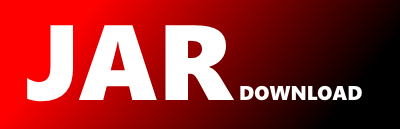
com.pulumi.azurenative.apimanagement.ProductApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.apimanagement;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.apimanagement.ProductApiArgs;
import com.pulumi.azurenative.apimanagement.outputs.ApiContactInformationResponse;
import com.pulumi.azurenative.apimanagement.outputs.ApiLicenseInformationResponse;
import com.pulumi.azurenative.apimanagement.outputs.ApiVersionSetContractDetailsResponse;
import com.pulumi.azurenative.apimanagement.outputs.AuthenticationSettingsContractResponse;
import com.pulumi.azurenative.apimanagement.outputs.SubscriptionKeyParameterNamesContractResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* API details.
* Azure REST API version: 2022-08-01. Prior API version in Azure Native 1.x: 2020-12-01.
*
* Other available API versions: 2017-03-01, 2018-06-01-preview, 2022-09-01-preview, 2023-03-01-preview, 2023-05-01-preview, 2023-09-01-preview, 2024-05-01.
*
* ## Example Usage
* ### ApiManagementCreateProductApi
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.apimanagement.ProductApi;
* import com.pulumi.azurenative.apimanagement.ProductApiArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var productApi = new ProductApi("productApi", ProductApiArgs.builder()
* .apiId("echo-api")
* .productId("testproduct")
* .resourceGroupName("rg1")
* .serviceName("apimService1")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:apimanagement:ProductApi 5931a75ae4bbd512a88c680b /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ApiManagement/service/{serviceName}/products/{productId}/apis/{apiId}
* ```
*
*/
@ResourceType(type="azure-native:apimanagement:ProductApi")
public class ProductApi extends com.pulumi.resources.CustomResource {
/**
* Describes the revision of the API. If no value is provided, default revision 1 is created
*
*/
@Export(name="apiRevision", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> apiRevision;
/**
* @return Describes the revision of the API. If no value is provided, default revision 1 is created
*
*/
public Output> apiRevision() {
return Codegen.optional(this.apiRevision);
}
/**
* Description of the API Revision.
*
*/
@Export(name="apiRevisionDescription", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> apiRevisionDescription;
/**
* @return Description of the API Revision.
*
*/
public Output> apiRevisionDescription() {
return Codegen.optional(this.apiRevisionDescription);
}
/**
* Type of API.
*
*/
@Export(name="apiType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> apiType;
/**
* @return Type of API.
*
*/
public Output> apiType() {
return Codegen.optional(this.apiType);
}
/**
* Indicates the version identifier of the API if the API is versioned
*
*/
@Export(name="apiVersion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> apiVersion;
/**
* @return Indicates the version identifier of the API if the API is versioned
*
*/
public Output> apiVersion() {
return Codegen.optional(this.apiVersion);
}
/**
* Description of the API Version.
*
*/
@Export(name="apiVersionDescription", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> apiVersionDescription;
/**
* @return Description of the API Version.
*
*/
public Output> apiVersionDescription() {
return Codegen.optional(this.apiVersionDescription);
}
/**
* Version set details
*
*/
@Export(name="apiVersionSet", refs={ApiVersionSetContractDetailsResponse.class}, tree="[0]")
private Output* @Nullable */ ApiVersionSetContractDetailsResponse> apiVersionSet;
/**
* @return Version set details
*
*/
public Output> apiVersionSet() {
return Codegen.optional(this.apiVersionSet);
}
/**
* A resource identifier for the related ApiVersionSet.
*
*/
@Export(name="apiVersionSetId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> apiVersionSetId;
/**
* @return A resource identifier for the related ApiVersionSet.
*
*/
public Output> apiVersionSetId() {
return Codegen.optional(this.apiVersionSetId);
}
/**
* Collection of authentication settings included into this API.
*
*/
@Export(name="authenticationSettings", refs={AuthenticationSettingsContractResponse.class}, tree="[0]")
private Output* @Nullable */ AuthenticationSettingsContractResponse> authenticationSettings;
/**
* @return Collection of authentication settings included into this API.
*
*/
public Output> authenticationSettings() {
return Codegen.optional(this.authenticationSettings);
}
/**
* Contact information for the API.
*
*/
@Export(name="contact", refs={ApiContactInformationResponse.class}, tree="[0]")
private Output* @Nullable */ ApiContactInformationResponse> contact;
/**
* @return Contact information for the API.
*
*/
public Output> contact() {
return Codegen.optional(this.contact);
}
/**
* Description of the API. May include HTML formatting tags.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Description of the API. May include HTML formatting tags.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* API name. Must be 1 to 300 characters long.
*
*/
@Export(name="displayName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> displayName;
/**
* @return API name. Must be 1 to 300 characters long.
*
*/
public Output> displayName() {
return Codegen.optional(this.displayName);
}
/**
* Indicates if API revision is current api revision.
*
*/
@Export(name="isCurrent", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> isCurrent;
/**
* @return Indicates if API revision is current api revision.
*
*/
public Output> isCurrent() {
return Codegen.optional(this.isCurrent);
}
/**
* Indicates if API revision is accessible via the gateway.
*
*/
@Export(name="isOnline", refs={Boolean.class}, tree="[0]")
private Output isOnline;
/**
* @return Indicates if API revision is accessible via the gateway.
*
*/
public Output isOnline() {
return this.isOnline;
}
/**
* License information for the API.
*
*/
@Export(name="license", refs={ApiLicenseInformationResponse.class}, tree="[0]")
private Output* @Nullable */ ApiLicenseInformationResponse> license;
/**
* @return License information for the API.
*
*/
public Output> license() {
return Codegen.optional(this.license);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* Relative URL uniquely identifying this API and all of its resource paths within the API Management service instance. It is appended to the API endpoint base URL specified during the service instance creation to form a public URL for this API.
*
*/
@Export(name="path", refs={String.class}, tree="[0]")
private Output path;
/**
* @return Relative URL uniquely identifying this API and all of its resource paths within the API Management service instance. It is appended to the API endpoint base URL specified during the service instance creation to form a public URL for this API.
*
*/
public Output path() {
return this.path;
}
/**
* Describes on which protocols the operations in this API can be invoked.
*
*/
@Export(name="protocols", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> protocols;
/**
* @return Describes on which protocols the operations in this API can be invoked.
*
*/
public Output>> protocols() {
return Codegen.optional(this.protocols);
}
/**
* Absolute URL of the backend service implementing this API. Cannot be more than 2000 characters long.
*
*/
@Export(name="serviceUrl", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> serviceUrl;
/**
* @return Absolute URL of the backend service implementing this API. Cannot be more than 2000 characters long.
*
*/
public Output> serviceUrl() {
return Codegen.optional(this.serviceUrl);
}
/**
* API identifier of the source API.
*
*/
@Export(name="sourceApiId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> sourceApiId;
/**
* @return API identifier of the source API.
*
*/
public Output> sourceApiId() {
return Codegen.optional(this.sourceApiId);
}
/**
* Protocols over which API is made available.
*
*/
@Export(name="subscriptionKeyParameterNames", refs={SubscriptionKeyParameterNamesContractResponse.class}, tree="[0]")
private Output* @Nullable */ SubscriptionKeyParameterNamesContractResponse> subscriptionKeyParameterNames;
/**
* @return Protocols over which API is made available.
*
*/
public Output> subscriptionKeyParameterNames() {
return Codegen.optional(this.subscriptionKeyParameterNames);
}
/**
* Specifies whether an API or Product subscription is required for accessing the API.
*
*/
@Export(name="subscriptionRequired", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> subscriptionRequired;
/**
* @return Specifies whether an API or Product subscription is required for accessing the API.
*
*/
public Output> subscriptionRequired() {
return Codegen.optional(this.subscriptionRequired);
}
/**
* A URL to the Terms of Service for the API. MUST be in the format of a URL.
*
*/
@Export(name="termsOfServiceUrl", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> termsOfServiceUrl;
/**
* @return A URL to the Terms of Service for the API. MUST be in the format of a URL.
*
*/
public Output> termsOfServiceUrl() {
return Codegen.optional(this.termsOfServiceUrl);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ProductApi(java.lang.String name) {
this(name, ProductApiArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ProductApi(java.lang.String name, ProductApiArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ProductApi(java.lang.String name, ProductApiArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:apimanagement:ProductApi", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private ProductApi(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:apimanagement:ProductApi", name, null, makeResourceOptions(options, id), false);
}
private static ProductApiArgs makeArgs(ProductApiArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ProductApiArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:apimanagement/v20170301:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20180101:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20180601preview:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20190101:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20191201:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20191201preview:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20200601preview:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20201201:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20210101preview:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20210401preview:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20210801:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20211201preview:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20220401preview:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20220801:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20220901preview:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20230301preview:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20230501preview:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20230901preview:ProductApi").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20240501:ProductApi").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ProductApi get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ProductApi(name, id, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy