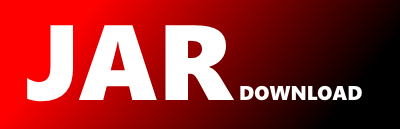
com.pulumi.azurenative.appplatform.inputs.CustomContainerArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.appplatform.inputs;
import com.pulumi.azurenative.appplatform.inputs.ImageRegistryCredentialArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Custom container payload
*
*/
public final class CustomContainerArgs extends com.pulumi.resources.ResourceArgs {
public static final CustomContainerArgs Empty = new CustomContainerArgs();
/**
* Arguments to the entrypoint. The docker image's CMD is used if this is not provided.
*
*/
@Import(name="args")
private @Nullable Output> args;
/**
* @return Arguments to the entrypoint. The docker image's CMD is used if this is not provided.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy