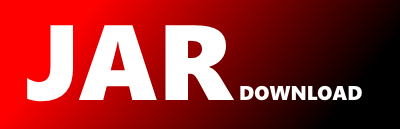
com.pulumi.azurenative.timeseriesinsights.Gen1EnvironmentArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.timeseriesinsights;
import com.pulumi.azurenative.timeseriesinsights.enums.StorageLimitExceededBehavior;
import com.pulumi.azurenative.timeseriesinsights.inputs.SkuArgs;
import com.pulumi.azurenative.timeseriesinsights.inputs.TimeSeriesIdPropertyArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class Gen1EnvironmentArgs extends com.pulumi.resources.ResourceArgs {
public static final Gen1EnvironmentArgs Empty = new Gen1EnvironmentArgs();
/**
* ISO8601 timespan specifying the minimum number of days the environment's events will be available for query.
*
*/
@Import(name="dataRetentionTime", required=true)
private Output dataRetentionTime;
/**
* @return ISO8601 timespan specifying the minimum number of days the environment's events will be available for query.
*
*/
public Output dataRetentionTime() {
return this.dataRetentionTime;
}
/**
* Name of the environment
*
*/
@Import(name="environmentName")
private @Nullable Output environmentName;
/**
* @return Name of the environment
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy