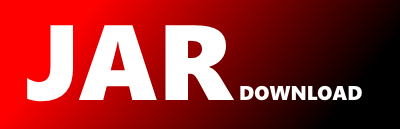
com.pulumi.azurenative.sql.SyncMemberArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.sql;
import com.pulumi.azurenative.sql.enums.SyncDirection;
import com.pulumi.azurenative.sql.enums.SyncMemberDbType;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SyncMemberArgs extends com.pulumi.resources.ResourceArgs {
public static final SyncMemberArgs Empty = new SyncMemberArgs();
/**
* Database name of the member database in the sync member.
*
*/
@Import(name="databaseName", required=true)
private Output databaseName;
/**
* @return Database name of the member database in the sync member.
*
*/
public Output databaseName() {
return this.databaseName;
}
/**
* Database type of the sync member.
*
*/
@Import(name="databaseType")
private @Nullable Output> databaseType;
/**
* @return Database type of the sync member.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy