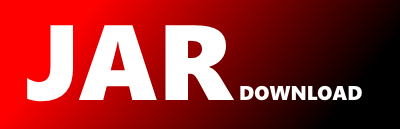
com.pulumi.azurenative.awsconnector.inputs.AwsNetworkFirewallFirewallPropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.inputs;
import com.pulumi.azurenative.awsconnector.inputs.SubnetMappingArgs;
import com.pulumi.azurenative.awsconnector.inputs.TagArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Definition of awsNetworkFirewallFirewall
*
*/
public final class AwsNetworkFirewallFirewallPropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final AwsNetworkFirewallFirewallPropertiesArgs Empty = new AwsNetworkFirewallFirewallPropertiesArgs();
/**
* Property deleteProtection
*
*/
@Import(name="deleteProtection")
private @Nullable Output deleteProtection;
/**
* @return Property deleteProtection
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy