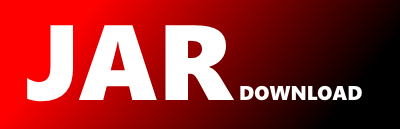
com.pulumi.azurenative.streamanalytics.outputs.GetClusterResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.streamanalytics.outputs;
import com.pulumi.azurenative.streamanalytics.outputs.ClusterSkuResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetClusterResult {
/**
* @return Represents the number of streaming units currently being used on the cluster.
*
*/
private Integer capacityAllocated;
/**
* @return Represents the sum of the SUs of all streaming jobs associated with the cluster. If all of the jobs were running, this would be the capacity allocated.
*
*/
private Integer capacityAssigned;
/**
* @return Unique identifier for the cluster.
*
*/
private String clusterId;
/**
* @return The date this cluster was created.
*
*/
private String createdDate;
/**
* @return The current entity tag for the cluster. This is an opaque string. You can use it to detect whether the resource has changed between requests. You can also use it in the If-Match or If-None-Match headers for write operations for optimistic concurrency.
*
*/
private String etag;
/**
* @return Fully qualified resource Id for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The geo-location where the resource lives
*
*/
private @Nullable String location;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The status of the cluster provisioning. The three terminal states are: Succeeded, Failed and Canceled
*
*/
private String provisioningState;
/**
* @return The SKU of the cluster. This determines the size/capacity of the cluster. Required on PUT (CreateOrUpdate) requests.
*
*/
private @Nullable ClusterSkuResponse sku;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource. Ex- Microsoft.Compute/virtualMachines or Microsoft.Storage/storageAccounts.
*
*/
private String type;
private GetClusterResult() {}
/**
* @return Represents the number of streaming units currently being used on the cluster.
*
*/
public Integer capacityAllocated() {
return this.capacityAllocated;
}
/**
* @return Represents the sum of the SUs of all streaming jobs associated with the cluster. If all of the jobs were running, this would be the capacity allocated.
*
*/
public Integer capacityAssigned() {
return this.capacityAssigned;
}
/**
* @return Unique identifier for the cluster.
*
*/
public String clusterId() {
return this.clusterId;
}
/**
* @return The date this cluster was created.
*
*/
public String createdDate() {
return this.createdDate;
}
/**
* @return The current entity tag for the cluster. This is an opaque string. You can use it to detect whether the resource has changed between requests. You can also use it in the If-Match or If-None-Match headers for write operations for optimistic concurrency.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Fully qualified resource Id for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The geo-location where the resource lives
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return The status of the cluster provisioning. The three terminal states are: Succeeded, Failed and Canceled
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The SKU of the cluster. This determines the size/capacity of the cluster. Required on PUT (CreateOrUpdate) requests.
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource. Ex- Microsoft.Compute/virtualMachines or Microsoft.Storage/storageAccounts.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetClusterResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Integer capacityAllocated;
private Integer capacityAssigned;
private String clusterId;
private String createdDate;
private String etag;
private String id;
private @Nullable String location;
private String name;
private String provisioningState;
private @Nullable ClusterSkuResponse sku;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetClusterResult defaults) {
Objects.requireNonNull(defaults);
this.capacityAllocated = defaults.capacityAllocated;
this.capacityAssigned = defaults.capacityAssigned;
this.clusterId = defaults.clusterId;
this.createdDate = defaults.createdDate;
this.etag = defaults.etag;
this.id = defaults.id;
this.location = defaults.location;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.sku = defaults.sku;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder capacityAllocated(Integer capacityAllocated) {
if (capacityAllocated == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "capacityAllocated");
}
this.capacityAllocated = capacityAllocated;
return this;
}
@CustomType.Setter
public Builder capacityAssigned(Integer capacityAssigned) {
if (capacityAssigned == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "capacityAssigned");
}
this.capacityAssigned = capacityAssigned;
return this;
}
@CustomType.Setter
public Builder clusterId(String clusterId) {
if (clusterId == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "clusterId");
}
this.clusterId = clusterId;
return this;
}
@CustomType.Setter
public Builder createdDate(String createdDate) {
if (createdDate == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "createdDate");
}
this.createdDate = createdDate;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable ClusterSkuResponse sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "type");
}
this.type = type;
return this;
}
public GetClusterResult build() {
final var _resultValue = new GetClusterResult();
_resultValue.capacityAllocated = capacityAllocated;
_resultValue.capacityAssigned = capacityAssigned;
_resultValue.clusterId = clusterId;
_resultValue.createdDate = createdDate;
_resultValue.etag = etag;
_resultValue.id = id;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.sku = sku;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy