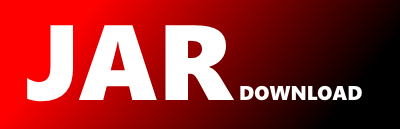
com.pulumi.azurenative.awsconnector.outputs.ViewerCertificateResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ViewerCertificateResponse {
/**
* @return In CloudFormation, this field name is ``AcmCertificateArn``. Note the different capitalization. If the distribution uses ``Aliases`` (alternate domain names or CNAMEs) and the SSL/TLS certificate is stored in [(ACM)](https://docs.aws.amazon.com/acm/latest/userguide/acm-overview.html), provide the Amazon Resource Name (ARN) of the ACM certificate. CloudFront only supports ACM certificates in the US East (N. Virginia) Region (``us-east-1``). If you specify an ACM certificate ARN, you must also specify values for ``MinimumProtocolVersion`` and ``SSLSupportMethod``. (In CloudFormation, the field name is ``SslSupportMethod``. Note the different capitalization.)
*
*/
private @Nullable String acmCertificateArn;
/**
* @return If the distribution uses the CloudFront domain name such as ``d111111abcdef8.cloudfront.net``, set this field to ``true``. If the distribution uses ``Aliases`` (alternate domain names or CNAMEs), omit this field and specify values for the following fields: + ``AcmCertificateArn`` or ``IamCertificateId`` (specify a value for one, not both) + ``MinimumProtocolVersion`` + ``SslSupportMethod``
*
*/
private @Nullable Boolean cloudFrontDefaultCertificate;
/**
* @return In CloudFormation, this field name is ``IamCertificateId``. Note the different capitalization. If the distribution uses ``Aliases`` (alternate domain names or CNAMEs) and the SSL/TLS certificate is stored in [(IAM)](https://docs.aws.amazon.com/IAM/latest/UserGuide/id_credentials_server-certs.html), provide the ID of the IAM certificate. If you specify an IAM certificate ID, you must also specify values for ``MinimumProtocolVersion`` and ``SSLSupportMethod``. (In CloudFormation, the field name is ``SslSupportMethod``. Note the different capitalization.)
*
*/
private @Nullable String iamCertificateId;
/**
* @return If the distribution uses ``Aliases`` (alternate domain names or CNAMEs), specify the security policy that you want CloudFront to use for HTTPS connections with viewers. The security policy determines two settings: + The minimum SSL/TLS protocol that CloudFront can use to communicate with viewers. + The ciphers that CloudFront can use to encrypt the content that it returns to viewers. For more information, see [Security Policy](https://docs.aws.amazon.com/AmazonCloudFront/latest/DeveloperGuide/distribution-web-values-specify.html#DownloadDistValues-security-policy) and [Supported Protocols and Ciphers Between Viewers and CloudFront](https://docs.aws.amazon.com/AmazonCloudFront/latest/DeveloperGuide/secure-connections-supported-viewer-protocols-ciphers.html#secure-connections-supported-ciphers) in the *Amazon CloudFront Developer Guide*. On the CloudFront console, this setting is called *Security Policy*. When you're using SNI only (you set ``SSLSupportMethod`` to ``sni-only``), you must specify ``TLSv1`` or higher. (In CloudFormation, the field name is ``SslSupportMethod``. Note the different capitalization.) If the distribution uses the CloudFront domain name such as ``d111111abcdef8.cloudfront.net`` (you set ``CloudFrontDefaultCertificate`` to ``true``), CloudFront automatically sets the security policy to ``TLSv1`` regardless of the value that you set here.
*
*/
private @Nullable String minimumProtocolVersion;
/**
* @return In CloudFormation, this field name is ``SslSupportMethod``. Note the different capitalization. If the distribution uses ``Aliases`` (alternate domain names or CNAMEs), specify which viewers the distribution accepts HTTPS connections from. + ``sni-only`` – The distribution accepts HTTPS connections from only viewers that support [server name indication (SNI)](https://docs.aws.amazon.com/https://en.wikipedia.org/wiki/Server_Name_Indication). This is recommended. Most browsers and clients support SNI. + ``vip`` – The distribution accepts HTTPS connections from all viewers including those that don't support SNI. This is not recommended, and results in additional monthly charges from CloudFront. + ``static-ip`` - Do not specify this value unless your distribution has been enabled for this feature by the CloudFront team. If you have a use case that requires static IP addresses for a distribution, contact CloudFront through the [Center](https://docs.aws.amazon.com/support/home). If the distribution uses the CloudFront domain name such as ``d111111abcdef8.cloudfront.net``, don't set a value for this field.
*
*/
private @Nullable String sslSupportMethod;
private ViewerCertificateResponse() {}
/**
* @return In CloudFormation, this field name is ``AcmCertificateArn``. Note the different capitalization. If the distribution uses ``Aliases`` (alternate domain names or CNAMEs) and the SSL/TLS certificate is stored in [(ACM)](https://docs.aws.amazon.com/acm/latest/userguide/acm-overview.html), provide the Amazon Resource Name (ARN) of the ACM certificate. CloudFront only supports ACM certificates in the US East (N. Virginia) Region (``us-east-1``). If you specify an ACM certificate ARN, you must also specify values for ``MinimumProtocolVersion`` and ``SSLSupportMethod``. (In CloudFormation, the field name is ``SslSupportMethod``. Note the different capitalization.)
*
*/
public Optional acmCertificateArn() {
return Optional.ofNullable(this.acmCertificateArn);
}
/**
* @return If the distribution uses the CloudFront domain name such as ``d111111abcdef8.cloudfront.net``, set this field to ``true``. If the distribution uses ``Aliases`` (alternate domain names or CNAMEs), omit this field and specify values for the following fields: + ``AcmCertificateArn`` or ``IamCertificateId`` (specify a value for one, not both) + ``MinimumProtocolVersion`` + ``SslSupportMethod``
*
*/
public Optional cloudFrontDefaultCertificate() {
return Optional.ofNullable(this.cloudFrontDefaultCertificate);
}
/**
* @return In CloudFormation, this field name is ``IamCertificateId``. Note the different capitalization. If the distribution uses ``Aliases`` (alternate domain names or CNAMEs) and the SSL/TLS certificate is stored in [(IAM)](https://docs.aws.amazon.com/IAM/latest/UserGuide/id_credentials_server-certs.html), provide the ID of the IAM certificate. If you specify an IAM certificate ID, you must also specify values for ``MinimumProtocolVersion`` and ``SSLSupportMethod``. (In CloudFormation, the field name is ``SslSupportMethod``. Note the different capitalization.)
*
*/
public Optional iamCertificateId() {
return Optional.ofNullable(this.iamCertificateId);
}
/**
* @return If the distribution uses ``Aliases`` (alternate domain names or CNAMEs), specify the security policy that you want CloudFront to use for HTTPS connections with viewers. The security policy determines two settings: + The minimum SSL/TLS protocol that CloudFront can use to communicate with viewers. + The ciphers that CloudFront can use to encrypt the content that it returns to viewers. For more information, see [Security Policy](https://docs.aws.amazon.com/AmazonCloudFront/latest/DeveloperGuide/distribution-web-values-specify.html#DownloadDistValues-security-policy) and [Supported Protocols and Ciphers Between Viewers and CloudFront](https://docs.aws.amazon.com/AmazonCloudFront/latest/DeveloperGuide/secure-connections-supported-viewer-protocols-ciphers.html#secure-connections-supported-ciphers) in the *Amazon CloudFront Developer Guide*. On the CloudFront console, this setting is called *Security Policy*. When you're using SNI only (you set ``SSLSupportMethod`` to ``sni-only``), you must specify ``TLSv1`` or higher. (In CloudFormation, the field name is ``SslSupportMethod``. Note the different capitalization.) If the distribution uses the CloudFront domain name such as ``d111111abcdef8.cloudfront.net`` (you set ``CloudFrontDefaultCertificate`` to ``true``), CloudFront automatically sets the security policy to ``TLSv1`` regardless of the value that you set here.
*
*/
public Optional minimumProtocolVersion() {
return Optional.ofNullable(this.minimumProtocolVersion);
}
/**
* @return In CloudFormation, this field name is ``SslSupportMethod``. Note the different capitalization. If the distribution uses ``Aliases`` (alternate domain names or CNAMEs), specify which viewers the distribution accepts HTTPS connections from. + ``sni-only`` – The distribution accepts HTTPS connections from only viewers that support [server name indication (SNI)](https://docs.aws.amazon.com/https://en.wikipedia.org/wiki/Server_Name_Indication). This is recommended. Most browsers and clients support SNI. + ``vip`` – The distribution accepts HTTPS connections from all viewers including those that don't support SNI. This is not recommended, and results in additional monthly charges from CloudFront. + ``static-ip`` - Do not specify this value unless your distribution has been enabled for this feature by the CloudFront team. If you have a use case that requires static IP addresses for a distribution, contact CloudFront through the [Center](https://docs.aws.amazon.com/support/home). If the distribution uses the CloudFront domain name such as ``d111111abcdef8.cloudfront.net``, don't set a value for this field.
*
*/
public Optional sslSupportMethod() {
return Optional.ofNullable(this.sslSupportMethod);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ViewerCertificateResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String acmCertificateArn;
private @Nullable Boolean cloudFrontDefaultCertificate;
private @Nullable String iamCertificateId;
private @Nullable String minimumProtocolVersion;
private @Nullable String sslSupportMethod;
public Builder() {}
public Builder(ViewerCertificateResponse defaults) {
Objects.requireNonNull(defaults);
this.acmCertificateArn = defaults.acmCertificateArn;
this.cloudFrontDefaultCertificate = defaults.cloudFrontDefaultCertificate;
this.iamCertificateId = defaults.iamCertificateId;
this.minimumProtocolVersion = defaults.minimumProtocolVersion;
this.sslSupportMethod = defaults.sslSupportMethod;
}
@CustomType.Setter
public Builder acmCertificateArn(@Nullable String acmCertificateArn) {
this.acmCertificateArn = acmCertificateArn;
return this;
}
@CustomType.Setter
public Builder cloudFrontDefaultCertificate(@Nullable Boolean cloudFrontDefaultCertificate) {
this.cloudFrontDefaultCertificate = cloudFrontDefaultCertificate;
return this;
}
@CustomType.Setter
public Builder iamCertificateId(@Nullable String iamCertificateId) {
this.iamCertificateId = iamCertificateId;
return this;
}
@CustomType.Setter
public Builder minimumProtocolVersion(@Nullable String minimumProtocolVersion) {
this.minimumProtocolVersion = minimumProtocolVersion;
return this;
}
@CustomType.Setter
public Builder sslSupportMethod(@Nullable String sslSupportMethod) {
this.sslSupportMethod = sslSupportMethod;
return this;
}
public ViewerCertificateResponse build() {
final var _resultValue = new ViewerCertificateResponse();
_resultValue.acmCertificateArn = acmCertificateArn;
_resultValue.cloudFrontDefaultCertificate = cloudFrontDefaultCertificate;
_resultValue.iamCertificateId = iamCertificateId;
_resultValue.minimumProtocolVersion = minimumProtocolVersion;
_resultValue.sslSupportMethod = sslSupportMethod;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy