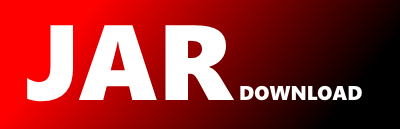
com.pulumi.azurenative.storage.outputs.EncryptionResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storage.outputs;
import com.pulumi.azurenative.storage.outputs.EncryptionIdentityResponse;
import com.pulumi.azurenative.storage.outputs.EncryptionServicesResponse;
import com.pulumi.azurenative.storage.outputs.KeyVaultPropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class EncryptionResponse {
/**
* @return The identity to be used with service-side encryption at rest.
*
*/
private @Nullable EncryptionIdentityResponse encryptionIdentity;
/**
* @return The encryption keySource (provider). Possible values (case-insensitive): Microsoft.Storage, Microsoft.Keyvault
*
*/
private @Nullable String keySource;
/**
* @return Properties provided by key vault.
*
*/
private @Nullable KeyVaultPropertiesResponse keyVaultProperties;
/**
* @return A boolean indicating whether or not the service applies a secondary layer of encryption with platform managed keys for data at rest.
*
*/
private @Nullable Boolean requireInfrastructureEncryption;
/**
* @return List of services which support encryption.
*
*/
private @Nullable EncryptionServicesResponse services;
private EncryptionResponse() {}
/**
* @return The identity to be used with service-side encryption at rest.
*
*/
public Optional encryptionIdentity() {
return Optional.ofNullable(this.encryptionIdentity);
}
/**
* @return The encryption keySource (provider). Possible values (case-insensitive): Microsoft.Storage, Microsoft.Keyvault
*
*/
public Optional keySource() {
return Optional.ofNullable(this.keySource);
}
/**
* @return Properties provided by key vault.
*
*/
public Optional keyVaultProperties() {
return Optional.ofNullable(this.keyVaultProperties);
}
/**
* @return A boolean indicating whether or not the service applies a secondary layer of encryption with platform managed keys for data at rest.
*
*/
public Optional requireInfrastructureEncryption() {
return Optional.ofNullable(this.requireInfrastructureEncryption);
}
/**
* @return List of services which support encryption.
*
*/
public Optional services() {
return Optional.ofNullable(this.services);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(EncryptionResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable EncryptionIdentityResponse encryptionIdentity;
private @Nullable String keySource;
private @Nullable KeyVaultPropertiesResponse keyVaultProperties;
private @Nullable Boolean requireInfrastructureEncryption;
private @Nullable EncryptionServicesResponse services;
public Builder() {}
public Builder(EncryptionResponse defaults) {
Objects.requireNonNull(defaults);
this.encryptionIdentity = defaults.encryptionIdentity;
this.keySource = defaults.keySource;
this.keyVaultProperties = defaults.keyVaultProperties;
this.requireInfrastructureEncryption = defaults.requireInfrastructureEncryption;
this.services = defaults.services;
}
@CustomType.Setter
public Builder encryptionIdentity(@Nullable EncryptionIdentityResponse encryptionIdentity) {
this.encryptionIdentity = encryptionIdentity;
return this;
}
@CustomType.Setter
public Builder keySource(@Nullable String keySource) {
this.keySource = keySource;
return this;
}
@CustomType.Setter
public Builder keyVaultProperties(@Nullable KeyVaultPropertiesResponse keyVaultProperties) {
this.keyVaultProperties = keyVaultProperties;
return this;
}
@CustomType.Setter
public Builder requireInfrastructureEncryption(@Nullable Boolean requireInfrastructureEncryption) {
this.requireInfrastructureEncryption = requireInfrastructureEncryption;
return this;
}
@CustomType.Setter
public Builder services(@Nullable EncryptionServicesResponse services) {
this.services = services;
return this;
}
public EncryptionResponse build() {
final var _resultValue = new EncryptionResponse();
_resultValue.encryptionIdentity = encryptionIdentity;
_resultValue.keySource = keySource;
_resultValue.keyVaultProperties = keyVaultProperties;
_resultValue.requireInfrastructureEncryption = requireInfrastructureEncryption;
_resultValue.services = services;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy