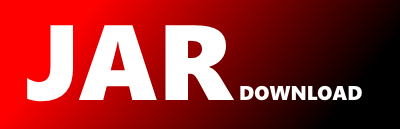
com.pulumi.azurenative.web.outputs.BackupItemResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web.outputs;
import com.pulumi.azurenative.web.outputs.DatabaseBackupSettingResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class BackupItemResponse {
/**
* @return Id of the backup.
*
*/
private Integer backupId;
/**
* @return Name of the blob which contains data for this backup.
*
*/
private String blobName;
/**
* @return Unique correlation identifier. Please use this along with the timestamp while communicating with Azure support.
*
*/
private String correlationId;
/**
* @return Timestamp of the backup creation.
*
*/
private String created;
/**
* @return List of databases included in the backup.
*
*/
private List databases;
/**
* @return Timestamp when this backup finished.
*
*/
private String finishedTimeStamp;
/**
* @return Resource Id.
*
*/
private String id;
/**
* @return Kind of resource.
*
*/
private @Nullable String kind;
/**
* @return Timestamp of a last restore operation which used this backup.
*
*/
private String lastRestoreTimeStamp;
/**
* @return Details regarding this backup. Might contain an error message.
*
*/
private String log;
/**
* @return Resource Name.
*
*/
private String name;
/**
* @return True if this backup has been created due to a schedule being triggered.
*
*/
private Boolean scheduled;
/**
* @return Size of the backup in bytes.
*
*/
private Double sizeInBytes;
/**
* @return Backup status.
*
*/
private String status;
/**
* @return SAS URL for the storage account container which contains this backup.
*
*/
private String storageAccountUrl;
/**
* @return Resource type.
*
*/
private String type;
/**
* @return Size of the original web app which has been backed up.
*
*/
private Double websiteSizeInBytes;
private BackupItemResponse() {}
/**
* @return Id of the backup.
*
*/
public Integer backupId() {
return this.backupId;
}
/**
* @return Name of the blob which contains data for this backup.
*
*/
public String blobName() {
return this.blobName;
}
/**
* @return Unique correlation identifier. Please use this along with the timestamp while communicating with Azure support.
*
*/
public String correlationId() {
return this.correlationId;
}
/**
* @return Timestamp of the backup creation.
*
*/
public String created() {
return this.created;
}
/**
* @return List of databases included in the backup.
*
*/
public List databases() {
return this.databases;
}
/**
* @return Timestamp when this backup finished.
*
*/
public String finishedTimeStamp() {
return this.finishedTimeStamp;
}
/**
* @return Resource Id.
*
*/
public String id() {
return this.id;
}
/**
* @return Kind of resource.
*
*/
public Optional kind() {
return Optional.ofNullable(this.kind);
}
/**
* @return Timestamp of a last restore operation which used this backup.
*
*/
public String lastRestoreTimeStamp() {
return this.lastRestoreTimeStamp;
}
/**
* @return Details regarding this backup. Might contain an error message.
*
*/
public String log() {
return this.log;
}
/**
* @return Resource Name.
*
*/
public String name() {
return this.name;
}
/**
* @return True if this backup has been created due to a schedule being triggered.
*
*/
public Boolean scheduled() {
return this.scheduled;
}
/**
* @return Size of the backup in bytes.
*
*/
public Double sizeInBytes() {
return this.sizeInBytes;
}
/**
* @return Backup status.
*
*/
public String status() {
return this.status;
}
/**
* @return SAS URL for the storage account container which contains this backup.
*
*/
public String storageAccountUrl() {
return this.storageAccountUrl;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
/**
* @return Size of the original web app which has been backed up.
*
*/
public Double websiteSizeInBytes() {
return this.websiteSizeInBytes;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(BackupItemResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Integer backupId;
private String blobName;
private String correlationId;
private String created;
private List databases;
private String finishedTimeStamp;
private String id;
private @Nullable String kind;
private String lastRestoreTimeStamp;
private String log;
private String name;
private Boolean scheduled;
private Double sizeInBytes;
private String status;
private String storageAccountUrl;
private String type;
private Double websiteSizeInBytes;
public Builder() {}
public Builder(BackupItemResponse defaults) {
Objects.requireNonNull(defaults);
this.backupId = defaults.backupId;
this.blobName = defaults.blobName;
this.correlationId = defaults.correlationId;
this.created = defaults.created;
this.databases = defaults.databases;
this.finishedTimeStamp = defaults.finishedTimeStamp;
this.id = defaults.id;
this.kind = defaults.kind;
this.lastRestoreTimeStamp = defaults.lastRestoreTimeStamp;
this.log = defaults.log;
this.name = defaults.name;
this.scheduled = defaults.scheduled;
this.sizeInBytes = defaults.sizeInBytes;
this.status = defaults.status;
this.storageAccountUrl = defaults.storageAccountUrl;
this.type = defaults.type;
this.websiteSizeInBytes = defaults.websiteSizeInBytes;
}
@CustomType.Setter
public Builder backupId(Integer backupId) {
if (backupId == null) {
throw new MissingRequiredPropertyException("BackupItemResponse", "backupId");
}
this.backupId = backupId;
return this;
}
@CustomType.Setter
public Builder blobName(String blobName) {
if (blobName == null) {
throw new MissingRequiredPropertyException("BackupItemResponse", "blobName");
}
this.blobName = blobName;
return this;
}
@CustomType.Setter
public Builder correlationId(String correlationId) {
if (correlationId == null) {
throw new MissingRequiredPropertyException("BackupItemResponse", "correlationId");
}
this.correlationId = correlationId;
return this;
}
@CustomType.Setter
public Builder created(String created) {
if (created == null) {
throw new MissingRequiredPropertyException("BackupItemResponse", "created");
}
this.created = created;
return this;
}
@CustomType.Setter
public Builder databases(List databases) {
if (databases == null) {
throw new MissingRequiredPropertyException("BackupItemResponse", "databases");
}
this.databases = databases;
return this;
}
public Builder databases(DatabaseBackupSettingResponse... databases) {
return databases(List.of(databases));
}
@CustomType.Setter
public Builder finishedTimeStamp(String finishedTimeStamp) {
if (finishedTimeStamp == null) {
throw new MissingRequiredPropertyException("BackupItemResponse", "finishedTimeStamp");
}
this.finishedTimeStamp = finishedTimeStamp;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("BackupItemResponse", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kind(@Nullable String kind) {
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder lastRestoreTimeStamp(String lastRestoreTimeStamp) {
if (lastRestoreTimeStamp == null) {
throw new MissingRequiredPropertyException("BackupItemResponse", "lastRestoreTimeStamp");
}
this.lastRestoreTimeStamp = lastRestoreTimeStamp;
return this;
}
@CustomType.Setter
public Builder log(String log) {
if (log == null) {
throw new MissingRequiredPropertyException("BackupItemResponse", "log");
}
this.log = log;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("BackupItemResponse", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder scheduled(Boolean scheduled) {
if (scheduled == null) {
throw new MissingRequiredPropertyException("BackupItemResponse", "scheduled");
}
this.scheduled = scheduled;
return this;
}
@CustomType.Setter
public Builder sizeInBytes(Double sizeInBytes) {
if (sizeInBytes == null) {
throw new MissingRequiredPropertyException("BackupItemResponse", "sizeInBytes");
}
this.sizeInBytes = sizeInBytes;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("BackupItemResponse", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder storageAccountUrl(String storageAccountUrl) {
if (storageAccountUrl == null) {
throw new MissingRequiredPropertyException("BackupItemResponse", "storageAccountUrl");
}
this.storageAccountUrl = storageAccountUrl;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("BackupItemResponse", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder websiteSizeInBytes(Double websiteSizeInBytes) {
if (websiteSizeInBytes == null) {
throw new MissingRequiredPropertyException("BackupItemResponse", "websiteSizeInBytes");
}
this.websiteSizeInBytes = websiteSizeInBytes;
return this;
}
public BackupItemResponse build() {
final var _resultValue = new BackupItemResponse();
_resultValue.backupId = backupId;
_resultValue.blobName = blobName;
_resultValue.correlationId = correlationId;
_resultValue.created = created;
_resultValue.databases = databases;
_resultValue.finishedTimeStamp = finishedTimeStamp;
_resultValue.id = id;
_resultValue.kind = kind;
_resultValue.lastRestoreTimeStamp = lastRestoreTimeStamp;
_resultValue.log = log;
_resultValue.name = name;
_resultValue.scheduled = scheduled;
_resultValue.sizeInBytes = sizeInBytes;
_resultValue.status = status;
_resultValue.storageAccountUrl = storageAccountUrl;
_resultValue.type = type;
_resultValue.websiteSizeInBytes = websiteSizeInBytes;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy