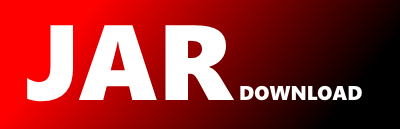
com.pulumi.azurenative.storage.BlobContainer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storage;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.storage.BlobContainerArgs;
import com.pulumi.azurenative.storage.outputs.ImmutabilityPolicyPropertiesResponse;
import com.pulumi.azurenative.storage.outputs.ImmutableStorageWithVersioningResponse;
import com.pulumi.azurenative.storage.outputs.LegalHoldPropertiesResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Properties of the blob container, including Id, resource name, resource type, Etag.
* Azure REST API version: 2022-09-01. Prior API version in Azure Native 1.x: 2021-02-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
* ## Example Usage
* ### PutContainerWithDefaultEncryptionScope
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.BlobContainer;
* import com.pulumi.azurenative.storage.BlobContainerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var blobContainer = new BlobContainer("blobContainer", BlobContainerArgs.builder()
* .accountName("sto328")
* .containerName("container6185")
* .defaultEncryptionScope("encryptionscope185")
* .denyEncryptionScopeOverride(true)
* .resourceGroupName("res3376")
* .build());
*
* }
* }
*
* }
*
* ### PutContainerWithObjectLevelWorm
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.BlobContainer;
* import com.pulumi.azurenative.storage.BlobContainerArgs;
* import com.pulumi.azurenative.storage.inputs.ImmutableStorageWithVersioningArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var blobContainer = new BlobContainer("blobContainer", BlobContainerArgs.builder()
* .accountName("sto328")
* .containerName("container6185")
* .immutableStorageWithVersioning(ImmutableStorageWithVersioningArgs.builder()
* .enabled(true)
* .build())
* .resourceGroupName("res3376")
* .build());
*
* }
* }
*
* }
*
* ### PutContainers
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.BlobContainer;
* import com.pulumi.azurenative.storage.BlobContainerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var blobContainer = new BlobContainer("blobContainer", BlobContainerArgs.builder()
* .accountName("sto328")
* .containerName("container6185")
* .resourceGroupName("res3376")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:storage:BlobContainer container6185 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Storage/storageAccounts/{accountName}/blobServices/default/containers/{containerName}
* ```
*
*/
@ResourceType(type="azure-native:storage:BlobContainer")
public class BlobContainer extends com.pulumi.resources.CustomResource {
/**
* Default the container to use specified encryption scope for all writes.
*
*/
@Export(name="defaultEncryptionScope", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> defaultEncryptionScope;
/**
* @return Default the container to use specified encryption scope for all writes.
*
*/
public Output> defaultEncryptionScope() {
return Codegen.optional(this.defaultEncryptionScope);
}
/**
* Indicates whether the blob container was deleted.
*
*/
@Export(name="deleted", refs={Boolean.class}, tree="[0]")
private Output deleted;
/**
* @return Indicates whether the blob container was deleted.
*
*/
public Output deleted() {
return this.deleted;
}
/**
* Blob container deletion time.
*
*/
@Export(name="deletedTime", refs={String.class}, tree="[0]")
private Output deletedTime;
/**
* @return Blob container deletion time.
*
*/
public Output deletedTime() {
return this.deletedTime;
}
/**
* Block override of encryption scope from the container default.
*
*/
@Export(name="denyEncryptionScopeOverride", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> denyEncryptionScopeOverride;
/**
* @return Block override of encryption scope from the container default.
*
*/
public Output> denyEncryptionScopeOverride() {
return Codegen.optional(this.denyEncryptionScopeOverride);
}
/**
* Enable NFSv3 all squash on blob container.
*
*/
@Export(name="enableNfsV3AllSquash", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableNfsV3AllSquash;
/**
* @return Enable NFSv3 all squash on blob container.
*
*/
public Output> enableNfsV3AllSquash() {
return Codegen.optional(this.enableNfsV3AllSquash);
}
/**
* Enable NFSv3 root squash on blob container.
*
*/
@Export(name="enableNfsV3RootSquash", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableNfsV3RootSquash;
/**
* @return Enable NFSv3 root squash on blob container.
*
*/
public Output> enableNfsV3RootSquash() {
return Codegen.optional(this.enableNfsV3RootSquash);
}
/**
* Resource Etag.
*
*/
@Export(name="etag", refs={String.class}, tree="[0]")
private Output etag;
/**
* @return Resource Etag.
*
*/
public Output etag() {
return this.etag;
}
/**
* The hasImmutabilityPolicy public property is set to true by SRP if ImmutabilityPolicy has been created for this container. The hasImmutabilityPolicy public property is set to false by SRP if ImmutabilityPolicy has not been created for this container.
*
*/
@Export(name="hasImmutabilityPolicy", refs={Boolean.class}, tree="[0]")
private Output hasImmutabilityPolicy;
/**
* @return The hasImmutabilityPolicy public property is set to true by SRP if ImmutabilityPolicy has been created for this container. The hasImmutabilityPolicy public property is set to false by SRP if ImmutabilityPolicy has not been created for this container.
*
*/
public Output hasImmutabilityPolicy() {
return this.hasImmutabilityPolicy;
}
/**
* The hasLegalHold public property is set to true by SRP if there are at least one existing tag. The hasLegalHold public property is set to false by SRP if all existing legal hold tags are cleared out. There can be a maximum of 1000 blob containers with hasLegalHold=true for a given account.
*
*/
@Export(name="hasLegalHold", refs={Boolean.class}, tree="[0]")
private Output hasLegalHold;
/**
* @return The hasLegalHold public property is set to true by SRP if there are at least one existing tag. The hasLegalHold public property is set to false by SRP if all existing legal hold tags are cleared out. There can be a maximum of 1000 blob containers with hasLegalHold=true for a given account.
*
*/
public Output hasLegalHold() {
return this.hasLegalHold;
}
/**
* The ImmutabilityPolicy property of the container.
*
*/
@Export(name="immutabilityPolicy", refs={ImmutabilityPolicyPropertiesResponse.class}, tree="[0]")
private Output immutabilityPolicy;
/**
* @return The ImmutabilityPolicy property of the container.
*
*/
public Output immutabilityPolicy() {
return this.immutabilityPolicy;
}
/**
* The object level immutability property of the container. The property is immutable and can only be set to true at the container creation time. Existing containers must undergo a migration process.
*
*/
@Export(name="immutableStorageWithVersioning", refs={ImmutableStorageWithVersioningResponse.class}, tree="[0]")
private Output* @Nullable */ ImmutableStorageWithVersioningResponse> immutableStorageWithVersioning;
/**
* @return The object level immutability property of the container. The property is immutable and can only be set to true at the container creation time. Existing containers must undergo a migration process.
*
*/
public Output> immutableStorageWithVersioning() {
return Codegen.optional(this.immutableStorageWithVersioning);
}
/**
* Returns the date and time the container was last modified.
*
*/
@Export(name="lastModifiedTime", refs={String.class}, tree="[0]")
private Output lastModifiedTime;
/**
* @return Returns the date and time the container was last modified.
*
*/
public Output lastModifiedTime() {
return this.lastModifiedTime;
}
/**
* Specifies whether the lease on a container is of infinite or fixed duration, only when the container is leased.
*
*/
@Export(name="leaseDuration", refs={String.class}, tree="[0]")
private Output leaseDuration;
/**
* @return Specifies whether the lease on a container is of infinite or fixed duration, only when the container is leased.
*
*/
public Output leaseDuration() {
return this.leaseDuration;
}
/**
* Lease state of the container.
*
*/
@Export(name="leaseState", refs={String.class}, tree="[0]")
private Output leaseState;
/**
* @return Lease state of the container.
*
*/
public Output leaseState() {
return this.leaseState;
}
/**
* The lease status of the container.
*
*/
@Export(name="leaseStatus", refs={String.class}, tree="[0]")
private Output leaseStatus;
/**
* @return The lease status of the container.
*
*/
public Output leaseStatus() {
return this.leaseStatus;
}
/**
* The LegalHold property of the container.
*
*/
@Export(name="legalHold", refs={LegalHoldPropertiesResponse.class}, tree="[0]")
private Output legalHold;
/**
* @return The LegalHold property of the container.
*
*/
public Output legalHold() {
return this.legalHold;
}
/**
* A name-value pair to associate with the container as metadata.
*
*/
@Export(name="metadata", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> metadata;
/**
* @return A name-value pair to associate with the container as metadata.
*
*/
public Output>> metadata() {
return Codegen.optional(this.metadata);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* Specifies whether data in the container may be accessed publicly and the level of access.
*
*/
@Export(name="publicAccess", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> publicAccess;
/**
* @return Specifies whether data in the container may be accessed publicly and the level of access.
*
*/
public Output> publicAccess() {
return Codegen.optional(this.publicAccess);
}
/**
* Remaining retention days for soft deleted blob container.
*
*/
@Export(name="remainingRetentionDays", refs={Integer.class}, tree="[0]")
private Output remainingRetentionDays;
/**
* @return Remaining retention days for soft deleted blob container.
*
*/
public Output remainingRetentionDays() {
return this.remainingRetentionDays;
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
* The version of the deleted blob container.
*
*/
@Export(name="version", refs={String.class}, tree="[0]")
private Output version;
/**
* @return The version of the deleted blob container.
*
*/
public Output version() {
return this.version;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public BlobContainer(java.lang.String name) {
this(name, BlobContainerArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public BlobContainer(java.lang.String name, BlobContainerArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public BlobContainer(java.lang.String name, BlobContainerArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:storage:BlobContainer", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private BlobContainer(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:storage:BlobContainer", name, null, makeResourceOptions(options, id), false);
}
private static BlobContainerArgs makeArgs(BlobContainerArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? BlobContainerArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:storage/v20180201:BlobContainer").build()),
Output.of(Alias.builder().type("azure-native:storage/v20180301preview:BlobContainer").build()),
Output.of(Alias.builder().type("azure-native:storage/v20180701:BlobContainer").build()),
Output.of(Alias.builder().type("azure-native:storage/v20181101:BlobContainer").build()),
Output.of(Alias.builder().type("azure-native:storage/v20190401:BlobContainer").build()),
Output.of(Alias.builder().type("azure-native:storage/v20190601:BlobContainer").build()),
Output.of(Alias.builder().type("azure-native:storage/v20200801preview:BlobContainer").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210101:BlobContainer").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210201:BlobContainer").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210401:BlobContainer").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210601:BlobContainer").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210801:BlobContainer").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210901:BlobContainer").build()),
Output.of(Alias.builder().type("azure-native:storage/v20220501:BlobContainer").build()),
Output.of(Alias.builder().type("azure-native:storage/v20220901:BlobContainer").build()),
Output.of(Alias.builder().type("azure-native:storage/v20230101:BlobContainer").build()),
Output.of(Alias.builder().type("azure-native:storage/v20230401:BlobContainer").build()),
Output.of(Alias.builder().type("azure-native:storage/v20230501:BlobContainer").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static BlobContainer get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new BlobContainer(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy