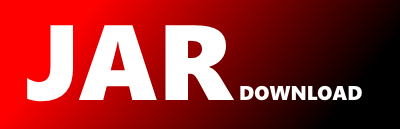
com.pulumi.azurenative.testbase.outputs.GetCustomImageResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.testbase.outputs;
import com.pulumi.azurenative.testbase.outputs.ImageValidationResultsResponse;
import com.pulumi.azurenative.testbase.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetCustomImageResult {
/**
* @return The UTC timestamp when the custom image was published.
*
*/
private String creationTime;
/**
* @return Image definition name.
*
*/
private String definitionName;
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
private String id;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return This property indicates the size of the VHD to be created.
*
*/
private Double osDiskImageSizeInGB;
/**
* @return Product of the custom image.
*
*/
private String product;
/**
* @return The provisioning state of the resource.
*
*/
private String provisioningState;
/**
* @return Release of the custom image OS.
*
*/
private String release;
/**
* @return The release version date of the release of the custom image OS.
*
*/
private String releaseVersionDate;
/**
* @return Custom image source type.
*
*/
private String source;
/**
* @return Status of the custom image.
*
*/
private String status;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return The validation result of the custom image.
*
*/
private ImageValidationResultsResponse validationResults;
/**
* @return Image version name.
*
*/
private String versionName;
/**
* @return The file name of the associated VHD resource.
*
*/
private String vhdFileName;
/**
* @return The Id of the associated VHD resource.
*
*/
private @Nullable String vhdId;
private GetCustomImageResult() {}
/**
* @return The UTC timestamp when the custom image was published.
*
*/
public String creationTime() {
return this.creationTime;
}
/**
* @return Image definition name.
*
*/
public String definitionName() {
return this.definitionName;
}
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
public String id() {
return this.id;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return This property indicates the size of the VHD to be created.
*
*/
public Double osDiskImageSizeInGB() {
return this.osDiskImageSizeInGB;
}
/**
* @return Product of the custom image.
*
*/
public String product() {
return this.product;
}
/**
* @return The provisioning state of the resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Release of the custom image OS.
*
*/
public String release() {
return this.release;
}
/**
* @return The release version date of the release of the custom image OS.
*
*/
public String releaseVersionDate() {
return this.releaseVersionDate;
}
/**
* @return Custom image source type.
*
*/
public String source() {
return this.source;
}
/**
* @return Status of the custom image.
*
*/
public String status() {
return this.status;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return The validation result of the custom image.
*
*/
public ImageValidationResultsResponse validationResults() {
return this.validationResults;
}
/**
* @return Image version name.
*
*/
public String versionName() {
return this.versionName;
}
/**
* @return The file name of the associated VHD resource.
*
*/
public String vhdFileName() {
return this.vhdFileName;
}
/**
* @return The Id of the associated VHD resource.
*
*/
public Optional vhdId() {
return Optional.ofNullable(this.vhdId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetCustomImageResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String creationTime;
private String definitionName;
private String id;
private String name;
private Double osDiskImageSizeInGB;
private String product;
private String provisioningState;
private String release;
private String releaseVersionDate;
private String source;
private String status;
private SystemDataResponse systemData;
private String type;
private ImageValidationResultsResponse validationResults;
private String versionName;
private String vhdFileName;
private @Nullable String vhdId;
public Builder() {}
public Builder(GetCustomImageResult defaults) {
Objects.requireNonNull(defaults);
this.creationTime = defaults.creationTime;
this.definitionName = defaults.definitionName;
this.id = defaults.id;
this.name = defaults.name;
this.osDiskImageSizeInGB = defaults.osDiskImageSizeInGB;
this.product = defaults.product;
this.provisioningState = defaults.provisioningState;
this.release = defaults.release;
this.releaseVersionDate = defaults.releaseVersionDate;
this.source = defaults.source;
this.status = defaults.status;
this.systemData = defaults.systemData;
this.type = defaults.type;
this.validationResults = defaults.validationResults;
this.versionName = defaults.versionName;
this.vhdFileName = defaults.vhdFileName;
this.vhdId = defaults.vhdId;
}
@CustomType.Setter
public Builder creationTime(String creationTime) {
if (creationTime == null) {
throw new MissingRequiredPropertyException("GetCustomImageResult", "creationTime");
}
this.creationTime = creationTime;
return this;
}
@CustomType.Setter
public Builder definitionName(String definitionName) {
if (definitionName == null) {
throw new MissingRequiredPropertyException("GetCustomImageResult", "definitionName");
}
this.definitionName = definitionName;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetCustomImageResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetCustomImageResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder osDiskImageSizeInGB(Double osDiskImageSizeInGB) {
if (osDiskImageSizeInGB == null) {
throw new MissingRequiredPropertyException("GetCustomImageResult", "osDiskImageSizeInGB");
}
this.osDiskImageSizeInGB = osDiskImageSizeInGB;
return this;
}
@CustomType.Setter
public Builder product(String product) {
if (product == null) {
throw new MissingRequiredPropertyException("GetCustomImageResult", "product");
}
this.product = product;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetCustomImageResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder release(String release) {
if (release == null) {
throw new MissingRequiredPropertyException("GetCustomImageResult", "release");
}
this.release = release;
return this;
}
@CustomType.Setter
public Builder releaseVersionDate(String releaseVersionDate) {
if (releaseVersionDate == null) {
throw new MissingRequiredPropertyException("GetCustomImageResult", "releaseVersionDate");
}
this.releaseVersionDate = releaseVersionDate;
return this;
}
@CustomType.Setter
public Builder source(String source) {
if (source == null) {
throw new MissingRequiredPropertyException("GetCustomImageResult", "source");
}
this.source = source;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetCustomImageResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetCustomImageResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetCustomImageResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder validationResults(ImageValidationResultsResponse validationResults) {
if (validationResults == null) {
throw new MissingRequiredPropertyException("GetCustomImageResult", "validationResults");
}
this.validationResults = validationResults;
return this;
}
@CustomType.Setter
public Builder versionName(String versionName) {
if (versionName == null) {
throw new MissingRequiredPropertyException("GetCustomImageResult", "versionName");
}
this.versionName = versionName;
return this;
}
@CustomType.Setter
public Builder vhdFileName(String vhdFileName) {
if (vhdFileName == null) {
throw new MissingRequiredPropertyException("GetCustomImageResult", "vhdFileName");
}
this.vhdFileName = vhdFileName;
return this;
}
@CustomType.Setter
public Builder vhdId(@Nullable String vhdId) {
this.vhdId = vhdId;
return this;
}
public GetCustomImageResult build() {
final var _resultValue = new GetCustomImageResult();
_resultValue.creationTime = creationTime;
_resultValue.definitionName = definitionName;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.osDiskImageSizeInGB = osDiskImageSizeInGB;
_resultValue.product = product;
_resultValue.provisioningState = provisioningState;
_resultValue.release = release;
_resultValue.releaseVersionDate = releaseVersionDate;
_resultValue.source = source;
_resultValue.status = status;
_resultValue.systemData = systemData;
_resultValue.type = type;
_resultValue.validationResults = validationResults;
_resultValue.versionName = versionName;
_resultValue.vhdFileName = vhdFileName;
_resultValue.vhdId = vhdId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy