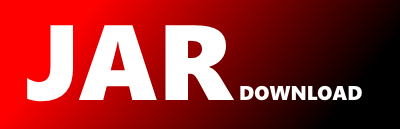
com.pulumi.azurenative.storagepool.outputs.GetIscsiTargetResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storagepool.outputs;
import com.pulumi.azurenative.storagepool.outputs.AclResponse;
import com.pulumi.azurenative.storagepool.outputs.IscsiLunResponse;
import com.pulumi.azurenative.storagepool.outputs.SystemMetadataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetIscsiTargetResult {
/**
* @return Mode for Target connectivity.
*
*/
private String aclMode;
/**
* @return List of private IPv4 addresses to connect to the iSCSI Target.
*
*/
private @Nullable List endpoints;
/**
* @return Fully qualified resource Id for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return List of LUNs to be exposed through iSCSI Target.
*
*/
private @Nullable List luns;
/**
* @return Azure resource id. Indicates if this resource is managed by another Azure resource.
*
*/
private String managedBy;
/**
* @return List of Azure resource ids that manage this resource.
*
*/
private List managedByExtended;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The port used by iSCSI Target portal group.
*
*/
private @Nullable Integer port;
/**
* @return State of the operation on the resource.
*
*/
private String provisioningState;
/**
* @return List of identifiers for active sessions on the iSCSI target
*
*/
private List sessions;
/**
* @return Access Control List (ACL) for an iSCSI Target; defines LUN masking policy
*
*/
private @Nullable List staticAcls;
/**
* @return Operational status of the iSCSI Target.
*
*/
private String status;
/**
* @return Resource metadata required by ARM RPC
*
*/
private SystemMetadataResponse systemData;
/**
* @return iSCSI Target IQN (iSCSI Qualified Name); example: "iqn.2005-03.org.iscsi:server".
*
*/
private String targetIqn;
/**
* @return The type of the resource. Ex- Microsoft.Compute/virtualMachines or Microsoft.Storage/storageAccounts.
*
*/
private String type;
private GetIscsiTargetResult() {}
/**
* @return Mode for Target connectivity.
*
*/
public String aclMode() {
return this.aclMode;
}
/**
* @return List of private IPv4 addresses to connect to the iSCSI Target.
*
*/
public List endpoints() {
return this.endpoints == null ? List.of() : this.endpoints;
}
/**
* @return Fully qualified resource Id for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return List of LUNs to be exposed through iSCSI Target.
*
*/
public List luns() {
return this.luns == null ? List.of() : this.luns;
}
/**
* @return Azure resource id. Indicates if this resource is managed by another Azure resource.
*
*/
public String managedBy() {
return this.managedBy;
}
/**
* @return List of Azure resource ids that manage this resource.
*
*/
public List managedByExtended() {
return this.managedByExtended;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return The port used by iSCSI Target portal group.
*
*/
public Optional port() {
return Optional.ofNullable(this.port);
}
/**
* @return State of the operation on the resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return List of identifiers for active sessions on the iSCSI target
*
*/
public List sessions() {
return this.sessions;
}
/**
* @return Access Control List (ACL) for an iSCSI Target; defines LUN masking policy
*
*/
public List staticAcls() {
return this.staticAcls == null ? List.of() : this.staticAcls;
}
/**
* @return Operational status of the iSCSI Target.
*
*/
public String status() {
return this.status;
}
/**
* @return Resource metadata required by ARM RPC
*
*/
public SystemMetadataResponse systemData() {
return this.systemData;
}
/**
* @return iSCSI Target IQN (iSCSI Qualified Name); example: "iqn.2005-03.org.iscsi:server".
*
*/
public String targetIqn() {
return this.targetIqn;
}
/**
* @return The type of the resource. Ex- Microsoft.Compute/virtualMachines or Microsoft.Storage/storageAccounts.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetIscsiTargetResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String aclMode;
private @Nullable List endpoints;
private String id;
private @Nullable List luns;
private String managedBy;
private List managedByExtended;
private String name;
private @Nullable Integer port;
private String provisioningState;
private List sessions;
private @Nullable List staticAcls;
private String status;
private SystemMetadataResponse systemData;
private String targetIqn;
private String type;
public Builder() {}
public Builder(GetIscsiTargetResult defaults) {
Objects.requireNonNull(defaults);
this.aclMode = defaults.aclMode;
this.endpoints = defaults.endpoints;
this.id = defaults.id;
this.luns = defaults.luns;
this.managedBy = defaults.managedBy;
this.managedByExtended = defaults.managedByExtended;
this.name = defaults.name;
this.port = defaults.port;
this.provisioningState = defaults.provisioningState;
this.sessions = defaults.sessions;
this.staticAcls = defaults.staticAcls;
this.status = defaults.status;
this.systemData = defaults.systemData;
this.targetIqn = defaults.targetIqn;
this.type = defaults.type;
}
@CustomType.Setter
public Builder aclMode(String aclMode) {
if (aclMode == null) {
throw new MissingRequiredPropertyException("GetIscsiTargetResult", "aclMode");
}
this.aclMode = aclMode;
return this;
}
@CustomType.Setter
public Builder endpoints(@Nullable List endpoints) {
this.endpoints = endpoints;
return this;
}
public Builder endpoints(String... endpoints) {
return endpoints(List.of(endpoints));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetIscsiTargetResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder luns(@Nullable List luns) {
this.luns = luns;
return this;
}
public Builder luns(IscsiLunResponse... luns) {
return luns(List.of(luns));
}
@CustomType.Setter
public Builder managedBy(String managedBy) {
if (managedBy == null) {
throw new MissingRequiredPropertyException("GetIscsiTargetResult", "managedBy");
}
this.managedBy = managedBy;
return this;
}
@CustomType.Setter
public Builder managedByExtended(List managedByExtended) {
if (managedByExtended == null) {
throw new MissingRequiredPropertyException("GetIscsiTargetResult", "managedByExtended");
}
this.managedByExtended = managedByExtended;
return this;
}
public Builder managedByExtended(String... managedByExtended) {
return managedByExtended(List.of(managedByExtended));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetIscsiTargetResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder port(@Nullable Integer port) {
this.port = port;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetIscsiTargetResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder sessions(List sessions) {
if (sessions == null) {
throw new MissingRequiredPropertyException("GetIscsiTargetResult", "sessions");
}
this.sessions = sessions;
return this;
}
public Builder sessions(String... sessions) {
return sessions(List.of(sessions));
}
@CustomType.Setter
public Builder staticAcls(@Nullable List staticAcls) {
this.staticAcls = staticAcls;
return this;
}
public Builder staticAcls(AclResponse... staticAcls) {
return staticAcls(List.of(staticAcls));
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetIscsiTargetResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder systemData(SystemMetadataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetIscsiTargetResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder targetIqn(String targetIqn) {
if (targetIqn == null) {
throw new MissingRequiredPropertyException("GetIscsiTargetResult", "targetIqn");
}
this.targetIqn = targetIqn;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetIscsiTargetResult", "type");
}
this.type = type;
return this;
}
public GetIscsiTargetResult build() {
final var _resultValue = new GetIscsiTargetResult();
_resultValue.aclMode = aclMode;
_resultValue.endpoints = endpoints;
_resultValue.id = id;
_resultValue.luns = luns;
_resultValue.managedBy = managedBy;
_resultValue.managedByExtended = managedByExtended;
_resultValue.name = name;
_resultValue.port = port;
_resultValue.provisioningState = provisioningState;
_resultValue.sessions = sessions;
_resultValue.staticAcls = staticAcls;
_resultValue.status = status;
_resultValue.systemData = systemData;
_resultValue.targetIqn = targetIqn;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy