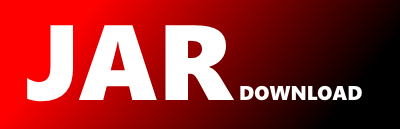
com.pulumi.azurenative.app.outputs.GetDaprComponentResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.app.outputs;
import com.pulumi.azurenative.app.outputs.DaprMetadataResponse;
import com.pulumi.azurenative.app.outputs.SecretResponse;
import com.pulumi.azurenative.app.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetDaprComponentResult {
/**
* @return Component type
*
*/
private @Nullable String componentType;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return Boolean describing if the component errors are ignores
*
*/
private @Nullable Boolean ignoreErrors;
/**
* @return Initialization timeout
*
*/
private @Nullable String initTimeout;
/**
* @return Component metadata
*
*/
private @Nullable List metadata;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Names of container apps that can use this Dapr component
*
*/
private @Nullable List scopes;
/**
* @return Name of a Dapr component to retrieve component secrets from
*
*/
private @Nullable String secretStoreComponent;
/**
* @return Collection of secrets used by a Dapr component
*
*/
private @Nullable List secrets;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return Component version
*
*/
private @Nullable String version;
private GetDaprComponentResult() {}
/**
* @return Component type
*
*/
public Optional componentType() {
return Optional.ofNullable(this.componentType);
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return Boolean describing if the component errors are ignores
*
*/
public Optional ignoreErrors() {
return Optional.ofNullable(this.ignoreErrors);
}
/**
* @return Initialization timeout
*
*/
public Optional initTimeout() {
return Optional.ofNullable(this.initTimeout);
}
/**
* @return Component metadata
*
*/
public List metadata() {
return this.metadata == null ? List.of() : this.metadata;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Names of container apps that can use this Dapr component
*
*/
public List scopes() {
return this.scopes == null ? List.of() : this.scopes;
}
/**
* @return Name of a Dapr component to retrieve component secrets from
*
*/
public Optional secretStoreComponent() {
return Optional.ofNullable(this.secretStoreComponent);
}
/**
* @return Collection of secrets used by a Dapr component
*
*/
public List secrets() {
return this.secrets == null ? List.of() : this.secrets;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return Component version
*
*/
public Optional version() {
return Optional.ofNullable(this.version);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDaprComponentResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String componentType;
private String id;
private @Nullable Boolean ignoreErrors;
private @Nullable String initTimeout;
private @Nullable List metadata;
private String name;
private @Nullable List scopes;
private @Nullable String secretStoreComponent;
private @Nullable List secrets;
private SystemDataResponse systemData;
private String type;
private @Nullable String version;
public Builder() {}
public Builder(GetDaprComponentResult defaults) {
Objects.requireNonNull(defaults);
this.componentType = defaults.componentType;
this.id = defaults.id;
this.ignoreErrors = defaults.ignoreErrors;
this.initTimeout = defaults.initTimeout;
this.metadata = defaults.metadata;
this.name = defaults.name;
this.scopes = defaults.scopes;
this.secretStoreComponent = defaults.secretStoreComponent;
this.secrets = defaults.secrets;
this.systemData = defaults.systemData;
this.type = defaults.type;
this.version = defaults.version;
}
@CustomType.Setter
public Builder componentType(@Nullable String componentType) {
this.componentType = componentType;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetDaprComponentResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder ignoreErrors(@Nullable Boolean ignoreErrors) {
this.ignoreErrors = ignoreErrors;
return this;
}
@CustomType.Setter
public Builder initTimeout(@Nullable String initTimeout) {
this.initTimeout = initTimeout;
return this;
}
@CustomType.Setter
public Builder metadata(@Nullable List metadata) {
this.metadata = metadata;
return this;
}
public Builder metadata(DaprMetadataResponse... metadata) {
return metadata(List.of(metadata));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetDaprComponentResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder scopes(@Nullable List scopes) {
this.scopes = scopes;
return this;
}
public Builder scopes(String... scopes) {
return scopes(List.of(scopes));
}
@CustomType.Setter
public Builder secretStoreComponent(@Nullable String secretStoreComponent) {
this.secretStoreComponent = secretStoreComponent;
return this;
}
@CustomType.Setter
public Builder secrets(@Nullable List secrets) {
this.secrets = secrets;
return this;
}
public Builder secrets(SecretResponse... secrets) {
return secrets(List.of(secrets));
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetDaprComponentResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetDaprComponentResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder version(@Nullable String version) {
this.version = version;
return this;
}
public GetDaprComponentResult build() {
final var _resultValue = new GetDaprComponentResult();
_resultValue.componentType = componentType;
_resultValue.id = id;
_resultValue.ignoreErrors = ignoreErrors;
_resultValue.initTimeout = initTimeout;
_resultValue.metadata = metadata;
_resultValue.name = name;
_resultValue.scopes = scopes;
_resultValue.secretStoreComponent = secretStoreComponent;
_resultValue.secrets = secrets;
_resultValue.systemData = systemData;
_resultValue.type = type;
_resultValue.version = version;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy