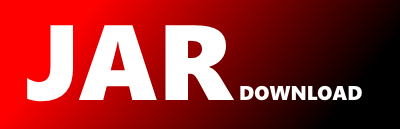
com.pulumi.azurenative.videoanalyzer.inputs.EccTokenKeyArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.videoanalyzer.inputs;
import com.pulumi.azurenative.videoanalyzer.enums.AccessPolicyEccAlgo;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
/**
* Required validation properties for tokens generated with Elliptical Curve algorithm.
*
*/
public final class EccTokenKeyArgs extends com.pulumi.resources.ResourceArgs {
public static final EccTokenKeyArgs Empty = new EccTokenKeyArgs();
/**
* Elliptical curve algorithm to be used: ES256, ES384 or ES512.
*
*/
@Import(name="alg", required=true)
private Output> alg;
/**
* @return Elliptical curve algorithm to be used: ES256, ES384 or ES512.
*
*/
public Output> alg() {
return this.alg;
}
/**
* JWT token key id. Validation keys are looked up based on the key id present on the JWT token header.
*
*/
@Import(name="kid", required=true)
private Output kid;
/**
* @return JWT token key id. Validation keys are looked up based on the key id present on the JWT token header.
*
*/
public Output kid() {
return this.kid;
}
/**
* The discriminator for derived types.
* Expected value is '#Microsoft.VideoAnalyzer.EccTokenKey'.
*
*/
@Import(name="type", required=true)
private Output type;
/**
* @return The discriminator for derived types.
* Expected value is '#Microsoft.VideoAnalyzer.EccTokenKey'.
*
*/
public Output type() {
return this.type;
}
/**
* X coordinate.
*
*/
@Import(name="x", required=true)
private Output x;
/**
* @return X coordinate.
*
*/
public Output x() {
return this.x;
}
/**
* Y coordinate.
*
*/
@Import(name="y", required=true)
private Output y;
/**
* @return Y coordinate.
*
*/
public Output y() {
return this.y;
}
private EccTokenKeyArgs() {}
private EccTokenKeyArgs(EccTokenKeyArgs $) {
this.alg = $.alg;
this.kid = $.kid;
this.type = $.type;
this.x = $.x;
this.y = $.y;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(EccTokenKeyArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private EccTokenKeyArgs $;
public Builder() {
$ = new EccTokenKeyArgs();
}
public Builder(EccTokenKeyArgs defaults) {
$ = new EccTokenKeyArgs(Objects.requireNonNull(defaults));
}
/**
* @param alg Elliptical curve algorithm to be used: ES256, ES384 or ES512.
*
* @return builder
*
*/
public Builder alg(Output> alg) {
$.alg = alg;
return this;
}
/**
* @param alg Elliptical curve algorithm to be used: ES256, ES384 or ES512.
*
* @return builder
*
*/
public Builder alg(Either alg) {
return alg(Output.of(alg));
}
/**
* @param alg Elliptical curve algorithm to be used: ES256, ES384 or ES512.
*
* @return builder
*
*/
public Builder alg(String alg) {
return alg(Either.ofLeft(alg));
}
/**
* @param alg Elliptical curve algorithm to be used: ES256, ES384 or ES512.
*
* @return builder
*
*/
public Builder alg(AccessPolicyEccAlgo alg) {
return alg(Either.ofRight(alg));
}
/**
* @param kid JWT token key id. Validation keys are looked up based on the key id present on the JWT token header.
*
* @return builder
*
*/
public Builder kid(Output kid) {
$.kid = kid;
return this;
}
/**
* @param kid JWT token key id. Validation keys are looked up based on the key id present on the JWT token header.
*
* @return builder
*
*/
public Builder kid(String kid) {
return kid(Output.of(kid));
}
/**
* @param type The discriminator for derived types.
* Expected value is '#Microsoft.VideoAnalyzer.EccTokenKey'.
*
* @return builder
*
*/
public Builder type(Output type) {
$.type = type;
return this;
}
/**
* @param type The discriminator for derived types.
* Expected value is '#Microsoft.VideoAnalyzer.EccTokenKey'.
*
* @return builder
*
*/
public Builder type(String type) {
return type(Output.of(type));
}
/**
* @param x X coordinate.
*
* @return builder
*
*/
public Builder x(Output x) {
$.x = x;
return this;
}
/**
* @param x X coordinate.
*
* @return builder
*
*/
public Builder x(String x) {
return x(Output.of(x));
}
/**
* @param y Y coordinate.
*
* @return builder
*
*/
public Builder y(Output y) {
$.y = y;
return this;
}
/**
* @param y Y coordinate.
*
* @return builder
*
*/
public Builder y(String y) {
return y(Output.of(y));
}
public EccTokenKeyArgs build() {
if ($.alg == null) {
throw new MissingRequiredPropertyException("EccTokenKeyArgs", "alg");
}
if ($.kid == null) {
throw new MissingRequiredPropertyException("EccTokenKeyArgs", "kid");
}
$.type = Codegen.stringProp("type").output().arg($.type).require();
if ($.x == null) {
throw new MissingRequiredPropertyException("EccTokenKeyArgs", "x");
}
if ($.y == null) {
throw new MissingRequiredPropertyException("EccTokenKeyArgs", "y");
}
return $;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy