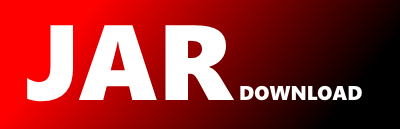
com.pulumi.azurenative.aad.inputs.LdapsSettingsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.aad.inputs;
import com.pulumi.azurenative.aad.enums.ExternalAccess;
import com.pulumi.azurenative.aad.enums.Ldaps;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Secure LDAP Settings
*
*/
public final class LdapsSettingsArgs extends com.pulumi.resources.ResourceArgs {
public static final LdapsSettingsArgs Empty = new LdapsSettingsArgs();
/**
* A flag to determine whether or not Secure LDAP access over the internet is enabled or disabled.
*
*/
@Import(name="externalAccess")
private @Nullable Output> externalAccess;
/**
* @return A flag to determine whether or not Secure LDAP access over the internet is enabled or disabled.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy