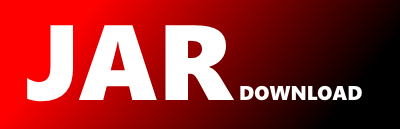
com.pulumi.azurenative.botservice.BotserviceFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.botservice;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.botservice.inputs.GetBotArgs;
import com.pulumi.azurenative.botservice.inputs.GetBotConnectionArgs;
import com.pulumi.azurenative.botservice.inputs.GetBotConnectionPlainArgs;
import com.pulumi.azurenative.botservice.inputs.GetBotPlainArgs;
import com.pulumi.azurenative.botservice.inputs.GetChannelArgs;
import com.pulumi.azurenative.botservice.inputs.GetChannelPlainArgs;
import com.pulumi.azurenative.botservice.inputs.GetPrivateEndpointConnectionArgs;
import com.pulumi.azurenative.botservice.inputs.GetPrivateEndpointConnectionPlainArgs;
import com.pulumi.azurenative.botservice.inputs.ListBotConnectionServiceProvidersArgs;
import com.pulumi.azurenative.botservice.inputs.ListBotConnectionServiceProvidersPlainArgs;
import com.pulumi.azurenative.botservice.inputs.ListBotConnectionWithSecretsArgs;
import com.pulumi.azurenative.botservice.inputs.ListBotConnectionWithSecretsPlainArgs;
import com.pulumi.azurenative.botservice.inputs.ListChannelWithKeysArgs;
import com.pulumi.azurenative.botservice.inputs.ListChannelWithKeysPlainArgs;
import com.pulumi.azurenative.botservice.inputs.ListQnAMakerEndpointKeyArgs;
import com.pulumi.azurenative.botservice.inputs.ListQnAMakerEndpointKeyPlainArgs;
import com.pulumi.azurenative.botservice.outputs.GetBotConnectionResult;
import com.pulumi.azurenative.botservice.outputs.GetBotResult;
import com.pulumi.azurenative.botservice.outputs.GetChannelResult;
import com.pulumi.azurenative.botservice.outputs.GetPrivateEndpointConnectionResult;
import com.pulumi.azurenative.botservice.outputs.ListBotConnectionServiceProvidersResult;
import com.pulumi.azurenative.botservice.outputs.ListBotConnectionWithSecretsResult;
import com.pulumi.azurenative.botservice.outputs.ListChannelWithKeysResult;
import com.pulumi.azurenative.botservice.outputs.ListQnAMakerEndpointKeyResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class BotserviceFunctions {
/**
* Returns a BotService specified by the parameters.
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static Output getBot(GetBotArgs args) {
return getBot(args, InvokeOptions.Empty);
}
/**
* Returns a BotService specified by the parameters.
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static CompletableFuture getBotPlain(GetBotPlainArgs args) {
return getBotPlain(args, InvokeOptions.Empty);
}
/**
* Returns a BotService specified by the parameters.
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static Output getBot(GetBotArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:botservice:getBot", TypeShape.of(GetBotResult.class), args, Utilities.withVersion(options));
}
/**
* Returns a BotService specified by the parameters.
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static CompletableFuture getBotPlain(GetBotPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:botservice:getBot", TypeShape.of(GetBotResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Connection Setting registration for a Bot Service
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static Output getBotConnection(GetBotConnectionArgs args) {
return getBotConnection(args, InvokeOptions.Empty);
}
/**
* Get a Connection Setting registration for a Bot Service
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static CompletableFuture getBotConnectionPlain(GetBotConnectionPlainArgs args) {
return getBotConnectionPlain(args, InvokeOptions.Empty);
}
/**
* Get a Connection Setting registration for a Bot Service
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static Output getBotConnection(GetBotConnectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:botservice:getBotConnection", TypeShape.of(GetBotConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Connection Setting registration for a Bot Service
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static CompletableFuture getBotConnectionPlain(GetBotConnectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:botservice:getBotConnection", TypeShape.of(GetBotConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Returns a BotService Channel registration specified by the parameters.
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static Output getChannel(GetChannelArgs args) {
return getChannel(args, InvokeOptions.Empty);
}
/**
* Returns a BotService Channel registration specified by the parameters.
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static CompletableFuture getChannelPlain(GetChannelPlainArgs args) {
return getChannelPlain(args, InvokeOptions.Empty);
}
/**
* Returns a BotService Channel registration specified by the parameters.
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static Output getChannel(GetChannelArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:botservice:getChannel", TypeShape.of(GetChannelResult.class), args, Utilities.withVersion(options));
}
/**
* Returns a BotService Channel registration specified by the parameters.
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static CompletableFuture getChannelPlain(GetChannelPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:botservice:getChannel", TypeShape.of(GetChannelResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified private endpoint connection associated with the Bot.
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args) {
return getPrivateEndpointConnection(args, InvokeOptions.Empty);
}
/**
* Gets the specified private endpoint connection associated with the Bot.
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args) {
return getPrivateEndpointConnectionPlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified private endpoint connection associated with the Bot.
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:botservice:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified private endpoint connection associated with the Bot.
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:botservice:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Lists the available Service Providers for creating Connection Settings
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2017-12-01, 2018-07-12, 2020-06-02, 2021-03-01, 2021-05-01-preview, 2022-06-15-preview, 2023-09-15-preview.
*
*/
public static Output listBotConnectionServiceProviders() {
return listBotConnectionServiceProviders(ListBotConnectionServiceProvidersArgs.Empty, InvokeOptions.Empty);
}
/**
* Lists the available Service Providers for creating Connection Settings
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2017-12-01, 2018-07-12, 2020-06-02, 2021-03-01, 2021-05-01-preview, 2022-06-15-preview, 2023-09-15-preview.
*
*/
public static CompletableFuture listBotConnectionServiceProvidersPlain() {
return listBotConnectionServiceProvidersPlain(ListBotConnectionServiceProvidersPlainArgs.Empty, InvokeOptions.Empty);
}
/**
* Lists the available Service Providers for creating Connection Settings
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2017-12-01, 2018-07-12, 2020-06-02, 2021-03-01, 2021-05-01-preview, 2022-06-15-preview, 2023-09-15-preview.
*
*/
public static Output listBotConnectionServiceProviders(ListBotConnectionServiceProvidersArgs args) {
return listBotConnectionServiceProviders(args, InvokeOptions.Empty);
}
/**
* Lists the available Service Providers for creating Connection Settings
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2017-12-01, 2018-07-12, 2020-06-02, 2021-03-01, 2021-05-01-preview, 2022-06-15-preview, 2023-09-15-preview.
*
*/
public static CompletableFuture listBotConnectionServiceProvidersPlain(ListBotConnectionServiceProvidersPlainArgs args) {
return listBotConnectionServiceProvidersPlain(args, InvokeOptions.Empty);
}
/**
* Lists the available Service Providers for creating Connection Settings
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2017-12-01, 2018-07-12, 2020-06-02, 2021-03-01, 2021-05-01-preview, 2022-06-15-preview, 2023-09-15-preview.
*
*/
public static Output listBotConnectionServiceProviders(ListBotConnectionServiceProvidersArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:botservice:listBotConnectionServiceProviders", TypeShape.of(ListBotConnectionServiceProvidersResult.class), args, Utilities.withVersion(options));
}
/**
* Lists the available Service Providers for creating Connection Settings
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2017-12-01, 2018-07-12, 2020-06-02, 2021-03-01, 2021-05-01-preview, 2022-06-15-preview, 2023-09-15-preview.
*
*/
public static CompletableFuture listBotConnectionServiceProvidersPlain(ListBotConnectionServiceProvidersPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:botservice:listBotConnectionServiceProviders", TypeShape.of(ListBotConnectionServiceProvidersResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Connection Setting registration for a Bot Service
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static Output listBotConnectionWithSecrets(ListBotConnectionWithSecretsArgs args) {
return listBotConnectionWithSecrets(args, InvokeOptions.Empty);
}
/**
* Get a Connection Setting registration for a Bot Service
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static CompletableFuture listBotConnectionWithSecretsPlain(ListBotConnectionWithSecretsPlainArgs args) {
return listBotConnectionWithSecretsPlain(args, InvokeOptions.Empty);
}
/**
* Get a Connection Setting registration for a Bot Service
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static Output listBotConnectionWithSecrets(ListBotConnectionWithSecretsArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:botservice:listBotConnectionWithSecrets", TypeShape.of(ListBotConnectionWithSecretsResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Connection Setting registration for a Bot Service
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static CompletableFuture listBotConnectionWithSecretsPlain(ListBotConnectionWithSecretsPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:botservice:listBotConnectionWithSecrets", TypeShape.of(ListBotConnectionWithSecretsResult.class), args, Utilities.withVersion(options));
}
/**
* Lists a Channel registration for a Bot Service including secrets
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static Output listChannelWithKeys(ListChannelWithKeysArgs args) {
return listChannelWithKeys(args, InvokeOptions.Empty);
}
/**
* Lists a Channel registration for a Bot Service including secrets
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static CompletableFuture listChannelWithKeysPlain(ListChannelWithKeysPlainArgs args) {
return listChannelWithKeysPlain(args, InvokeOptions.Empty);
}
/**
* Lists a Channel registration for a Bot Service including secrets
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static Output listChannelWithKeys(ListChannelWithKeysArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:botservice:listChannelWithKeys", TypeShape.of(ListChannelWithKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Lists a Channel registration for a Bot Service including secrets
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2023-09-15-preview.
*
*/
public static CompletableFuture listChannelWithKeysPlain(ListChannelWithKeysPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:botservice:listChannelWithKeys", TypeShape.of(ListChannelWithKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Lists the QnA Maker endpoint keys
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2022-06-15-preview, 2023-09-15-preview.
*
*/
public static Output listQnAMakerEndpointKey() {
return listQnAMakerEndpointKey(ListQnAMakerEndpointKeyArgs.Empty, InvokeOptions.Empty);
}
/**
* Lists the QnA Maker endpoint keys
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2022-06-15-preview, 2023-09-15-preview.
*
*/
public static CompletableFuture listQnAMakerEndpointKeyPlain() {
return listQnAMakerEndpointKeyPlain(ListQnAMakerEndpointKeyPlainArgs.Empty, InvokeOptions.Empty);
}
/**
* Lists the QnA Maker endpoint keys
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2022-06-15-preview, 2023-09-15-preview.
*
*/
public static Output listQnAMakerEndpointKey(ListQnAMakerEndpointKeyArgs args) {
return listQnAMakerEndpointKey(args, InvokeOptions.Empty);
}
/**
* Lists the QnA Maker endpoint keys
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2022-06-15-preview, 2023-09-15-preview.
*
*/
public static CompletableFuture listQnAMakerEndpointKeyPlain(ListQnAMakerEndpointKeyPlainArgs args) {
return listQnAMakerEndpointKeyPlain(args, InvokeOptions.Empty);
}
/**
* Lists the QnA Maker endpoint keys
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2022-06-15-preview, 2023-09-15-preview.
*
*/
public static Output listQnAMakerEndpointKey(ListQnAMakerEndpointKeyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:botservice:listQnAMakerEndpointKey", TypeShape.of(ListQnAMakerEndpointKeyResult.class), args, Utilities.withVersion(options));
}
/**
* Lists the QnA Maker endpoint keys
* Azure REST API version: 2022-09-15.
*
* Other available API versions: 2022-06-15-preview, 2023-09-15-preview.
*
*/
public static CompletableFuture listQnAMakerEndpointKeyPlain(ListQnAMakerEndpointKeyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:botservice:listQnAMakerEndpointKey", TypeShape.of(ListQnAMakerEndpointKeyResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy