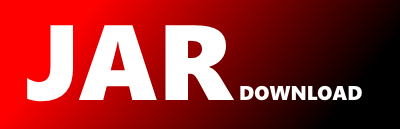
com.pulumi.azurenative.web.StaticSitePrivateEndpointConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.web.StaticSitePrivateEndpointConnectionArgs;
import com.pulumi.azurenative.web.outputs.ArmIdWrapperResponse;
import com.pulumi.azurenative.web.outputs.PrivateLinkConnectionStateResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Remote Private Endpoint Connection ARM resource.
* Azure REST API version: 2022-09-01. Prior API version in Azure Native 1.x: 2020-12-01.
*
* Other available API versions: 2023-01-01, 2023-12-01, 2024-04-01.
*
* ## Example Usage
* ### Approves or rejects a private endpoint connection for a site.
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.web.StaticSitePrivateEndpointConnection;
* import com.pulumi.azurenative.web.StaticSitePrivateEndpointConnectionArgs;
* import com.pulumi.azurenative.web.inputs.PrivateLinkConnectionStateArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var staticSitePrivateEndpointConnection = new StaticSitePrivateEndpointConnection("staticSitePrivateEndpointConnection", StaticSitePrivateEndpointConnectionArgs.builder()
* .name("testSite")
* .privateEndpointConnectionName("connection")
* .privateLinkServiceConnectionState(PrivateLinkConnectionStateArgs.builder()
* .actionsRequired("")
* .description("Approved by admin.")
* .status("Approved")
* .build())
* .resourceGroupName("rg")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:web:StaticSitePrivateEndpointConnection connection /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Web/staticSites/{name}/privateEndpointConnections/{privateEndpointConnectionName}
* ```
*
*/
@ResourceType(type="azure-native:web:StaticSitePrivateEndpointConnection")
public class StaticSitePrivateEndpointConnection extends com.pulumi.resources.CustomResource {
/**
* Private IPAddresses mapped to the remote private endpoint
*
*/
@Export(name="ipAddresses", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> ipAddresses;
/**
* @return Private IPAddresses mapped to the remote private endpoint
*
*/
public Output>> ipAddresses() {
return Codegen.optional(this.ipAddresses);
}
/**
* Kind of resource.
*
*/
@Export(name="kind", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> kind;
/**
* @return Kind of resource.
*
*/
public Output> kind() {
return Codegen.optional(this.kind);
}
/**
* Resource Name.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource Name.
*
*/
public Output name() {
return this.name;
}
/**
* PrivateEndpoint of a remote private endpoint connection
*
*/
@Export(name="privateEndpoint", refs={ArmIdWrapperResponse.class}, tree="[0]")
private Output* @Nullable */ ArmIdWrapperResponse> privateEndpoint;
/**
* @return PrivateEndpoint of a remote private endpoint connection
*
*/
public Output> privateEndpoint() {
return Codegen.optional(this.privateEndpoint);
}
/**
* The state of a private link connection
*
*/
@Export(name="privateLinkServiceConnectionState", refs={PrivateLinkConnectionStateResponse.class}, tree="[0]")
private Output* @Nullable */ PrivateLinkConnectionStateResponse> privateLinkServiceConnectionState;
/**
* @return The state of a private link connection
*
*/
public Output> privateLinkServiceConnectionState() {
return Codegen.optional(this.privateLinkServiceConnectionState);
}
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
public Output provisioningState() {
return this.provisioningState;
}
/**
* Resource type.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type.
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public StaticSitePrivateEndpointConnection(java.lang.String name) {
this(name, StaticSitePrivateEndpointConnectionArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public StaticSitePrivateEndpointConnection(java.lang.String name, StaticSitePrivateEndpointConnectionArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public StaticSitePrivateEndpointConnection(java.lang.String name, StaticSitePrivateEndpointConnectionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:web:StaticSitePrivateEndpointConnection", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private StaticSitePrivateEndpointConnection(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:web:StaticSitePrivateEndpointConnection", name, null, makeResourceOptions(options, id), false);
}
private static StaticSitePrivateEndpointConnectionArgs makeArgs(StaticSitePrivateEndpointConnectionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? StaticSitePrivateEndpointConnectionArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:web/v20201201:StaticSitePrivateEndpointConnection").build()),
Output.of(Alias.builder().type("azure-native:web/v20210101:StaticSitePrivateEndpointConnection").build()),
Output.of(Alias.builder().type("azure-native:web/v20210115:StaticSitePrivateEndpointConnection").build()),
Output.of(Alias.builder().type("azure-native:web/v20210201:StaticSitePrivateEndpointConnection").build()),
Output.of(Alias.builder().type("azure-native:web/v20210301:StaticSitePrivateEndpointConnection").build()),
Output.of(Alias.builder().type("azure-native:web/v20220301:StaticSitePrivateEndpointConnection").build()),
Output.of(Alias.builder().type("azure-native:web/v20220901:StaticSitePrivateEndpointConnection").build()),
Output.of(Alias.builder().type("azure-native:web/v20230101:StaticSitePrivateEndpointConnection").build()),
Output.of(Alias.builder().type("azure-native:web/v20231201:StaticSitePrivateEndpointConnection").build()),
Output.of(Alias.builder().type("azure-native:web/v20240401:StaticSitePrivateEndpointConnection").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static StaticSitePrivateEndpointConnection get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new StaticSitePrivateEndpointConnection(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy