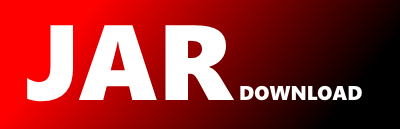
com.pulumi.azurenative.awsconnector.outputs.VolumeResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.awsconnector.outputs;
import com.pulumi.azurenative.awsconnector.outputs.DockerVolumeConfigurationResponse;
import com.pulumi.azurenative.awsconnector.outputs.EFSVolumeConfigurationResponse;
import com.pulumi.azurenative.awsconnector.outputs.FSxWindowsFileServerVolumeConfigurationResponse;
import com.pulumi.azurenative.awsconnector.outputs.HostVolumePropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class VolumeResponse {
/**
* @return Indicates whether the volume should be configured at launch time. This is used to create Amazon EBS volumes for standalone tasks or tasks created as part of a service. Each task definition revision may only have one volume configured at launch in the volume configuration. To configure a volume at launch time, use this task definition revision and specify a ``volumeConfigurations`` object when calling the ``CreateService``, ``UpdateService``, ``RunTask`` or ``StartTask`` APIs.
*
*/
private @Nullable Boolean configuredAtLaunch;
/**
* @return This parameter is specified when you use Docker volumes. Windows containers only support the use of the ``local`` driver. To use bind mounts, specify the ``host`` parameter instead. Docker volumes aren't supported by tasks run on FARGATElong. The ``DockerVolumeConfiguration`` property specifies a Docker volume configuration and is used when you use Docker volumes. Docker volumes are only supported when you are using the EC2 launch type. Windows containers only support the use of the ``local`` driver. To use bind mounts, specify a ``host`` instead.
*
*/
private @Nullable DockerVolumeConfigurationResponse dockerVolumeConfiguration;
/**
* @return This parameter is specified when you use an Amazon Elastic File System file system for task storage. This parameter is specified when you're using an Amazon Elastic File System file system for task storage. For more information, see [Amazon EFS volumes](https://docs.aws.amazon.com/AmazonECS/latest/developerguide/efs-volumes.html) in the *Amazon Elastic Container Service Developer Guide*.
*
*/
private @Nullable EFSVolumeConfigurationResponse efsVolumeConfiguration;
/**
* @return This parameter is specified when you use Amazon FSx for Windows File Server file system for task storage. This parameter is specified when you're using [Amazon FSx for Windows File Server](https://docs.aws.amazon.com/fsx/latest/WindowsGuide/what-is.html) file system for task storage. For more information and the input format, see [Amazon FSx for Windows File Server volumes](https://docs.aws.amazon.com/AmazonECS/latest/developerguide/wfsx-volumes.html) in the *Amazon Elastic Container Service Developer Guide*.
*
*/
private @Nullable FSxWindowsFileServerVolumeConfigurationResponse fSxWindowsFileServerVolumeConfiguration;
/**
* @return This parameter is specified when you use bind mount host volumes. The contents of the ``host`` parameter determine whether your bind mount host volume persists on the host container instance and where it's stored. If the ``host`` parameter is empty, then the Docker daemon assigns a host path for your data volume. However, the data isn't guaranteed to persist after the containers that are associated with it stop running. Windows containers can mount whole directories on the same drive as ``$env:ProgramData``. Windows containers can't mount directories on a different drive, and mount point can't be across drives. For example, you can mount ``C:\my\path:C:\my\path`` and ``D:\:D:\``, but not ``D:\my\path:C:\my\path`` or ``D:\:C:\my\path``. The ``HostVolumeProperties`` property specifies details on a container instance bind mount host volume.
*
*/
private @Nullable HostVolumePropertiesResponse host;
/**
* @return The name of the volume. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are allowed. When using a volume configured at launch, the ``name`` is required and must also be specified as the volume name in the ``ServiceVolumeConfiguration`` or ``TaskVolumeConfiguration`` parameter when creating your service or standalone task. For all other types of volumes, this name is referenced in the ``sourceVolume`` parameter of the ``mountPoints`` object in the container definition. When a volume is using the ``efsVolumeConfiguration``, the name is required.
*
*/
private @Nullable String name;
private VolumeResponse() {}
/**
* @return Indicates whether the volume should be configured at launch time. This is used to create Amazon EBS volumes for standalone tasks or tasks created as part of a service. Each task definition revision may only have one volume configured at launch in the volume configuration. To configure a volume at launch time, use this task definition revision and specify a ``volumeConfigurations`` object when calling the ``CreateService``, ``UpdateService``, ``RunTask`` or ``StartTask`` APIs.
*
*/
public Optional configuredAtLaunch() {
return Optional.ofNullable(this.configuredAtLaunch);
}
/**
* @return This parameter is specified when you use Docker volumes. Windows containers only support the use of the ``local`` driver. To use bind mounts, specify the ``host`` parameter instead. Docker volumes aren't supported by tasks run on FARGATElong. The ``DockerVolumeConfiguration`` property specifies a Docker volume configuration and is used when you use Docker volumes. Docker volumes are only supported when you are using the EC2 launch type. Windows containers only support the use of the ``local`` driver. To use bind mounts, specify a ``host`` instead.
*
*/
public Optional dockerVolumeConfiguration() {
return Optional.ofNullable(this.dockerVolumeConfiguration);
}
/**
* @return This parameter is specified when you use an Amazon Elastic File System file system for task storage. This parameter is specified when you're using an Amazon Elastic File System file system for task storage. For more information, see [Amazon EFS volumes](https://docs.aws.amazon.com/AmazonECS/latest/developerguide/efs-volumes.html) in the *Amazon Elastic Container Service Developer Guide*.
*
*/
public Optional efsVolumeConfiguration() {
return Optional.ofNullable(this.efsVolumeConfiguration);
}
/**
* @return This parameter is specified when you use Amazon FSx for Windows File Server file system for task storage. This parameter is specified when you're using [Amazon FSx for Windows File Server](https://docs.aws.amazon.com/fsx/latest/WindowsGuide/what-is.html) file system for task storage. For more information and the input format, see [Amazon FSx for Windows File Server volumes](https://docs.aws.amazon.com/AmazonECS/latest/developerguide/wfsx-volumes.html) in the *Amazon Elastic Container Service Developer Guide*.
*
*/
public Optional fSxWindowsFileServerVolumeConfiguration() {
return Optional.ofNullable(this.fSxWindowsFileServerVolumeConfiguration);
}
/**
* @return This parameter is specified when you use bind mount host volumes. The contents of the ``host`` parameter determine whether your bind mount host volume persists on the host container instance and where it's stored. If the ``host`` parameter is empty, then the Docker daemon assigns a host path for your data volume. However, the data isn't guaranteed to persist after the containers that are associated with it stop running. Windows containers can mount whole directories on the same drive as ``$env:ProgramData``. Windows containers can't mount directories on a different drive, and mount point can't be across drives. For example, you can mount ``C:\my\path:C:\my\path`` and ``D:\:D:\``, but not ``D:\my\path:C:\my\path`` or ``D:\:C:\my\path``. The ``HostVolumeProperties`` property specifies details on a container instance bind mount host volume.
*
*/
public Optional host() {
return Optional.ofNullable(this.host);
}
/**
* @return The name of the volume. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are allowed. When using a volume configured at launch, the ``name`` is required and must also be specified as the volume name in the ``ServiceVolumeConfiguration`` or ``TaskVolumeConfiguration`` parameter when creating your service or standalone task. For all other types of volumes, this name is referenced in the ``sourceVolume`` parameter of the ``mountPoints`` object in the container definition. When a volume is using the ``efsVolumeConfiguration``, the name is required.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(VolumeResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean configuredAtLaunch;
private @Nullable DockerVolumeConfigurationResponse dockerVolumeConfiguration;
private @Nullable EFSVolumeConfigurationResponse efsVolumeConfiguration;
private @Nullable FSxWindowsFileServerVolumeConfigurationResponse fSxWindowsFileServerVolumeConfiguration;
private @Nullable HostVolumePropertiesResponse host;
private @Nullable String name;
public Builder() {}
public Builder(VolumeResponse defaults) {
Objects.requireNonNull(defaults);
this.configuredAtLaunch = defaults.configuredAtLaunch;
this.dockerVolumeConfiguration = defaults.dockerVolumeConfiguration;
this.efsVolumeConfiguration = defaults.efsVolumeConfiguration;
this.fSxWindowsFileServerVolumeConfiguration = defaults.fSxWindowsFileServerVolumeConfiguration;
this.host = defaults.host;
this.name = defaults.name;
}
@CustomType.Setter
public Builder configuredAtLaunch(@Nullable Boolean configuredAtLaunch) {
this.configuredAtLaunch = configuredAtLaunch;
return this;
}
@CustomType.Setter
public Builder dockerVolumeConfiguration(@Nullable DockerVolumeConfigurationResponse dockerVolumeConfiguration) {
this.dockerVolumeConfiguration = dockerVolumeConfiguration;
return this;
}
@CustomType.Setter
public Builder efsVolumeConfiguration(@Nullable EFSVolumeConfigurationResponse efsVolumeConfiguration) {
this.efsVolumeConfiguration = efsVolumeConfiguration;
return this;
}
@CustomType.Setter
public Builder fSxWindowsFileServerVolumeConfiguration(@Nullable FSxWindowsFileServerVolumeConfigurationResponse fSxWindowsFileServerVolumeConfiguration) {
this.fSxWindowsFileServerVolumeConfiguration = fSxWindowsFileServerVolumeConfiguration;
return this;
}
@CustomType.Setter
public Builder host(@Nullable HostVolumePropertiesResponse host) {
this.host = host;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
public VolumeResponse build() {
final var _resultValue = new VolumeResponse();
_resultValue.configuredAtLaunch = configuredAtLaunch;
_resultValue.dockerVolumeConfiguration = dockerVolumeConfiguration;
_resultValue.efsVolumeConfiguration = efsVolumeConfiguration;
_resultValue.fSxWindowsFileServerVolumeConfiguration = fSxWindowsFileServerVolumeConfiguration;
_resultValue.host = host;
_resultValue.name = name;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy