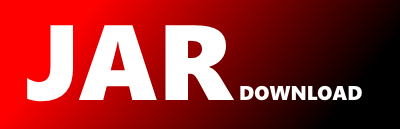
com.pulumi.azurenative.aad.outputs.ReplicaSetResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.aad.outputs;
import com.pulumi.azurenative.aad.outputs.HealthAlertResponse;
import com.pulumi.azurenative.aad.outputs.HealthMonitorResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ReplicaSetResponse {
/**
* @return List of Domain Controller IP Address
*
*/
private List domainControllerIpAddress;
/**
* @return External access ip address.
*
*/
private String externalAccessIpAddress;
/**
* @return List of Domain Health Alerts
*
*/
private List healthAlerts;
/**
* @return Last domain evaluation run DateTime
*
*/
private String healthLastEvaluated;
/**
* @return List of Domain Health Monitors
*
*/
private List healthMonitors;
/**
* @return Virtual network location
*
*/
private @Nullable String location;
/**
* @return ReplicaSet Id
*
*/
private String replicaSetId;
/**
* @return Status of Domain Service instance
*
*/
private String serviceStatus;
/**
* @return The name of the virtual network that Domain Services will be deployed on. The id of the subnet that Domain Services will be deployed on. /virtualNetwork/vnetName/subnets/subnetName.
*
*/
private @Nullable String subnetId;
/**
* @return Virtual network site id
*
*/
private String vnetSiteId;
private ReplicaSetResponse() {}
/**
* @return List of Domain Controller IP Address
*
*/
public List domainControllerIpAddress() {
return this.domainControllerIpAddress;
}
/**
* @return External access ip address.
*
*/
public String externalAccessIpAddress() {
return this.externalAccessIpAddress;
}
/**
* @return List of Domain Health Alerts
*
*/
public List healthAlerts() {
return this.healthAlerts;
}
/**
* @return Last domain evaluation run DateTime
*
*/
public String healthLastEvaluated() {
return this.healthLastEvaluated;
}
/**
* @return List of Domain Health Monitors
*
*/
public List healthMonitors() {
return this.healthMonitors;
}
/**
* @return Virtual network location
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return ReplicaSet Id
*
*/
public String replicaSetId() {
return this.replicaSetId;
}
/**
* @return Status of Domain Service instance
*
*/
public String serviceStatus() {
return this.serviceStatus;
}
/**
* @return The name of the virtual network that Domain Services will be deployed on. The id of the subnet that Domain Services will be deployed on. /virtualNetwork/vnetName/subnets/subnetName.
*
*/
public Optional subnetId() {
return Optional.ofNullable(this.subnetId);
}
/**
* @return Virtual network site id
*
*/
public String vnetSiteId() {
return this.vnetSiteId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ReplicaSetResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List domainControllerIpAddress;
private String externalAccessIpAddress;
private List healthAlerts;
private String healthLastEvaluated;
private List healthMonitors;
private @Nullable String location;
private String replicaSetId;
private String serviceStatus;
private @Nullable String subnetId;
private String vnetSiteId;
public Builder() {}
public Builder(ReplicaSetResponse defaults) {
Objects.requireNonNull(defaults);
this.domainControllerIpAddress = defaults.domainControllerIpAddress;
this.externalAccessIpAddress = defaults.externalAccessIpAddress;
this.healthAlerts = defaults.healthAlerts;
this.healthLastEvaluated = defaults.healthLastEvaluated;
this.healthMonitors = defaults.healthMonitors;
this.location = defaults.location;
this.replicaSetId = defaults.replicaSetId;
this.serviceStatus = defaults.serviceStatus;
this.subnetId = defaults.subnetId;
this.vnetSiteId = defaults.vnetSiteId;
}
@CustomType.Setter
public Builder domainControllerIpAddress(List domainControllerIpAddress) {
if (domainControllerIpAddress == null) {
throw new MissingRequiredPropertyException("ReplicaSetResponse", "domainControllerIpAddress");
}
this.domainControllerIpAddress = domainControllerIpAddress;
return this;
}
public Builder domainControllerIpAddress(String... domainControllerIpAddress) {
return domainControllerIpAddress(List.of(domainControllerIpAddress));
}
@CustomType.Setter
public Builder externalAccessIpAddress(String externalAccessIpAddress) {
if (externalAccessIpAddress == null) {
throw new MissingRequiredPropertyException("ReplicaSetResponse", "externalAccessIpAddress");
}
this.externalAccessIpAddress = externalAccessIpAddress;
return this;
}
@CustomType.Setter
public Builder healthAlerts(List healthAlerts) {
if (healthAlerts == null) {
throw new MissingRequiredPropertyException("ReplicaSetResponse", "healthAlerts");
}
this.healthAlerts = healthAlerts;
return this;
}
public Builder healthAlerts(HealthAlertResponse... healthAlerts) {
return healthAlerts(List.of(healthAlerts));
}
@CustomType.Setter
public Builder healthLastEvaluated(String healthLastEvaluated) {
if (healthLastEvaluated == null) {
throw new MissingRequiredPropertyException("ReplicaSetResponse", "healthLastEvaluated");
}
this.healthLastEvaluated = healthLastEvaluated;
return this;
}
@CustomType.Setter
public Builder healthMonitors(List healthMonitors) {
if (healthMonitors == null) {
throw new MissingRequiredPropertyException("ReplicaSetResponse", "healthMonitors");
}
this.healthMonitors = healthMonitors;
return this;
}
public Builder healthMonitors(HealthMonitorResponse... healthMonitors) {
return healthMonitors(List.of(healthMonitors));
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder replicaSetId(String replicaSetId) {
if (replicaSetId == null) {
throw new MissingRequiredPropertyException("ReplicaSetResponse", "replicaSetId");
}
this.replicaSetId = replicaSetId;
return this;
}
@CustomType.Setter
public Builder serviceStatus(String serviceStatus) {
if (serviceStatus == null) {
throw new MissingRequiredPropertyException("ReplicaSetResponse", "serviceStatus");
}
this.serviceStatus = serviceStatus;
return this;
}
@CustomType.Setter
public Builder subnetId(@Nullable String subnetId) {
this.subnetId = subnetId;
return this;
}
@CustomType.Setter
public Builder vnetSiteId(String vnetSiteId) {
if (vnetSiteId == null) {
throw new MissingRequiredPropertyException("ReplicaSetResponse", "vnetSiteId");
}
this.vnetSiteId = vnetSiteId;
return this;
}
public ReplicaSetResponse build() {
final var _resultValue = new ReplicaSetResponse();
_resultValue.domainControllerIpAddress = domainControllerIpAddress;
_resultValue.externalAccessIpAddress = externalAccessIpAddress;
_resultValue.healthAlerts = healthAlerts;
_resultValue.healthLastEvaluated = healthLastEvaluated;
_resultValue.healthMonitors = healthMonitors;
_resultValue.location = location;
_resultValue.replicaSetId = replicaSetId;
_resultValue.serviceStatus = serviceStatus;
_resultValue.subnetId = subnetId;
_resultValue.vnetSiteId = vnetSiteId;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy