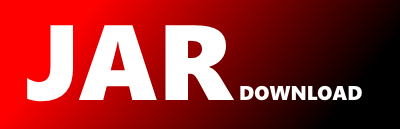
com.pulumi.azurenative.awsconnector.outputs.MethodSettingResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class MethodSettingResponse {
/**
* @return Specifies whether the cached responses are encrypted.
*
*/
private @Nullable Boolean cacheDataEncrypted;
/**
* @return Specifies the time to live (TTL), in seconds, for cached responses. The higher the TTL, the longer the response will be cached.
*
*/
private @Nullable Integer cacheTtlInSeconds;
/**
* @return Specifies whether responses should be cached and returned for requests. A cache cluster must be enabled on the stage for responses to be cached.
*
*/
private @Nullable Boolean cachingEnabled;
/**
* @return Specifies whether data trace logging is enabled for this method, which affects the log entries pushed to Amazon CloudWatch Logs. This can be useful to troubleshoot APIs, but can result in logging sensitive data. We recommend that you don't enable this option for production APIs.
*
*/
private @Nullable Boolean dataTraceEnabled;
/**
* @return The HTTP method. To apply settings to multiple resources and methods, specify an asterisk (``*``) for the ``HttpMethod`` and ``/*`` for the ``ResourcePath``. This parameter is required when you specify a ``MethodSetting``.
*
*/
private @Nullable String httpMethod;
/**
* @return Specifies the logging level for this method, which affects the log entries pushed to Amazon CloudWatch Logs. Valid values are ``OFF``, ``ERROR``, and ``INFO``. Choose ``ERROR`` to write only error-level entries to CloudWatch Logs, or choose ``INFO`` to include all ``ERROR`` events as well as extra informational events.
*
*/
private @Nullable String loggingLevel;
/**
* @return Specifies whether Amazon CloudWatch metrics are enabled for this method.
*
*/
private @Nullable Boolean metricsEnabled;
/**
* @return The resource path for this method. Forward slashes (``/``) are encoded as ``~1`` and the initial slash must include a forward slash. For example, the path value ``/resource/subresource`` must be encoded as ``/~1resource~1subresource``. To specify the root path, use only a slash (``/``). To apply settings to multiple resources and methods, specify an asterisk (``*``) for the ``HttpMethod`` and ``/*`` for the ``ResourcePath``. This parameter is required when you specify a ``MethodSetting``.
*
*/
private @Nullable String resourcePath;
/**
* @return Specifies the throttling burst limit.
*
*/
private @Nullable Integer throttlingBurstLimit;
/**
* @return Specifies the throttling rate limit.
*
*/
private @Nullable Integer throttlingRateLimit;
private MethodSettingResponse() {}
/**
* @return Specifies whether the cached responses are encrypted.
*
*/
public Optional cacheDataEncrypted() {
return Optional.ofNullable(this.cacheDataEncrypted);
}
/**
* @return Specifies the time to live (TTL), in seconds, for cached responses. The higher the TTL, the longer the response will be cached.
*
*/
public Optional cacheTtlInSeconds() {
return Optional.ofNullable(this.cacheTtlInSeconds);
}
/**
* @return Specifies whether responses should be cached and returned for requests. A cache cluster must be enabled on the stage for responses to be cached.
*
*/
public Optional cachingEnabled() {
return Optional.ofNullable(this.cachingEnabled);
}
/**
* @return Specifies whether data trace logging is enabled for this method, which affects the log entries pushed to Amazon CloudWatch Logs. This can be useful to troubleshoot APIs, but can result in logging sensitive data. We recommend that you don't enable this option for production APIs.
*
*/
public Optional dataTraceEnabled() {
return Optional.ofNullable(this.dataTraceEnabled);
}
/**
* @return The HTTP method. To apply settings to multiple resources and methods, specify an asterisk (``*``) for the ``HttpMethod`` and ``/*`` for the ``ResourcePath``. This parameter is required when you specify a ``MethodSetting``.
*
*/
public Optional httpMethod() {
return Optional.ofNullable(this.httpMethod);
}
/**
* @return Specifies the logging level for this method, which affects the log entries pushed to Amazon CloudWatch Logs. Valid values are ``OFF``, ``ERROR``, and ``INFO``. Choose ``ERROR`` to write only error-level entries to CloudWatch Logs, or choose ``INFO`` to include all ``ERROR`` events as well as extra informational events.
*
*/
public Optional loggingLevel() {
return Optional.ofNullable(this.loggingLevel);
}
/**
* @return Specifies whether Amazon CloudWatch metrics are enabled for this method.
*
*/
public Optional metricsEnabled() {
return Optional.ofNullable(this.metricsEnabled);
}
/**
* @return The resource path for this method. Forward slashes (``/``) are encoded as ``~1`` and the initial slash must include a forward slash. For example, the path value ``/resource/subresource`` must be encoded as ``/~1resource~1subresource``. To specify the root path, use only a slash (``/``). To apply settings to multiple resources and methods, specify an asterisk (``*``) for the ``HttpMethod`` and ``/*`` for the ``ResourcePath``. This parameter is required when you specify a ``MethodSetting``.
*
*/
public Optional resourcePath() {
return Optional.ofNullable(this.resourcePath);
}
/**
* @return Specifies the throttling burst limit.
*
*/
public Optional throttlingBurstLimit() {
return Optional.ofNullable(this.throttlingBurstLimit);
}
/**
* @return Specifies the throttling rate limit.
*
*/
public Optional throttlingRateLimit() {
return Optional.ofNullable(this.throttlingRateLimit);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(MethodSettingResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean cacheDataEncrypted;
private @Nullable Integer cacheTtlInSeconds;
private @Nullable Boolean cachingEnabled;
private @Nullable Boolean dataTraceEnabled;
private @Nullable String httpMethod;
private @Nullable String loggingLevel;
private @Nullable Boolean metricsEnabled;
private @Nullable String resourcePath;
private @Nullable Integer throttlingBurstLimit;
private @Nullable Integer throttlingRateLimit;
public Builder() {}
public Builder(MethodSettingResponse defaults) {
Objects.requireNonNull(defaults);
this.cacheDataEncrypted = defaults.cacheDataEncrypted;
this.cacheTtlInSeconds = defaults.cacheTtlInSeconds;
this.cachingEnabled = defaults.cachingEnabled;
this.dataTraceEnabled = defaults.dataTraceEnabled;
this.httpMethod = defaults.httpMethod;
this.loggingLevel = defaults.loggingLevel;
this.metricsEnabled = defaults.metricsEnabled;
this.resourcePath = defaults.resourcePath;
this.throttlingBurstLimit = defaults.throttlingBurstLimit;
this.throttlingRateLimit = defaults.throttlingRateLimit;
}
@CustomType.Setter
public Builder cacheDataEncrypted(@Nullable Boolean cacheDataEncrypted) {
this.cacheDataEncrypted = cacheDataEncrypted;
return this;
}
@CustomType.Setter
public Builder cacheTtlInSeconds(@Nullable Integer cacheTtlInSeconds) {
this.cacheTtlInSeconds = cacheTtlInSeconds;
return this;
}
@CustomType.Setter
public Builder cachingEnabled(@Nullable Boolean cachingEnabled) {
this.cachingEnabled = cachingEnabled;
return this;
}
@CustomType.Setter
public Builder dataTraceEnabled(@Nullable Boolean dataTraceEnabled) {
this.dataTraceEnabled = dataTraceEnabled;
return this;
}
@CustomType.Setter
public Builder httpMethod(@Nullable String httpMethod) {
this.httpMethod = httpMethod;
return this;
}
@CustomType.Setter
public Builder loggingLevel(@Nullable String loggingLevel) {
this.loggingLevel = loggingLevel;
return this;
}
@CustomType.Setter
public Builder metricsEnabled(@Nullable Boolean metricsEnabled) {
this.metricsEnabled = metricsEnabled;
return this;
}
@CustomType.Setter
public Builder resourcePath(@Nullable String resourcePath) {
this.resourcePath = resourcePath;
return this;
}
@CustomType.Setter
public Builder throttlingBurstLimit(@Nullable Integer throttlingBurstLimit) {
this.throttlingBurstLimit = throttlingBurstLimit;
return this;
}
@CustomType.Setter
public Builder throttlingRateLimit(@Nullable Integer throttlingRateLimit) {
this.throttlingRateLimit = throttlingRateLimit;
return this;
}
public MethodSettingResponse build() {
final var _resultValue = new MethodSettingResponse();
_resultValue.cacheDataEncrypted = cacheDataEncrypted;
_resultValue.cacheTtlInSeconds = cacheTtlInSeconds;
_resultValue.cachingEnabled = cachingEnabled;
_resultValue.dataTraceEnabled = dataTraceEnabled;
_resultValue.httpMethod = httpMethod;
_resultValue.loggingLevel = loggingLevel;
_resultValue.metricsEnabled = metricsEnabled;
_resultValue.resourcePath = resourcePath;
_resultValue.throttlingBurstLimit = throttlingBurstLimit;
_resultValue.throttlingRateLimit = throttlingRateLimit;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy