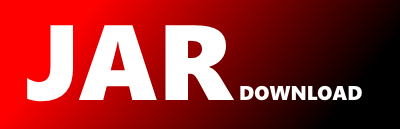
com.pulumi.azurenative.awsconnector.outputs.DiskResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DiskResponse {
/**
* @return Instance attached to the disk.
*
*/
private @Nullable String attachedTo;
/**
* @return Attachment state of the disk.
*
*/
private @Nullable String attachmentState;
/**
* @return The names to use for your new Lightsail disk.
*
*/
private @Nullable String diskName;
/**
* @return IOPS of disk.
*
*/
private @Nullable Integer iops;
/**
* @return Is the Attached disk is the system disk of the Instance.
*
*/
private @Nullable Boolean isSystemDisk;
/**
* @return Path of the disk attached to the instance.
*
*/
private @Nullable String path;
/**
* @return Size of the disk attached to the Instance.
*
*/
private @Nullable String sizeInGb;
private DiskResponse() {}
/**
* @return Instance attached to the disk.
*
*/
public Optional attachedTo() {
return Optional.ofNullable(this.attachedTo);
}
/**
* @return Attachment state of the disk.
*
*/
public Optional attachmentState() {
return Optional.ofNullable(this.attachmentState);
}
/**
* @return The names to use for your new Lightsail disk.
*
*/
public Optional diskName() {
return Optional.ofNullable(this.diskName);
}
/**
* @return IOPS of disk.
*
*/
public Optional iops() {
return Optional.ofNullable(this.iops);
}
/**
* @return Is the Attached disk is the system disk of the Instance.
*
*/
public Optional isSystemDisk() {
return Optional.ofNullable(this.isSystemDisk);
}
/**
* @return Path of the disk attached to the instance.
*
*/
public Optional path() {
return Optional.ofNullable(this.path);
}
/**
* @return Size of the disk attached to the Instance.
*
*/
public Optional sizeInGb() {
return Optional.ofNullable(this.sizeInGb);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DiskResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String attachedTo;
private @Nullable String attachmentState;
private @Nullable String diskName;
private @Nullable Integer iops;
private @Nullable Boolean isSystemDisk;
private @Nullable String path;
private @Nullable String sizeInGb;
public Builder() {}
public Builder(DiskResponse defaults) {
Objects.requireNonNull(defaults);
this.attachedTo = defaults.attachedTo;
this.attachmentState = defaults.attachmentState;
this.diskName = defaults.diskName;
this.iops = defaults.iops;
this.isSystemDisk = defaults.isSystemDisk;
this.path = defaults.path;
this.sizeInGb = defaults.sizeInGb;
}
@CustomType.Setter
public Builder attachedTo(@Nullable String attachedTo) {
this.attachedTo = attachedTo;
return this;
}
@CustomType.Setter
public Builder attachmentState(@Nullable String attachmentState) {
this.attachmentState = attachmentState;
return this;
}
@CustomType.Setter
public Builder diskName(@Nullable String diskName) {
this.diskName = diskName;
return this;
}
@CustomType.Setter
public Builder iops(@Nullable Integer iops) {
this.iops = iops;
return this;
}
@CustomType.Setter
public Builder isSystemDisk(@Nullable Boolean isSystemDisk) {
this.isSystemDisk = isSystemDisk;
return this;
}
@CustomType.Setter
public Builder path(@Nullable String path) {
this.path = path;
return this;
}
@CustomType.Setter
public Builder sizeInGb(@Nullable String sizeInGb) {
this.sizeInGb = sizeInGb;
return this;
}
public DiskResponse build() {
final var _resultValue = new DiskResponse();
_resultValue.attachedTo = attachedTo;
_resultValue.attachmentState = attachmentState;
_resultValue.diskName = diskName;
_resultValue.iops = iops;
_resultValue.isSystemDisk = isSystemDisk;
_resultValue.path = path;
_resultValue.sizeInGb = sizeInGb;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy