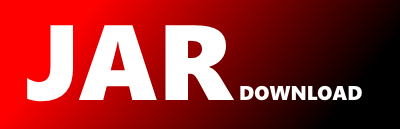
com.pulumi.azurenative.web.WebAppPremierAddOnArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class WebAppPremierAddOnArgs extends com.pulumi.resources.ResourceArgs {
public static final WebAppPremierAddOnArgs Empty = new WebAppPremierAddOnArgs();
/**
* Kind of resource.
*
*/
@Import(name="kind")
private @Nullable Output kind;
/**
* @return Kind of resource.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy