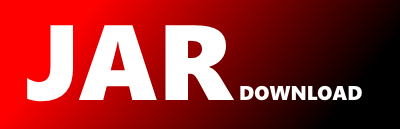
com.pulumi.azurenative.batch.BatchFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.batch;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.batch.inputs.GetApplicationArgs;
import com.pulumi.azurenative.batch.inputs.GetApplicationPackageArgs;
import com.pulumi.azurenative.batch.inputs.GetApplicationPackagePlainArgs;
import com.pulumi.azurenative.batch.inputs.GetApplicationPlainArgs;
import com.pulumi.azurenative.batch.inputs.GetBatchAccountArgs;
import com.pulumi.azurenative.batch.inputs.GetBatchAccountPlainArgs;
import com.pulumi.azurenative.batch.inputs.GetPoolArgs;
import com.pulumi.azurenative.batch.inputs.GetPoolPlainArgs;
import com.pulumi.azurenative.batch.inputs.ListBatchAccountKeysArgs;
import com.pulumi.azurenative.batch.inputs.ListBatchAccountKeysPlainArgs;
import com.pulumi.azurenative.batch.outputs.GetApplicationPackageResult;
import com.pulumi.azurenative.batch.outputs.GetApplicationResult;
import com.pulumi.azurenative.batch.outputs.GetBatchAccountResult;
import com.pulumi.azurenative.batch.outputs.GetPoolResult;
import com.pulumi.azurenative.batch.outputs.ListBatchAccountKeysResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class BatchFunctions {
/**
* Gets information about the specified application.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2017-09-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static Output getApplication(GetApplicationArgs args) {
return getApplication(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified application.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2017-09-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static CompletableFuture getApplicationPlain(GetApplicationPlainArgs args) {
return getApplicationPlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified application.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2017-09-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static Output getApplication(GetApplicationArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:batch:getApplication", TypeShape.of(GetApplicationResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified application.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2017-09-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static CompletableFuture getApplicationPlain(GetApplicationPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:batch:getApplication", TypeShape.of(GetApplicationResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified application package.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2017-09-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static Output getApplicationPackage(GetApplicationPackageArgs args) {
return getApplicationPackage(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified application package.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2017-09-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static CompletableFuture getApplicationPackagePlain(GetApplicationPackagePlainArgs args) {
return getApplicationPackagePlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified application package.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2017-09-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static Output getApplicationPackage(GetApplicationPackageArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:batch:getApplicationPackage", TypeShape.of(GetApplicationPackageResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified application package.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2017-09-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static CompletableFuture getApplicationPackagePlain(GetApplicationPackagePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:batch:getApplicationPackage", TypeShape.of(GetApplicationPackageResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified Batch account.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2017-01-01, 2022-01-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static Output getBatchAccount(GetBatchAccountArgs args) {
return getBatchAccount(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified Batch account.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2017-01-01, 2022-01-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static CompletableFuture getBatchAccountPlain(GetBatchAccountPlainArgs args) {
return getBatchAccountPlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified Batch account.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2017-01-01, 2022-01-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static Output getBatchAccount(GetBatchAccountArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:batch:getBatchAccount", TypeShape.of(GetBatchAccountResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified Batch account.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2017-01-01, 2022-01-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static CompletableFuture getBatchAccountPlain(GetBatchAccountPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:batch:getBatchAccount", TypeShape.of(GetBatchAccountResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified pool.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-05-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static Output getPool(GetPoolArgs args) {
return getPool(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified pool.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-05-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static CompletableFuture getPoolPlain(GetPoolPlainArgs args) {
return getPoolPlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified pool.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-05-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static Output getPool(GetPoolArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:batch:getPool", TypeShape.of(GetPoolResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified pool.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-05-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static CompletableFuture getPoolPlain(GetPoolPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:batch:getPool", TypeShape.of(GetPoolResult.class), args, Utilities.withVersion(options));
}
/**
* This operation applies only to Batch accounts with allowedAuthenticationModes containing 'SharedKey'. If the Batch account doesn't contain 'SharedKey' in its allowedAuthenticationMode, clients cannot use shared keys to authenticate, and must use another allowedAuthenticationModes instead. In this case, getting the keys will fail.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2017-01-01, 2022-01-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static Output listBatchAccountKeys(ListBatchAccountKeysArgs args) {
return listBatchAccountKeys(args, InvokeOptions.Empty);
}
/**
* This operation applies only to Batch accounts with allowedAuthenticationModes containing 'SharedKey'. If the Batch account doesn't contain 'SharedKey' in its allowedAuthenticationMode, clients cannot use shared keys to authenticate, and must use another allowedAuthenticationModes instead. In this case, getting the keys will fail.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2017-01-01, 2022-01-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static CompletableFuture listBatchAccountKeysPlain(ListBatchAccountKeysPlainArgs args) {
return listBatchAccountKeysPlain(args, InvokeOptions.Empty);
}
/**
* This operation applies only to Batch accounts with allowedAuthenticationModes containing 'SharedKey'. If the Batch account doesn't contain 'SharedKey' in its allowedAuthenticationMode, clients cannot use shared keys to authenticate, and must use another allowedAuthenticationModes instead. In this case, getting the keys will fail.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2017-01-01, 2022-01-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static Output listBatchAccountKeys(ListBatchAccountKeysArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:batch:listBatchAccountKeys", TypeShape.of(ListBatchAccountKeysResult.class), args, Utilities.withVersion(options));
}
/**
* This operation applies only to Batch accounts with allowedAuthenticationModes containing 'SharedKey'. If the Batch account doesn't contain 'SharedKey' in its allowedAuthenticationMode, clients cannot use shared keys to authenticate, and must use another allowedAuthenticationModes instead. In this case, getting the keys will fail.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2017-01-01, 2022-01-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
*/
public static CompletableFuture listBatchAccountKeysPlain(ListBatchAccountKeysPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:batch:listBatchAccountKeys", TypeShape.of(ListBatchAccountKeysResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy