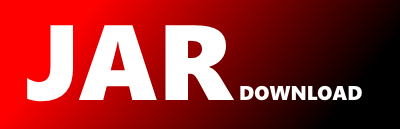
com.pulumi.azurenative.botservice.inputs.BotPropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.botservice.inputs;
import com.pulumi.azurenative.botservice.enums.MsaAppType;
import com.pulumi.azurenative.botservice.enums.PublicNetworkAccess;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The parameters to provide for the Bot.
*
*/
public final class BotPropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final BotPropertiesArgs Empty = new BotPropertiesArgs();
/**
* Contains resource all settings defined as key/value pairs.
*
*/
@Import(name="allSettings")
private @Nullable Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy