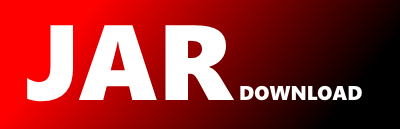
com.pulumi.azurenative.signalrservice.outputs.UpstreamTemplateResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.signalrservice.outputs;
import com.pulumi.azurenative.signalrservice.outputs.UpstreamAuthSettingsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class UpstreamTemplateResponse {
/**
* @return Upstream auth settings. If not set, no auth is used for upstream messages.
*
*/
private @Nullable UpstreamAuthSettingsResponse auth;
/**
* @return Gets or sets the matching pattern for category names. If not set, it matches any category.
* There are 3 kind of patterns supported:
* 1. "*", it to matches any category name.
* 2. Combine multiple categories with ",", for example "connections,messages", it matches category "connections" and "messages".
* 3. The single category name, for example, "connections", it matches the category "connections".
*
*/
private @Nullable String categoryPattern;
/**
* @return Gets or sets the matching pattern for event names. If not set, it matches any event.
* There are 3 kind of patterns supported:
* 1. "*", it to matches any event name.
* 2. Combine multiple events with ",", for example "connect,disconnect", it matches event "connect" and "disconnect".
* 3. The single event name, for example, "connect", it matches "connect".
*
*/
private @Nullable String eventPattern;
/**
* @return Gets or sets the matching pattern for hub names. If not set, it matches any hub.
* There are 3 kind of patterns supported:
* 1. "*", it to matches any hub name.
* 2. Combine multiple hubs with ",", for example "hub1,hub2", it matches "hub1" and "hub2".
* 3. The single hub name, for example, "hub1", it matches "hub1".
*
*/
private @Nullable String hubPattern;
/**
* @return Gets or sets the Upstream URL template. You can use 3 predefined parameters {hub}, {category} {event} inside the template, the value of the Upstream URL is dynamically calculated when the client request comes in.
* For example, if the urlTemplate is `http://example.com/{hub}/api/{event}`, with a client request from hub `chat` connects, it will first POST to this URL: `http://example.com/chat/api/connect`.
*
*/
private String urlTemplate;
private UpstreamTemplateResponse() {}
/**
* @return Upstream auth settings. If not set, no auth is used for upstream messages.
*
*/
public Optional auth() {
return Optional.ofNullable(this.auth);
}
/**
* @return Gets or sets the matching pattern for category names. If not set, it matches any category.
* There are 3 kind of patterns supported:
* 1. "*", it to matches any category name.
* 2. Combine multiple categories with ",", for example "connections,messages", it matches category "connections" and "messages".
* 3. The single category name, for example, "connections", it matches the category "connections".
*
*/
public Optional categoryPattern() {
return Optional.ofNullable(this.categoryPattern);
}
/**
* @return Gets or sets the matching pattern for event names. If not set, it matches any event.
* There are 3 kind of patterns supported:
* 1. "*", it to matches any event name.
* 2. Combine multiple events with ",", for example "connect,disconnect", it matches event "connect" and "disconnect".
* 3. The single event name, for example, "connect", it matches "connect".
*
*/
public Optional eventPattern() {
return Optional.ofNullable(this.eventPattern);
}
/**
* @return Gets or sets the matching pattern for hub names. If not set, it matches any hub.
* There are 3 kind of patterns supported:
* 1. "*", it to matches any hub name.
* 2. Combine multiple hubs with ",", for example "hub1,hub2", it matches "hub1" and "hub2".
* 3. The single hub name, for example, "hub1", it matches "hub1".
*
*/
public Optional hubPattern() {
return Optional.ofNullable(this.hubPattern);
}
/**
* @return Gets or sets the Upstream URL template. You can use 3 predefined parameters {hub}, {category} {event} inside the template, the value of the Upstream URL is dynamically calculated when the client request comes in.
* For example, if the urlTemplate is `http://example.com/{hub}/api/{event}`, with a client request from hub `chat` connects, it will first POST to this URL: `http://example.com/chat/api/connect`.
*
*/
public String urlTemplate() {
return this.urlTemplate;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(UpstreamTemplateResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable UpstreamAuthSettingsResponse auth;
private @Nullable String categoryPattern;
private @Nullable String eventPattern;
private @Nullable String hubPattern;
private String urlTemplate;
public Builder() {}
public Builder(UpstreamTemplateResponse defaults) {
Objects.requireNonNull(defaults);
this.auth = defaults.auth;
this.categoryPattern = defaults.categoryPattern;
this.eventPattern = defaults.eventPattern;
this.hubPattern = defaults.hubPattern;
this.urlTemplate = defaults.urlTemplate;
}
@CustomType.Setter
public Builder auth(@Nullable UpstreamAuthSettingsResponse auth) {
this.auth = auth;
return this;
}
@CustomType.Setter
public Builder categoryPattern(@Nullable String categoryPattern) {
this.categoryPattern = categoryPattern;
return this;
}
@CustomType.Setter
public Builder eventPattern(@Nullable String eventPattern) {
this.eventPattern = eventPattern;
return this;
}
@CustomType.Setter
public Builder hubPattern(@Nullable String hubPattern) {
this.hubPattern = hubPattern;
return this;
}
@CustomType.Setter
public Builder urlTemplate(String urlTemplate) {
if (urlTemplate == null) {
throw new MissingRequiredPropertyException("UpstreamTemplateResponse", "urlTemplate");
}
this.urlTemplate = urlTemplate;
return this;
}
public UpstreamTemplateResponse build() {
final var _resultValue = new UpstreamTemplateResponse();
_resultValue.auth = auth;
_resultValue.categoryPattern = categoryPattern;
_resultValue.eventPattern = eventPattern;
_resultValue.hubPattern = hubPattern;
_resultValue.urlTemplate = urlTemplate;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy