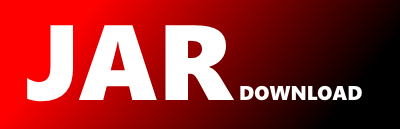
com.pulumi.azurenative.timeseriesinsights.Gen1Environment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.timeseriesinsights;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.timeseriesinsights.Gen1EnvironmentArgs;
import com.pulumi.azurenative.timeseriesinsights.outputs.EnvironmentStatusResponse;
import com.pulumi.azurenative.timeseriesinsights.outputs.SkuResponse;
import com.pulumi.azurenative.timeseriesinsights.outputs.TimeSeriesIdPropertyResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* An environment is a set of time-series data available for query, and is the top level Azure Time Series Insights resource. Gen1 environments have data retention limits.
* Azure REST API version: 2020-05-15. Prior API version in Azure Native 1.x: 2020-05-15.
*
* ## Example Usage
* ### EnvironmentsCreate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.timeseriesinsights.Gen1Environment;
* import com.pulumi.azurenative.timeseriesinsights.Gen1EnvironmentArgs;
* import com.pulumi.azurenative.timeseriesinsights.inputs.TimeSeriesIdPropertyArgs;
* import com.pulumi.azurenative.timeseriesinsights.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var gen1Environment = new Gen1Environment("gen1Environment", Gen1EnvironmentArgs.builder()
* .dataRetentionTime("P31D")
* .environmentName("env1")
* .kind("Gen1")
* .location("West US")
* .partitionKeyProperties(TimeSeriesIdPropertyArgs.builder()
* .name("DeviceId1")
* .type("String")
* .build())
* .resourceGroupName("rg1")
* .sku(SkuArgs.builder()
* .capacity(1)
* .name("S1")
* .build())
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:timeseriesinsights:Gen1Environment env1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.TimeSeriesInsights/environments/{environmentName}
* ```
*
*/
@ResourceType(type="azure-native:timeseriesinsights:Gen1Environment")
public class Gen1Environment extends com.pulumi.resources.CustomResource {
/**
* The time the resource was created.
*
*/
@Export(name="creationTime", refs={String.class}, tree="[0]")
private Output creationTime;
/**
* @return The time the resource was created.
*
*/
public Output creationTime() {
return this.creationTime;
}
/**
* The fully qualified domain name used to access the environment data, e.g. to query the environment's events or upload reference data for the environment.
*
*/
@Export(name="dataAccessFqdn", refs={String.class}, tree="[0]")
private Output dataAccessFqdn;
/**
* @return The fully qualified domain name used to access the environment data, e.g. to query the environment's events or upload reference data for the environment.
*
*/
public Output dataAccessFqdn() {
return this.dataAccessFqdn;
}
/**
* An id used to access the environment data, e.g. to query the environment's events or upload reference data for the environment.
*
*/
@Export(name="dataAccessId", refs={String.class}, tree="[0]")
private Output dataAccessId;
/**
* @return An id used to access the environment data, e.g. to query the environment's events or upload reference data for the environment.
*
*/
public Output dataAccessId() {
return this.dataAccessId;
}
/**
* ISO8601 timespan specifying the minimum number of days the environment's events will be available for query.
*
*/
@Export(name="dataRetentionTime", refs={String.class}, tree="[0]")
private Output dataRetentionTime;
/**
* @return ISO8601 timespan specifying the minimum number of days the environment's events will be available for query.
*
*/
public Output dataRetentionTime() {
return this.dataRetentionTime;
}
/**
* The kind of the environment.
* Expected value is 'Gen1'.
*
*/
@Export(name="kind", refs={String.class}, tree="[0]")
private Output kind;
/**
* @return The kind of the environment.
* Expected value is 'Gen1'.
*
*/
public Output kind() {
return this.kind;
}
/**
* Resource location
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return Resource location
*
*/
public Output location() {
return this.location;
}
/**
* Resource name
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource name
*
*/
public Output name() {
return this.name;
}
/**
* The list of event properties which will be used to partition data in the environment. Currently, only a single partition key property is supported.
*
*/
@Export(name="partitionKeyProperties", refs={List.class,TimeSeriesIdPropertyResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> partitionKeyProperties;
/**
* @return The list of event properties which will be used to partition data in the environment. Currently, only a single partition key property is supported.
*
*/
public Output>> partitionKeyProperties() {
return Codegen.optional(this.partitionKeyProperties);
}
/**
* Provisioning state of the resource.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Provisioning state of the resource.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* The sku determines the type of environment, either Gen1 (S1 or S2) or Gen2 (L1). For Gen1 environments the sku determines the capacity of the environment, the ingress rate, and the billing rate.
*
*/
@Export(name="sku", refs={SkuResponse.class}, tree="[0]")
private Output sku;
/**
* @return The sku determines the type of environment, either Gen1 (S1 or S2) or Gen2 (L1). For Gen1 environments the sku determines the capacity of the environment, the ingress rate, and the billing rate.
*
*/
public Output sku() {
return this.sku;
}
/**
* An object that represents the status of the environment, and its internal state in the Time Series Insights service.
*
*/
@Export(name="status", refs={EnvironmentStatusResponse.class}, tree="[0]")
private Output status;
/**
* @return An object that represents the status of the environment, and its internal state in the Time Series Insights service.
*
*/
public Output status() {
return this.status;
}
/**
* The behavior the Time Series Insights service should take when the environment's capacity has been exceeded. If "PauseIngress" is specified, new events will not be read from the event source. If "PurgeOldData" is specified, new events will continue to be read and old events will be deleted from the environment. The default behavior is PurgeOldData.
*
*/
@Export(name="storageLimitExceededBehavior", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> storageLimitExceededBehavior;
/**
* @return The behavior the Time Series Insights service should take when the environment's capacity has been exceeded. If "PauseIngress" is specified, new events will not be read from the event source. If "PurgeOldData" is specified, new events will continue to be read and old events will be deleted from the environment. The default behavior is PurgeOldData.
*
*/
public Output> storageLimitExceededBehavior() {
return Codegen.optional(this.storageLimitExceededBehavior);
}
/**
* Resource tags
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Resource type
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Gen1Environment(java.lang.String name) {
this(name, Gen1EnvironmentArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Gen1Environment(java.lang.String name, Gen1EnvironmentArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Gen1Environment(java.lang.String name, Gen1EnvironmentArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:timeseriesinsights:Gen1Environment", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Gen1Environment(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:timeseriesinsights:Gen1Environment", name, null, makeResourceOptions(options, id), false);
}
private static Gen1EnvironmentArgs makeArgs(Gen1EnvironmentArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
var builder = args == null ? Gen1EnvironmentArgs.builder() : Gen1EnvironmentArgs.builder(args);
return builder
.kind("Gen1")
.build();
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:timeseriesinsights/v20170228preview:Gen1Environment").build()),
Output.of(Alias.builder().type("azure-native:timeseriesinsights/v20171115:Gen1Environment").build()),
Output.of(Alias.builder().type("azure-native:timeseriesinsights/v20180815preview:Gen1Environment").build()),
Output.of(Alias.builder().type("azure-native:timeseriesinsights/v20200515:Gen1Environment").build()),
Output.of(Alias.builder().type("azure-native:timeseriesinsights/v20210331preview:Gen1Environment").build()),
Output.of(Alias.builder().type("azure-native:timeseriesinsights/v20210630preview:Gen1Environment").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Gen1Environment get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Gen1Environment(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy