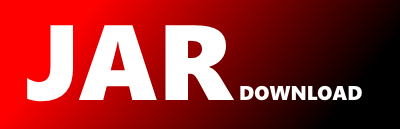
com.pulumi.azurenative.solutions.ApplicationDefinitionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.solutions;
import com.pulumi.azurenative.solutions.enums.ApplicationLockLevel;
import com.pulumi.azurenative.solutions.inputs.ApplicationAuthorizationArgs;
import com.pulumi.azurenative.solutions.inputs.ApplicationDefinitionArtifactArgs;
import com.pulumi.azurenative.solutions.inputs.ApplicationDeploymentPolicyArgs;
import com.pulumi.azurenative.solutions.inputs.ApplicationManagementPolicyArgs;
import com.pulumi.azurenative.solutions.inputs.ApplicationNotificationPolicyArgs;
import com.pulumi.azurenative.solutions.inputs.ApplicationPackageLockingPolicyDefinitionArgs;
import com.pulumi.azurenative.solutions.inputs.ApplicationPolicyArgs;
import com.pulumi.azurenative.solutions.inputs.SkuArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ApplicationDefinitionArgs extends com.pulumi.resources.ResourceArgs {
public static final ApplicationDefinitionArgs Empty = new ApplicationDefinitionArgs();
/**
* The name of the managed application definition.
*
*/
@Import(name="applicationDefinitionName")
private @Nullable Output applicationDefinitionName;
/**
* @return The name of the managed application definition.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy