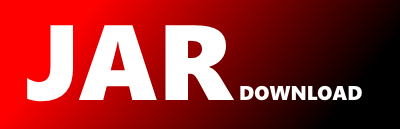
com.pulumi.azurenative.azurestackhci.Cluster Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.azurestackhci;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.azurestackhci.ClusterArgs;
import com.pulumi.azurenative.azurestackhci.outputs.ClusterDesiredPropertiesResponse;
import com.pulumi.azurenative.azurestackhci.outputs.ClusterReportedPropertiesResponse;
import com.pulumi.azurenative.azurestackhci.outputs.SoftwareAssurancePropertiesResponse;
import com.pulumi.azurenative.azurestackhci.outputs.SystemDataResponse;
import com.pulumi.azurenative.azurestackhci.outputs.UserAssignedIdentityResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Cluster details.
* Azure REST API version: 2023-03-01. Prior API version in Azure Native 1.x: 2020-10-01.
*
* Other available API versions: 2022-01-01, 2022-09-01, 2022-12-15-preview, 2023-06-01, 2023-08-01, 2023-08-01-preview, 2023-11-01-preview, 2024-01-01, 2024-02-15-preview, 2024-04-01, 2024-09-01-preview.
*
* ## Example Usage
* ### Create cluster
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.azurestackhci.Cluster;
* import com.pulumi.azurenative.azurestackhci.ClusterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var cluster = new Cluster("cluster", ClusterArgs.builder()
* .aadClientId("24a6e53d-04e5-44d2-b7cc-1b732a847dfc")
* .aadTenantId("7e589cc1-a8b6-4dff-91bd-5ec0fa18db94")
* .cloudManagementEndpoint("https://98294836-31be-4668-aeae-698667faf99b.waconazure.com")
* .clusterName("myCluster")
* .location("East US")
* .resourceGroupName("test-rg")
* .type("SystemAssigned")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:azurestackhci:Cluster myCluster /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.AzureStackHCI/clusters/{clusterName}
* ```
*
*/
@ResourceType(type="azure-native:azurestackhci:Cluster")
public class Cluster extends com.pulumi.resources.CustomResource {
/**
* Object id of cluster AAD identity.
*
*/
@Export(name="aadApplicationObjectId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> aadApplicationObjectId;
/**
* @return Object id of cluster AAD identity.
*
*/
public Output> aadApplicationObjectId() {
return Codegen.optional(this.aadApplicationObjectId);
}
/**
* App id of cluster AAD identity.
*
*/
@Export(name="aadClientId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> aadClientId;
/**
* @return App id of cluster AAD identity.
*
*/
public Output> aadClientId() {
return Codegen.optional(this.aadClientId);
}
/**
* Id of cluster identity service principal.
*
*/
@Export(name="aadServicePrincipalObjectId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> aadServicePrincipalObjectId;
/**
* @return Id of cluster identity service principal.
*
*/
public Output> aadServicePrincipalObjectId() {
return Codegen.optional(this.aadServicePrincipalObjectId);
}
/**
* Tenant id of cluster AAD identity.
*
*/
@Export(name="aadTenantId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> aadTenantId;
/**
* @return Tenant id of cluster AAD identity.
*
*/
public Output> aadTenantId() {
return Codegen.optional(this.aadTenantId);
}
/**
* Type of billing applied to the resource.
*
*/
@Export(name="billingModel", refs={String.class}, tree="[0]")
private Output billingModel;
/**
* @return Type of billing applied to the resource.
*
*/
public Output billingModel() {
return this.billingModel;
}
/**
* Unique, immutable resource id.
*
*/
@Export(name="cloudId", refs={String.class}, tree="[0]")
private Output cloudId;
/**
* @return Unique, immutable resource id.
*
*/
public Output cloudId() {
return this.cloudId;
}
/**
* Endpoint configured for management from the Azure portal.
*
*/
@Export(name="cloudManagementEndpoint", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> cloudManagementEndpoint;
/**
* @return Endpoint configured for management from the Azure portal.
*
*/
public Output> cloudManagementEndpoint() {
return Codegen.optional(this.cloudManagementEndpoint);
}
/**
* Desired properties of the cluster.
*
*/
@Export(name="desiredProperties", refs={ClusterDesiredPropertiesResponse.class}, tree="[0]")
private Output* @Nullable */ ClusterDesiredPropertiesResponse> desiredProperties;
/**
* @return Desired properties of the cluster.
*
*/
public Output> desiredProperties() {
return Codegen.optional(this.desiredProperties);
}
/**
* Most recent billing meter timestamp.
*
*/
@Export(name="lastBillingTimestamp", refs={String.class}, tree="[0]")
private Output lastBillingTimestamp;
/**
* @return Most recent billing meter timestamp.
*
*/
public Output lastBillingTimestamp() {
return this.lastBillingTimestamp;
}
/**
* Most recent cluster sync timestamp.
*
*/
@Export(name="lastSyncTimestamp", refs={String.class}, tree="[0]")
private Output lastSyncTimestamp;
/**
* @return Most recent cluster sync timestamp.
*
*/
public Output lastSyncTimestamp() {
return this.lastSyncTimestamp;
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The geo-location where the resource lives
*
*/
public Output location() {
return this.location;
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* The service principal ID of the system assigned identity. This property will only be provided for a system assigned identity.
*
*/
@Export(name="principalId", refs={String.class}, tree="[0]")
private Output principalId;
/**
* @return The service principal ID of the system assigned identity. This property will only be provided for a system assigned identity.
*
*/
public Output principalId() {
return this.principalId;
}
/**
* Provisioning state.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Provisioning state.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* First cluster sync timestamp.
*
*/
@Export(name="registrationTimestamp", refs={String.class}, tree="[0]")
private Output registrationTimestamp;
/**
* @return First cluster sync timestamp.
*
*/
public Output registrationTimestamp() {
return this.registrationTimestamp;
}
/**
* Properties reported by cluster agent.
*
*/
@Export(name="reportedProperties", refs={ClusterReportedPropertiesResponse.class}, tree="[0]")
private Output reportedProperties;
/**
* @return Properties reported by cluster agent.
*
*/
public Output reportedProperties() {
return this.reportedProperties;
}
/**
* Object id of RP Service Principal
*
*/
@Export(name="resourceProviderObjectId", refs={String.class}, tree="[0]")
private Output resourceProviderObjectId;
/**
* @return Object id of RP Service Principal
*
*/
public Output resourceProviderObjectId() {
return this.resourceProviderObjectId;
}
/**
* Region specific DataPath Endpoint of the cluster.
*
*/
@Export(name="serviceEndpoint", refs={String.class}, tree="[0]")
private Output serviceEndpoint;
/**
* @return Region specific DataPath Endpoint of the cluster.
*
*/
public Output serviceEndpoint() {
return this.serviceEndpoint;
}
/**
* Software Assurance properties of the cluster.
*
*/
@Export(name="softwareAssuranceProperties", refs={SoftwareAssurancePropertiesResponse.class}, tree="[0]")
private Output* @Nullable */ SoftwareAssurancePropertiesResponse> softwareAssuranceProperties;
/**
* @return Software Assurance properties of the cluster.
*
*/
public Output> softwareAssuranceProperties() {
return Codegen.optional(this.softwareAssuranceProperties);
}
/**
* Status of the cluster agent.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return Status of the cluster agent.
*
*/
public Output status() {
return this.status;
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* The tenant ID of the system assigned identity. This property will only be provided for a system assigned identity.
*
*/
@Export(name="tenantId", refs={String.class}, tree="[0]")
private Output tenantId;
/**
* @return The tenant ID of the system assigned identity. This property will only be provided for a system assigned identity.
*
*/
public Output tenantId() {
return this.tenantId;
}
/**
* Number of days remaining in the trial period.
*
*/
@Export(name="trialDaysRemaining", refs={Double.class}, tree="[0]")
private Output trialDaysRemaining;
/**
* @return Number of days remaining in the trial period.
*
*/
public Output trialDaysRemaining() {
return this.trialDaysRemaining;
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
* The set of user assigned identities associated with the resource. The userAssignedIdentities dictionary keys will be ARM resource ids in the form: '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ManagedIdentity/userAssignedIdentities/{identityName}. The dictionary values can be empty objects ({}) in requests.
*
*/
@Export(name="userAssignedIdentities", refs={Map.class,String.class,UserAssignedIdentityResponse.class}, tree="[0,1,2]")
private Output* @Nullable */ Map> userAssignedIdentities;
/**
* @return The set of user assigned identities associated with the resource. The userAssignedIdentities dictionary keys will be ARM resource ids in the form: '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ManagedIdentity/userAssignedIdentities/{identityName}. The dictionary values can be empty objects ({}) in requests.
*
*/
public Output>> userAssignedIdentities() {
return Codegen.optional(this.userAssignedIdentities);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Cluster(java.lang.String name) {
this(name, ClusterArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Cluster(java.lang.String name, ClusterArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Cluster(java.lang.String name, ClusterArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:azurestackhci:Cluster", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Cluster(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:azurestackhci:Cluster", name, null, makeResourceOptions(options, id), false);
}
private static ClusterArgs makeArgs(ClusterArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ClusterArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:azurestackhci/v20200301preview:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20201001:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20210101preview:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20210901:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20210901preview:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20220101:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20220301:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20220501:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20220901:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20221001:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20221201:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20221215preview:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20230201:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20230301:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20230601:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20230801:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20230801preview:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20231101preview:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20240101:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20240215preview:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20240401:Cluster").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20240901preview:Cluster").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Cluster get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Cluster(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy