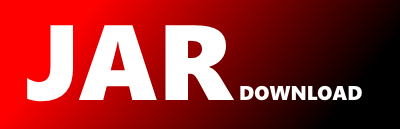
com.pulumi.azurenative.awsconnector.outputs.CodeResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class CodeResponse {
/**
* @return URI of a [container image](https://docs.aws.amazon.com/lambda/latest/dg/lambda-images.html) in the Amazon ECR registry.
*
*/
private @Nullable String imageUri;
/**
* @return An Amazon S3 bucket in the same AWS-Region as your function. The bucket can be in a different AWS-account.
*
*/
private @Nullable String s3Bucket;
/**
* @return The Amazon S3 key of the deployment package.
*
*/
private @Nullable String s3Key;
/**
* @return For versioned objects, the version of the deployment package object to use.
*
*/
private @Nullable String s3ObjectVersion;
/**
* @return (Node.js and Python) The source code of your Lambda function. If you include your function source inline with this parameter, CFN places it in a file named ``index`` and zips it to create a [deployment package](https://docs.aws.amazon.com/lambda/latest/dg/gettingstarted-package.html). This zip file cannot exceed 4MB. For the ``Handler`` property, the first part of the handler identifier must be ``index``. For example, ``index.handler``. For JSON, you must escape quotes and special characters such as newline (``\n``) with a backslash. If you specify a function that interacts with an AWS CloudFormation custom resource, you don't have to write your own functions to send responses to the custom resource that invoked the function. AWS CloudFormation provides a response module ([cfn-response](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/cfn-lambda-function-code-cfnresponsemodule.html)) that simplifies sending responses. See [Using Lambda with CloudFormation](https://docs.aws.amazon.com/lambda/latest/dg/services-cloudformation.html) for details.
*
*/
private @Nullable String zipFile;
private CodeResponse() {}
/**
* @return URI of a [container image](https://docs.aws.amazon.com/lambda/latest/dg/lambda-images.html) in the Amazon ECR registry.
*
*/
public Optional imageUri() {
return Optional.ofNullable(this.imageUri);
}
/**
* @return An Amazon S3 bucket in the same AWS-Region as your function. The bucket can be in a different AWS-account.
*
*/
public Optional s3Bucket() {
return Optional.ofNullable(this.s3Bucket);
}
/**
* @return The Amazon S3 key of the deployment package.
*
*/
public Optional s3Key() {
return Optional.ofNullable(this.s3Key);
}
/**
* @return For versioned objects, the version of the deployment package object to use.
*
*/
public Optional s3ObjectVersion() {
return Optional.ofNullable(this.s3ObjectVersion);
}
/**
* @return (Node.js and Python) The source code of your Lambda function. If you include your function source inline with this parameter, CFN places it in a file named ``index`` and zips it to create a [deployment package](https://docs.aws.amazon.com/lambda/latest/dg/gettingstarted-package.html). This zip file cannot exceed 4MB. For the ``Handler`` property, the first part of the handler identifier must be ``index``. For example, ``index.handler``. For JSON, you must escape quotes and special characters such as newline (``\n``) with a backslash. If you specify a function that interacts with an AWS CloudFormation custom resource, you don't have to write your own functions to send responses to the custom resource that invoked the function. AWS CloudFormation provides a response module ([cfn-response](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/cfn-lambda-function-code-cfnresponsemodule.html)) that simplifies sending responses. See [Using Lambda with CloudFormation](https://docs.aws.amazon.com/lambda/latest/dg/services-cloudformation.html) for details.
*
*/
public Optional zipFile() {
return Optional.ofNullable(this.zipFile);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(CodeResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String imageUri;
private @Nullable String s3Bucket;
private @Nullable String s3Key;
private @Nullable String s3ObjectVersion;
private @Nullable String zipFile;
public Builder() {}
public Builder(CodeResponse defaults) {
Objects.requireNonNull(defaults);
this.imageUri = defaults.imageUri;
this.s3Bucket = defaults.s3Bucket;
this.s3Key = defaults.s3Key;
this.s3ObjectVersion = defaults.s3ObjectVersion;
this.zipFile = defaults.zipFile;
}
@CustomType.Setter
public Builder imageUri(@Nullable String imageUri) {
this.imageUri = imageUri;
return this;
}
@CustomType.Setter
public Builder s3Bucket(@Nullable String s3Bucket) {
this.s3Bucket = s3Bucket;
return this;
}
@CustomType.Setter
public Builder s3Key(@Nullable String s3Key) {
this.s3Key = s3Key;
return this;
}
@CustomType.Setter
public Builder s3ObjectVersion(@Nullable String s3ObjectVersion) {
this.s3ObjectVersion = s3ObjectVersion;
return this;
}
@CustomType.Setter
public Builder zipFile(@Nullable String zipFile) {
this.zipFile = zipFile;
return this;
}
public CodeResponse build() {
final var _resultValue = new CodeResponse();
_resultValue.imageUri = imageUri;
_resultValue.s3Bucket = s3Bucket;
_resultValue.s3Key = s3Key;
_resultValue.s3ObjectVersion = s3ObjectVersion;
_resultValue.zipFile = zipFile;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy