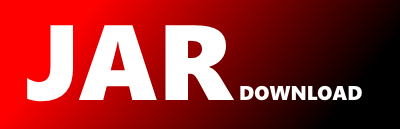
com.pulumi.azurenative.web.inputs.BackupScheduleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web.inputs;
import com.pulumi.azurenative.web.enums.FrequencyUnit;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Description of a backup schedule. Describes how often should be the backup performed and what should be the retention policy.
*
*/
public final class BackupScheduleArgs extends com.pulumi.resources.ResourceArgs {
public static final BackupScheduleArgs Empty = new BackupScheduleArgs();
/**
* How often the backup should be executed (e.g. for weekly backup, this should be set to 7 and FrequencyUnit should be set to Day)
*
*/
@Import(name="frequencyInterval", required=true)
private Output frequencyInterval;
/**
* @return How often the backup should be executed (e.g. for weekly backup, this should be set to 7 and FrequencyUnit should be set to Day)
*
*/
public Output frequencyInterval() {
return this.frequencyInterval;
}
/**
* The unit of time for how often the backup should be executed (e.g. for weekly backup, this should be set to Day and FrequencyInterval should be set to 7)
*
*/
@Import(name="frequencyUnit", required=true)
private Output frequencyUnit;
/**
* @return The unit of time for how often the backup should be executed (e.g. for weekly backup, this should be set to Day and FrequencyInterval should be set to 7)
*
*/
public Output frequencyUnit() {
return this.frequencyUnit;
}
/**
* True if the retention policy should always keep at least one backup in the storage account, regardless how old it is; false otherwise.
*
*/
@Import(name="keepAtLeastOneBackup", required=true)
private Output keepAtLeastOneBackup;
/**
* @return True if the retention policy should always keep at least one backup in the storage account, regardless how old it is; false otherwise.
*
*/
public Output keepAtLeastOneBackup() {
return this.keepAtLeastOneBackup;
}
/**
* After how many days backups should be deleted.
*
*/
@Import(name="retentionPeriodInDays", required=true)
private Output retentionPeriodInDays;
/**
* @return After how many days backups should be deleted.
*
*/
public Output retentionPeriodInDays() {
return this.retentionPeriodInDays;
}
/**
* When the schedule should start working.
*
*/
@Import(name="startTime")
private @Nullable Output startTime;
/**
* @return When the schedule should start working.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy