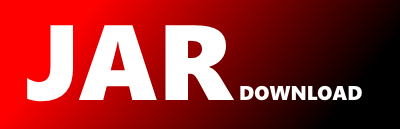
com.pulumi.azurenative.sql.outputs.GetFailoverGroupResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.sql.outputs;
import com.pulumi.azurenative.sql.outputs.FailoverGroupReadOnlyEndpointResponse;
import com.pulumi.azurenative.sql.outputs.FailoverGroupReadWriteEndpointResponse;
import com.pulumi.azurenative.sql.outputs.PartnerInfoResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetFailoverGroupResult {
/**
* @return List of databases in the failover group.
*
*/
private @Nullable List databases;
/**
* @return Resource ID.
*
*/
private String id;
/**
* @return Resource location.
*
*/
private String location;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return List of partner server information for the failover group.
*
*/
private List partnerServers;
/**
* @return Read-only endpoint of the failover group instance.
*
*/
private @Nullable FailoverGroupReadOnlyEndpointResponse readOnlyEndpoint;
/**
* @return Read-write endpoint of the failover group instance.
*
*/
private FailoverGroupReadWriteEndpointResponse readWriteEndpoint;
/**
* @return Local replication role of the failover group instance.
*
*/
private String replicationRole;
/**
* @return Replication state of the failover group instance.
*
*/
private String replicationState;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Resource type.
*
*/
private String type;
private GetFailoverGroupResult() {}
/**
* @return List of databases in the failover group.
*
*/
public List databases() {
return this.databases == null ? List.of() : this.databases;
}
/**
* @return Resource ID.
*
*/
public String id() {
return this.id;
}
/**
* @return Resource location.
*
*/
public String location() {
return this.location;
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return List of partner server information for the failover group.
*
*/
public List partnerServers() {
return this.partnerServers;
}
/**
* @return Read-only endpoint of the failover group instance.
*
*/
public Optional readOnlyEndpoint() {
return Optional.ofNullable(this.readOnlyEndpoint);
}
/**
* @return Read-write endpoint of the failover group instance.
*
*/
public FailoverGroupReadWriteEndpointResponse readWriteEndpoint() {
return this.readWriteEndpoint;
}
/**
* @return Local replication role of the failover group instance.
*
*/
public String replicationRole() {
return this.replicationRole;
}
/**
* @return Replication state of the failover group instance.
*
*/
public String replicationState() {
return this.replicationState;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetFailoverGroupResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List databases;
private String id;
private String location;
private String name;
private List partnerServers;
private @Nullable FailoverGroupReadOnlyEndpointResponse readOnlyEndpoint;
private FailoverGroupReadWriteEndpointResponse readWriteEndpoint;
private String replicationRole;
private String replicationState;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetFailoverGroupResult defaults) {
Objects.requireNonNull(defaults);
this.databases = defaults.databases;
this.id = defaults.id;
this.location = defaults.location;
this.name = defaults.name;
this.partnerServers = defaults.partnerServers;
this.readOnlyEndpoint = defaults.readOnlyEndpoint;
this.readWriteEndpoint = defaults.readWriteEndpoint;
this.replicationRole = defaults.replicationRole;
this.replicationState = defaults.replicationState;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder databases(@Nullable List databases) {
this.databases = databases;
return this;
}
public Builder databases(String... databases) {
return databases(List.of(databases));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetFailoverGroupResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetFailoverGroupResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetFailoverGroupResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder partnerServers(List partnerServers) {
if (partnerServers == null) {
throw new MissingRequiredPropertyException("GetFailoverGroupResult", "partnerServers");
}
this.partnerServers = partnerServers;
return this;
}
public Builder partnerServers(PartnerInfoResponse... partnerServers) {
return partnerServers(List.of(partnerServers));
}
@CustomType.Setter
public Builder readOnlyEndpoint(@Nullable FailoverGroupReadOnlyEndpointResponse readOnlyEndpoint) {
this.readOnlyEndpoint = readOnlyEndpoint;
return this;
}
@CustomType.Setter
public Builder readWriteEndpoint(FailoverGroupReadWriteEndpointResponse readWriteEndpoint) {
if (readWriteEndpoint == null) {
throw new MissingRequiredPropertyException("GetFailoverGroupResult", "readWriteEndpoint");
}
this.readWriteEndpoint = readWriteEndpoint;
return this;
}
@CustomType.Setter
public Builder replicationRole(String replicationRole) {
if (replicationRole == null) {
throw new MissingRequiredPropertyException("GetFailoverGroupResult", "replicationRole");
}
this.replicationRole = replicationRole;
return this;
}
@CustomType.Setter
public Builder replicationState(String replicationState) {
if (replicationState == null) {
throw new MissingRequiredPropertyException("GetFailoverGroupResult", "replicationState");
}
this.replicationState = replicationState;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetFailoverGroupResult", "type");
}
this.type = type;
return this;
}
public GetFailoverGroupResult build() {
final var _resultValue = new GetFailoverGroupResult();
_resultValue.databases = databases;
_resultValue.id = id;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.partnerServers = partnerServers;
_resultValue.readOnlyEndpoint = readOnlyEndpoint;
_resultValue.readWriteEndpoint = readWriteEndpoint;
_resultValue.replicationRole = replicationRole;
_resultValue.replicationState = replicationState;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy