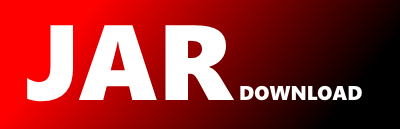
com.pulumi.azurenative.synapse.BigDataPoolArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.synapse;
import com.pulumi.azurenative.synapse.enums.NodeSize;
import com.pulumi.azurenative.synapse.enums.NodeSizeFamily;
import com.pulumi.azurenative.synapse.inputs.AutoPausePropertiesArgs;
import com.pulumi.azurenative.synapse.inputs.AutoScalePropertiesArgs;
import com.pulumi.azurenative.synapse.inputs.DynamicExecutorAllocationArgs;
import com.pulumi.azurenative.synapse.inputs.LibraryInfoArgs;
import com.pulumi.azurenative.synapse.inputs.LibraryRequirementsArgs;
import com.pulumi.azurenative.synapse.inputs.SparkConfigPropertiesArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class BigDataPoolArgs extends com.pulumi.resources.ResourceArgs {
public static final BigDataPoolArgs Empty = new BigDataPoolArgs();
/**
* Auto-pausing properties
*
*/
@Import(name="autoPause")
private @Nullable Output autoPause;
/**
* @return Auto-pausing properties
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy