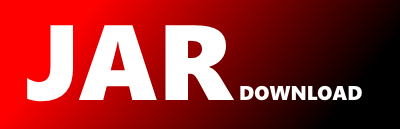
com.pulumi.azurenative.streamanalytics.outputs.AzureDataLakeStoreOutputDataSourceResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.streamanalytics.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AzureDataLakeStoreOutputDataSourceResponse {
/**
* @return The name of the Azure Data Lake Store account. Required on PUT (CreateOrReplace) requests.
*
*/
private @Nullable String accountName;
/**
* @return Authentication Mode.
*
*/
private @Nullable String authenticationMode;
/**
* @return The date format. Wherever {date} appears in filePathPrefix, the value of this property is used as the date format instead.
*
*/
private @Nullable String dateFormat;
/**
* @return The location of the file to which the output should be written to. Required on PUT (CreateOrReplace) requests.
*
*/
private @Nullable String filePathPrefix;
/**
* @return A refresh token that can be used to obtain a valid access token that can then be used to authenticate with the data source. A valid refresh token is currently only obtainable via the Azure Portal. It is recommended to put a dummy string value here when creating the data source and then going to the Azure Portal to authenticate the data source which will update this property with a valid refresh token. Required on PUT (CreateOrReplace) requests.
*
*/
private @Nullable String refreshToken;
/**
* @return The tenant id of the user used to obtain the refresh token. Required on PUT (CreateOrReplace) requests.
*
*/
private @Nullable String tenantId;
/**
* @return The time format. Wherever {time} appears in filePathPrefix, the value of this property is used as the time format instead.
*
*/
private @Nullable String timeFormat;
/**
* @return The user display name of the user that was used to obtain the refresh token. Use this property to help remember which user was used to obtain the refresh token.
*
*/
private @Nullable String tokenUserDisplayName;
/**
* @return The user principal name (UPN) of the user that was used to obtain the refresh token. Use this property to help remember which user was used to obtain the refresh token.
*
*/
private @Nullable String tokenUserPrincipalName;
/**
* @return Indicates the type of data source output will be written to. Required on PUT (CreateOrReplace) requests.
* Expected value is 'Microsoft.DataLake/Accounts'.
*
*/
private String type;
private AzureDataLakeStoreOutputDataSourceResponse() {}
/**
* @return The name of the Azure Data Lake Store account. Required on PUT (CreateOrReplace) requests.
*
*/
public Optional accountName() {
return Optional.ofNullable(this.accountName);
}
/**
* @return Authentication Mode.
*
*/
public Optional authenticationMode() {
return Optional.ofNullable(this.authenticationMode);
}
/**
* @return The date format. Wherever {date} appears in filePathPrefix, the value of this property is used as the date format instead.
*
*/
public Optional dateFormat() {
return Optional.ofNullable(this.dateFormat);
}
/**
* @return The location of the file to which the output should be written to. Required on PUT (CreateOrReplace) requests.
*
*/
public Optional filePathPrefix() {
return Optional.ofNullable(this.filePathPrefix);
}
/**
* @return A refresh token that can be used to obtain a valid access token that can then be used to authenticate with the data source. A valid refresh token is currently only obtainable via the Azure Portal. It is recommended to put a dummy string value here when creating the data source and then going to the Azure Portal to authenticate the data source which will update this property with a valid refresh token. Required on PUT (CreateOrReplace) requests.
*
*/
public Optional refreshToken() {
return Optional.ofNullable(this.refreshToken);
}
/**
* @return The tenant id of the user used to obtain the refresh token. Required on PUT (CreateOrReplace) requests.
*
*/
public Optional tenantId() {
return Optional.ofNullable(this.tenantId);
}
/**
* @return The time format. Wherever {time} appears in filePathPrefix, the value of this property is used as the time format instead.
*
*/
public Optional timeFormat() {
return Optional.ofNullable(this.timeFormat);
}
/**
* @return The user display name of the user that was used to obtain the refresh token. Use this property to help remember which user was used to obtain the refresh token.
*
*/
public Optional tokenUserDisplayName() {
return Optional.ofNullable(this.tokenUserDisplayName);
}
/**
* @return The user principal name (UPN) of the user that was used to obtain the refresh token. Use this property to help remember which user was used to obtain the refresh token.
*
*/
public Optional tokenUserPrincipalName() {
return Optional.ofNullable(this.tokenUserPrincipalName);
}
/**
* @return Indicates the type of data source output will be written to. Required on PUT (CreateOrReplace) requests.
* Expected value is 'Microsoft.DataLake/Accounts'.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AzureDataLakeStoreOutputDataSourceResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String accountName;
private @Nullable String authenticationMode;
private @Nullable String dateFormat;
private @Nullable String filePathPrefix;
private @Nullable String refreshToken;
private @Nullable String tenantId;
private @Nullable String timeFormat;
private @Nullable String tokenUserDisplayName;
private @Nullable String tokenUserPrincipalName;
private String type;
public Builder() {}
public Builder(AzureDataLakeStoreOutputDataSourceResponse defaults) {
Objects.requireNonNull(defaults);
this.accountName = defaults.accountName;
this.authenticationMode = defaults.authenticationMode;
this.dateFormat = defaults.dateFormat;
this.filePathPrefix = defaults.filePathPrefix;
this.refreshToken = defaults.refreshToken;
this.tenantId = defaults.tenantId;
this.timeFormat = defaults.timeFormat;
this.tokenUserDisplayName = defaults.tokenUserDisplayName;
this.tokenUserPrincipalName = defaults.tokenUserPrincipalName;
this.type = defaults.type;
}
@CustomType.Setter
public Builder accountName(@Nullable String accountName) {
this.accountName = accountName;
return this;
}
@CustomType.Setter
public Builder authenticationMode(@Nullable String authenticationMode) {
this.authenticationMode = authenticationMode;
return this;
}
@CustomType.Setter
public Builder dateFormat(@Nullable String dateFormat) {
this.dateFormat = dateFormat;
return this;
}
@CustomType.Setter
public Builder filePathPrefix(@Nullable String filePathPrefix) {
this.filePathPrefix = filePathPrefix;
return this;
}
@CustomType.Setter
public Builder refreshToken(@Nullable String refreshToken) {
this.refreshToken = refreshToken;
return this;
}
@CustomType.Setter
public Builder tenantId(@Nullable String tenantId) {
this.tenantId = tenantId;
return this;
}
@CustomType.Setter
public Builder timeFormat(@Nullable String timeFormat) {
this.timeFormat = timeFormat;
return this;
}
@CustomType.Setter
public Builder tokenUserDisplayName(@Nullable String tokenUserDisplayName) {
this.tokenUserDisplayName = tokenUserDisplayName;
return this;
}
@CustomType.Setter
public Builder tokenUserPrincipalName(@Nullable String tokenUserPrincipalName) {
this.tokenUserPrincipalName = tokenUserPrincipalName;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("AzureDataLakeStoreOutputDataSourceResponse", "type");
}
this.type = type;
return this;
}
public AzureDataLakeStoreOutputDataSourceResponse build() {
final var _resultValue = new AzureDataLakeStoreOutputDataSourceResponse();
_resultValue.accountName = accountName;
_resultValue.authenticationMode = authenticationMode;
_resultValue.dateFormat = dateFormat;
_resultValue.filePathPrefix = filePathPrefix;
_resultValue.refreshToken = refreshToken;
_resultValue.tenantId = tenantId;
_resultValue.timeFormat = timeFormat;
_resultValue.tokenUserDisplayName = tokenUserDisplayName;
_resultValue.tokenUserPrincipalName = tokenUserPrincipalName;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy