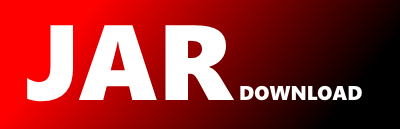
com.pulumi.azurenative.testbase.outputs.TargetOSInfoResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.testbase.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class TargetOSInfoResponse {
/**
* @return Specifies the baseline OSs to be tested.
*
*/
private @Nullable List baselineOSs;
/**
* @return Insider Channel Ids. Only used for feature update.
*
*/
private @Nullable List insiderChannelIds;
/**
* @return Specifies the OS update type to test against, e.g., 'Security updates' or 'Feature updates'.
*
*/
private String osUpdateType;
/**
* @return Specifies the ids of the target OSs from Custom Images to be tested.
*
*/
private @Nullable List targetOSImageIds;
/**
* @return Specifies the target OSs to be tested.
*
*/
private List targetOSs;
private TargetOSInfoResponse() {}
/**
* @return Specifies the baseline OSs to be tested.
*
*/
public List baselineOSs() {
return this.baselineOSs == null ? List.of() : this.baselineOSs;
}
/**
* @return Insider Channel Ids. Only used for feature update.
*
*/
public List insiderChannelIds() {
return this.insiderChannelIds == null ? List.of() : this.insiderChannelIds;
}
/**
* @return Specifies the OS update type to test against, e.g., 'Security updates' or 'Feature updates'.
*
*/
public String osUpdateType() {
return this.osUpdateType;
}
/**
* @return Specifies the ids of the target OSs from Custom Images to be tested.
*
*/
public List targetOSImageIds() {
return this.targetOSImageIds == null ? List.of() : this.targetOSImageIds;
}
/**
* @return Specifies the target OSs to be tested.
*
*/
public List targetOSs() {
return this.targetOSs;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(TargetOSInfoResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List baselineOSs;
private @Nullable List insiderChannelIds;
private String osUpdateType;
private @Nullable List targetOSImageIds;
private List targetOSs;
public Builder() {}
public Builder(TargetOSInfoResponse defaults) {
Objects.requireNonNull(defaults);
this.baselineOSs = defaults.baselineOSs;
this.insiderChannelIds = defaults.insiderChannelIds;
this.osUpdateType = defaults.osUpdateType;
this.targetOSImageIds = defaults.targetOSImageIds;
this.targetOSs = defaults.targetOSs;
}
@CustomType.Setter
public Builder baselineOSs(@Nullable List baselineOSs) {
this.baselineOSs = baselineOSs;
return this;
}
public Builder baselineOSs(String... baselineOSs) {
return baselineOSs(List.of(baselineOSs));
}
@CustomType.Setter
public Builder insiderChannelIds(@Nullable List insiderChannelIds) {
this.insiderChannelIds = insiderChannelIds;
return this;
}
public Builder insiderChannelIds(String... insiderChannelIds) {
return insiderChannelIds(List.of(insiderChannelIds));
}
@CustomType.Setter
public Builder osUpdateType(String osUpdateType) {
if (osUpdateType == null) {
throw new MissingRequiredPropertyException("TargetOSInfoResponse", "osUpdateType");
}
this.osUpdateType = osUpdateType;
return this;
}
@CustomType.Setter
public Builder targetOSImageIds(@Nullable List targetOSImageIds) {
this.targetOSImageIds = targetOSImageIds;
return this;
}
public Builder targetOSImageIds(String... targetOSImageIds) {
return targetOSImageIds(List.of(targetOSImageIds));
}
@CustomType.Setter
public Builder targetOSs(List targetOSs) {
if (targetOSs == null) {
throw new MissingRequiredPropertyException("TargetOSInfoResponse", "targetOSs");
}
this.targetOSs = targetOSs;
return this;
}
public Builder targetOSs(String... targetOSs) {
return targetOSs(List.of(targetOSs));
}
public TargetOSInfoResponse build() {
final var _resultValue = new TargetOSInfoResponse();
_resultValue.baselineOSs = baselineOSs;
_resultValue.insiderChannelIds = insiderChannelIds;
_resultValue.osUpdateType = osUpdateType;
_resultValue.targetOSImageIds = targetOSImageIds;
_resultValue.targetOSs = targetOSs;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy