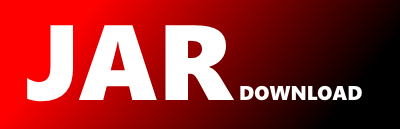
com.pulumi.azurenative.awsconnector.outputs.InstanceMetadataOptionsResponseResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.outputs;
import com.pulumi.azurenative.awsconnector.outputs.HttpTokensStateEnumValueResponse;
import com.pulumi.azurenative.awsconnector.outputs.InstanceMetadataEndpointStateEnumValueResponse;
import com.pulumi.azurenative.awsconnector.outputs.InstanceMetadataOptionsStateEnumValueResponse;
import com.pulumi.azurenative.awsconnector.outputs.InstanceMetadataProtocolStateEnumValueResponse;
import com.pulumi.azurenative.awsconnector.outputs.InstanceMetadataTagsStateEnumValueResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class InstanceMetadataOptionsResponseResponse {
/**
* @return <p>Indicates whether the HTTP metadata endpoint on your instances is enabled or disabled.</p> <p>If the value is <code>disabled</code>, you cannot access your instance metadata.</p>
*
*/
private @Nullable InstanceMetadataEndpointStateEnumValueResponse httpEndpoint;
/**
* @return <p>Indicates whether the IPv6 endpoint for the instance metadata service is enabled or disabled.</p> <p>Default: <code>disabled</code> </p>
*
*/
private @Nullable InstanceMetadataProtocolStateEnumValueResponse httpProtocolIpv6;
/**
* @return <p>The maximum number of hops that the metadata token can travel.</p> <p>Possible values: Integers from <code>1</code> to <code>64</code> </p>
*
*/
private @Nullable Integer httpPutResponseHopLimit;
/**
* @return <p>Indicates whether IMDSv2 is required.</p> <ul> <li> <p> <code>optional</code> - IMDSv2 is optional, which means that you can use either IMDSv2 or IMDSv1.</p> </li> <li> <p> <code>required</code> - IMDSv2 is required, which means that IMDSv1 is disabled, and you must use IMDSv2.</p> </li> </ul>
*
*/
private @Nullable HttpTokensStateEnumValueResponse httpTokens;
/**
* @return <p>Indicates whether access to instance tags from the instance metadata is enabled or disabled. For more information, see <a href='https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/Using_Tags.html#work-with-tags-in-IMDS'>Work with instance tags using the instance metadata</a>.</p>
*
*/
private @Nullable InstanceMetadataTagsStateEnumValueResponse instanceMetadataTags;
/**
* @return <p>The state of the metadata option changes.</p> <p> <code>pending</code> - The metadata options are being updated and the instance is not ready to process metadata traffic with the new selection.</p> <p> <code>applied</code> - The metadata options have been successfully applied on the instance.</p>
*
*/
private @Nullable InstanceMetadataOptionsStateEnumValueResponse state;
private InstanceMetadataOptionsResponseResponse() {}
/**
* @return <p>Indicates whether the HTTP metadata endpoint on your instances is enabled or disabled.</p> <p>If the value is <code>disabled</code>, you cannot access your instance metadata.</p>
*
*/
public Optional httpEndpoint() {
return Optional.ofNullable(this.httpEndpoint);
}
/**
* @return <p>Indicates whether the IPv6 endpoint for the instance metadata service is enabled or disabled.</p> <p>Default: <code>disabled</code> </p>
*
*/
public Optional httpProtocolIpv6() {
return Optional.ofNullable(this.httpProtocolIpv6);
}
/**
* @return <p>The maximum number of hops that the metadata token can travel.</p> <p>Possible values: Integers from <code>1</code> to <code>64</code> </p>
*
*/
public Optional httpPutResponseHopLimit() {
return Optional.ofNullable(this.httpPutResponseHopLimit);
}
/**
* @return <p>Indicates whether IMDSv2 is required.</p> <ul> <li> <p> <code>optional</code> - IMDSv2 is optional, which means that you can use either IMDSv2 or IMDSv1.</p> </li> <li> <p> <code>required</code> - IMDSv2 is required, which means that IMDSv1 is disabled, and you must use IMDSv2.</p> </li> </ul>
*
*/
public Optional httpTokens() {
return Optional.ofNullable(this.httpTokens);
}
/**
* @return <p>Indicates whether access to instance tags from the instance metadata is enabled or disabled. For more information, see <a href='https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/Using_Tags.html#work-with-tags-in-IMDS'>Work with instance tags using the instance metadata</a>.</p>
*
*/
public Optional instanceMetadataTags() {
return Optional.ofNullable(this.instanceMetadataTags);
}
/**
* @return <p>The state of the metadata option changes.</p> <p> <code>pending</code> - The metadata options are being updated and the instance is not ready to process metadata traffic with the new selection.</p> <p> <code>applied</code> - The metadata options have been successfully applied on the instance.</p>
*
*/
public Optional state() {
return Optional.ofNullable(this.state);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(InstanceMetadataOptionsResponseResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable InstanceMetadataEndpointStateEnumValueResponse httpEndpoint;
private @Nullable InstanceMetadataProtocolStateEnumValueResponse httpProtocolIpv6;
private @Nullable Integer httpPutResponseHopLimit;
private @Nullable HttpTokensStateEnumValueResponse httpTokens;
private @Nullable InstanceMetadataTagsStateEnumValueResponse instanceMetadataTags;
private @Nullable InstanceMetadataOptionsStateEnumValueResponse state;
public Builder() {}
public Builder(InstanceMetadataOptionsResponseResponse defaults) {
Objects.requireNonNull(defaults);
this.httpEndpoint = defaults.httpEndpoint;
this.httpProtocolIpv6 = defaults.httpProtocolIpv6;
this.httpPutResponseHopLimit = defaults.httpPutResponseHopLimit;
this.httpTokens = defaults.httpTokens;
this.instanceMetadataTags = defaults.instanceMetadataTags;
this.state = defaults.state;
}
@CustomType.Setter
public Builder httpEndpoint(@Nullable InstanceMetadataEndpointStateEnumValueResponse httpEndpoint) {
this.httpEndpoint = httpEndpoint;
return this;
}
@CustomType.Setter
public Builder httpProtocolIpv6(@Nullable InstanceMetadataProtocolStateEnumValueResponse httpProtocolIpv6) {
this.httpProtocolIpv6 = httpProtocolIpv6;
return this;
}
@CustomType.Setter
public Builder httpPutResponseHopLimit(@Nullable Integer httpPutResponseHopLimit) {
this.httpPutResponseHopLimit = httpPutResponseHopLimit;
return this;
}
@CustomType.Setter
public Builder httpTokens(@Nullable HttpTokensStateEnumValueResponse httpTokens) {
this.httpTokens = httpTokens;
return this;
}
@CustomType.Setter
public Builder instanceMetadataTags(@Nullable InstanceMetadataTagsStateEnumValueResponse instanceMetadataTags) {
this.instanceMetadataTags = instanceMetadataTags;
return this;
}
@CustomType.Setter
public Builder state(@Nullable InstanceMetadataOptionsStateEnumValueResponse state) {
this.state = state;
return this;
}
public InstanceMetadataOptionsResponseResponse build() {
final var _resultValue = new InstanceMetadataOptionsResponseResponse();
_resultValue.httpEndpoint = httpEndpoint;
_resultValue.httpProtocolIpv6 = httpProtocolIpv6;
_resultValue.httpPutResponseHopLimit = httpPutResponseHopLimit;
_resultValue.httpTokens = httpTokens;
_resultValue.instanceMetadataTags = instanceMetadataTags;
_resultValue.state = state;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy