
return this;
}
@CustomType.Setter
public Builder healthCheckRetryTimeout(String healthCheckRetryTimeout) {
if (healthCheckRetryTimeout == null) {
throw new MissingRequiredPropertyException("RollingUpgradeMonitoringPolicyResponse", "healthCheckRetryTimeout");
}
this.healthCheckRetryTimeout = healthCheckRetryTimeout;
return this;
}
@CustomType.Setter
public Builder healthCheckStableDuration(String healthCheckStableDuration) {
if (healthCheckStableDuration == null) {
throw new MissingRequiredPropertyException("RollingUpgradeMonitoringPolicyResponse", "healthCheckStableDuration");
}
this.healthCheckStableDuration = healthCheckStableDuration;
return this;
}
@CustomType.Setter
public Builder healthCheckWaitDuration(String healthCheckWaitDuration) {
if (healthCheckWaitDuration == null) {
throw new MissingRequiredPropertyException("RollingUpgradeMonitoringPolicyResponse", "healthCheckWaitDuration");
}
this.healthCheckWaitDuration = healthCheckWaitDuration;
return this;
}
@CustomType.Setter
public Builder upgradeDomainTimeout(String upgradeDomainTimeout) {
if (upgradeDomainTimeout == null) {
throw new MissingRequiredPropertyException("RollingUpgradeMonitoringPolicyResponse", "upgradeDomainTimeout");
}
this.upgradeDomainTimeout = upgradeDomainTimeout;
return this;
}
@CustomType.Setter
public Builder upgradeTimeout(String upgradeTimeout) {
if (upgradeTimeout == null) {
throw new MissingRequiredPropertyException("RollingUpgradeMonitoringPolicyResponse", "upgradeTimeout");
}
this.upgradeTimeout = upgradeTimeout;
return this;
}
public RollingUpgradeMonitoringPolicyResponse build() {
final var _resultValue = new RollingUpgradeMonitoringPolicyResponse();
_resultValue.failureAction = failureAction;
_resultValue.healthCheckRetryTimeout = healthCheckRetryTimeout;
_resultValue.healthCheckStableDuration = healthCheckStableDuration;
_resultValue.healthCheckWaitDuration = healthCheckWaitDuration;
_resultValue.upgradeDomainTimeout = upgradeDomainTimeout;
_resultValue.upgradeTimeout = upgradeTimeout;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy