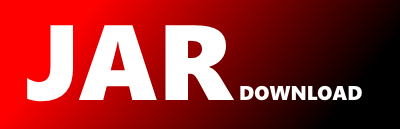
com.pulumi.azurenative.signalrservice.inputs.SignalRFeatureArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.signalrservice.inputs;
import com.pulumi.azurenative.signalrservice.enums.FeatureFlags;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Feature of a resource, which controls the runtime behavior.
*
*/
public final class SignalRFeatureArgs extends com.pulumi.resources.ResourceArgs {
public static final SignalRFeatureArgs Empty = new SignalRFeatureArgs();
/**
* FeatureFlags is the supported features of Azure SignalR service.
* - ServiceMode: Flag for backend server for SignalR service. Values allowed: "Default": have your own backend server; "Serverless": your application doesn't have a backend server; "Classic": for backward compatibility. Support both Default and Serverless mode but not recommended; "PredefinedOnly": for future use.
* - EnableConnectivityLogs: "true"/"false", to enable/disable the connectivity log category respectively.
* - EnableMessagingLogs: "true"/"false", to enable/disable the connectivity log category respectively.
* - EnableLiveTrace: Live Trace allows you to know what's happening inside Azure SignalR service, it will give you live traces in real time, it will be helpful when you developing your own Azure SignalR based web application or self-troubleshooting some issues. Please note that live traces are counted as outbound messages that will be charged. Values allowed: "true"/"false", to enable/disable live trace feature.
*
*/
@Import(name="flag", required=true)
private Output> flag;
/**
* @return FeatureFlags is the supported features of Azure SignalR service.
* - ServiceMode: Flag for backend server for SignalR service. Values allowed: "Default": have your own backend server; "Serverless": your application doesn't have a backend server; "Classic": for backward compatibility. Support both Default and Serverless mode but not recommended; "PredefinedOnly": for future use.
* - EnableConnectivityLogs: "true"/"false", to enable/disable the connectivity log category respectively.
* - EnableMessagingLogs: "true"/"false", to enable/disable the connectivity log category respectively.
* - EnableLiveTrace: Live Trace allows you to know what's happening inside Azure SignalR service, it will give you live traces in real time, it will be helpful when you developing your own Azure SignalR based web application or self-troubleshooting some issues. Please note that live traces are counted as outbound messages that will be charged. Values allowed: "true"/"false", to enable/disable live trace feature.
*
*/
public Output> flag() {
return this.flag;
}
/**
* Optional properties related to this feature.
*
*/
@Import(name="properties")
private @Nullable Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy