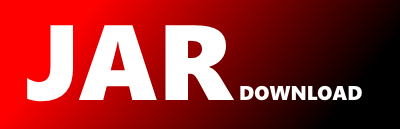
com.pulumi.azurenative.awsconnector.inputs.AwsIamPasswordPolicyPropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Definition of awsIamPasswordPolicy
*
*/
public final class AwsIamPasswordPolicyPropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final AwsIamPasswordPolicyPropertiesArgs Empty = new AwsIamPasswordPolicyPropertiesArgs();
/**
* <p>Specifies whether IAM users are allowed to change their own password. Gives IAM users permissions to <code>iam:ChangePassword</code> for only their user and to the <code>iam:GetAccountPasswordPolicy</code> action. This option does not attach a permissions policy to each user, rather the permissions are applied at the account-level for all users by IAM.</p>
*
*/
@Import(name="allowUsersToChangePassword")
private @Nullable Output allowUsersToChangePassword;
/**
* @return <p>Specifies whether IAM users are allowed to change their own password. Gives IAM users permissions to <code>iam:ChangePassword</code> for only their user and to the <code>iam:GetAccountPasswordPolicy</code> action. This option does not attach a permissions policy to each user, rather the permissions are applied at the account-level for all users by IAM.</p>
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy