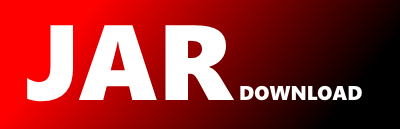
com.pulumi.azurenative.azurestackhci.SecurityRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.azurestackhci;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.azurestackhci.SecurityRuleArgs;
import com.pulumi.azurenative.azurestackhci.outputs.ExtendedLocationResponse;
import com.pulumi.azurenative.azurestackhci.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Security Rule resource.
* Azure REST API version: 2024-02-01-preview.
*
* Other available API versions: 2024-05-01-preview, 2024-07-15-preview, 2024-08-01-preview.
*
* ## Example Usage
* ### SecurityRulesCreateOrUpdate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.azurestackhci.SecurityRule;
* import com.pulumi.azurenative.azurestackhci.SecurityRuleArgs;
* import com.pulumi.azurenative.azurestackhci.inputs.ExtendedLocationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var securityRule = new SecurityRule("securityRule", SecurityRuleArgs.builder()
* .access("Allow")
* .destinationAddressPrefixes("*")
* .destinationPortRanges("80")
* .direction("Inbound")
* .extendedLocation(ExtendedLocationArgs.builder()
* .name("/subscriptions/fd3c3665-1729-4b7b-9a38-238e83b0f98b/resourceGroups/testrg/providers/Microsoft.ExtendedLocation/customLocations/dogfood-location")
* .type("CustomLocation")
* .build())
* .networkSecurityGroupName("testnsg")
* .priority(130)
* .protocol("*")
* .resourceGroupName("testrg")
* .securityRuleName("rule1")
* .sourceAddressPrefixes("*")
* .sourcePortRanges("*")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:azurestackhci:SecurityRule rule1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.AzureStackHCI/networkSecurityGroups/{networkSecurityGroupName}/securityRules/{securityRuleName}
* ```
*
*/
@ResourceType(type="azure-native:azurestackhci:SecurityRule")
public class SecurityRule extends com.pulumi.resources.CustomResource {
/**
* The network traffic is allowed or denied.
*
*/
@Export(name="access", refs={String.class}, tree="[0]")
private Output access;
/**
* @return The network traffic is allowed or denied.
*
*/
public Output access() {
return this.access;
}
/**
* A description for this rule. Restricted to 140 chars.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return A description for this rule. Restricted to 140 chars.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* The destination address prefixes. CIDR or destination IP ranges.
*
*/
@Export(name="destinationAddressPrefixes", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> destinationAddressPrefixes;
/**
* @return The destination address prefixes. CIDR or destination IP ranges.
*
*/
public Output>> destinationAddressPrefixes() {
return Codegen.optional(this.destinationAddressPrefixes);
}
/**
* The destination port ranges. Integer or range between 0 and 65535. Asterisk '*' can also be used to match all ports.
*
*/
@Export(name="destinationPortRanges", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> destinationPortRanges;
/**
* @return The destination port ranges. Integer or range between 0 and 65535. Asterisk '*' can also be used to match all ports.
*
*/
public Output>> destinationPortRanges() {
return Codegen.optional(this.destinationPortRanges);
}
/**
* The direction of the rule. The direction specifies if rule will be evaluated on incoming or outgoing traffic.
*
*/
@Export(name="direction", refs={String.class}, tree="[0]")
private Output direction;
/**
* @return The direction of the rule. The direction specifies if rule will be evaluated on incoming or outgoing traffic.
*
*/
public Output direction() {
return this.direction;
}
/**
* The extendedLocation of the resource.
*
*/
@Export(name="extendedLocation", refs={ExtendedLocationResponse.class}, tree="[0]")
private Output* @Nullable */ ExtendedLocationResponse> extendedLocation;
/**
* @return The extendedLocation of the resource.
*
*/
public Output> extendedLocation() {
return Codegen.optional(this.extendedLocation);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* The priority of the rule. The value can be between 100 and 4096. The priority number must be unique for each rule in the collection. The lower the priority number, the higher the priority of the rule.
*
*/
@Export(name="priority", refs={Integer.class}, tree="[0]")
private Output priority;
/**
* @return The priority of the rule. The value can be between 100 and 4096. The priority number must be unique for each rule in the collection. The lower the priority number, the higher the priority of the rule.
*
*/
public Output priority() {
return this.priority;
}
/**
* Network protocol this rule applies to.
*
*/
@Export(name="protocol", refs={String.class}, tree="[0]")
private Output protocol;
/**
* @return Network protocol this rule applies to.
*
*/
public Output protocol() {
return this.protocol;
}
/**
* Provisioning state of the SR
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Provisioning state of the SR
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* The CIDR or source IP ranges.
*
*/
@Export(name="sourceAddressPrefixes", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> sourceAddressPrefixes;
/**
* @return The CIDR or source IP ranges.
*
*/
public Output>> sourceAddressPrefixes() {
return Codegen.optional(this.sourceAddressPrefixes);
}
/**
* The source port ranges. Integer or range between 0 and 65535. Asterisk '*' can also be used to match all ports.
*
*/
@Export(name="sourcePortRanges", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> sourcePortRanges;
/**
* @return The source port ranges. Integer or range between 0 and 65535. Asterisk '*' can also be used to match all ports.
*
*/
public Output>> sourcePortRanges() {
return Codegen.optional(this.sourcePortRanges);
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public SecurityRule(java.lang.String name) {
this(name, SecurityRuleArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public SecurityRule(java.lang.String name, SecurityRuleArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public SecurityRule(java.lang.String name, SecurityRuleArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:azurestackhci:SecurityRule", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private SecurityRule(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:azurestackhci:SecurityRule", name, null, makeResourceOptions(options, id), false);
}
private static SecurityRuleArgs makeArgs(SecurityRuleArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? SecurityRuleArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:azurestackhci/v20240201preview:SecurityRule").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20240501preview:SecurityRule").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20240715preview:SecurityRule").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20240801preview:SecurityRule").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static SecurityRule get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new SecurityRule(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy