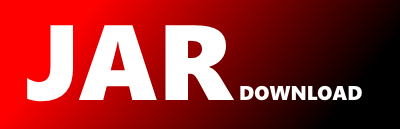
com.pulumi.azurenative.botservice.outputs.BotPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.botservice.outputs;
import com.pulumi.azurenative.botservice.outputs.PrivateEndpointConnectionResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class BotPropertiesResponse {
/**
* @return Contains resource all settings defined as key/value pairs.
*
*/
private @Nullable Map allSettings;
/**
* @return The hint (e.g. keyVault secret resourceId) on how to fetch the app secret
*
*/
private @Nullable String appPasswordHint;
/**
* @return The CMK encryption status
*
*/
private String cmekEncryptionStatus;
/**
* @return The CMK Url
*
*/
private @Nullable String cmekKeyVaultUrl;
/**
* @return Collection of channels for which the bot is configured
*
*/
private List configuredChannels;
/**
* @return The description of the bot
*
*/
private @Nullable String description;
/**
* @return The Application Insights key
*
*/
private @Nullable String developerAppInsightKey;
/**
* @return The Application Insights Api Key
*
*/
private @Nullable String developerAppInsightsApiKey;
/**
* @return The Application Insights App Id
*
*/
private @Nullable String developerAppInsightsApplicationId;
/**
* @return Opt-out of local authentication and ensure only MSI and AAD can be used exclusively for authentication.
*
*/
private @Nullable Boolean disableLocalAuth;
/**
* @return The Name of the bot
*
*/
private String displayName;
/**
* @return Collection of channels for which the bot is enabled
*
*/
private List enabledChannels;
/**
* @return The bot's endpoint
*
*/
private String endpoint;
/**
* @return The bot's endpoint version
*
*/
private String endpointVersion;
/**
* @return The Icon Url of the bot
*
*/
private @Nullable String iconUrl;
/**
* @return Whether Cmek is enabled
*
*/
private @Nullable Boolean isCmekEnabled;
/**
* @return Whether the bot is developerAppInsightsApiKey set
*
*/
private Boolean isDeveloperAppInsightsApiKeySet;
/**
* @return Whether the bot is streaming supported
*
*/
private @Nullable Boolean isStreamingSupported;
/**
* @return Collection of LUIS App Ids
*
*/
private @Nullable List luisAppIds;
/**
* @return The LUIS Key
*
*/
private @Nullable String luisKey;
/**
* @return The bot's manifest url
*
*/
private @Nullable String manifestUrl;
/**
* @return Token used to migrate non Azure bot to azure subscription
*
*/
private String migrationToken;
/**
* @return Microsoft App Id for the bot
*
*/
private String msaAppId;
/**
* @return Microsoft App Managed Identity Resource Id for the bot
*
*/
private @Nullable String msaAppMSIResourceId;
/**
* @return Microsoft App Tenant Id for the bot
*
*/
private @Nullable String msaAppTenantId;
/**
* @return Microsoft App Type for the bot
*
*/
private @Nullable String msaAppType;
/**
* @return The hint to browser (e.g. protocol handler) on how to open the bot for authoring
*
*/
private @Nullable String openWithHint;
/**
* @return Contains resource parameters defined as key/value pairs.
*
*/
private @Nullable Map parameters;
/**
* @return List of Private Endpoint Connections configured for the bot
*
*/
private List privateEndpointConnections;
/**
* @return Provisioning state of the resource
*
*/
private String provisioningState;
/**
* @return Whether the bot is in an isolated network
*
*/
private @Nullable String publicNetworkAccess;
/**
* @return Publishing credentials of the resource
*
*/
private @Nullable String publishingCredentials;
/**
* @return The channel schema transformation version for the bot
*
*/
private @Nullable String schemaTransformationVersion;
/**
* @return The storage resourceId for the bot
*
*/
private @Nullable String storageResourceId;
/**
* @return The Tenant Id for the bot
*
*/
private @Nullable String tenantId;
private BotPropertiesResponse() {}
/**
* @return Contains resource all settings defined as key/value pairs.
*
*/
public Map allSettings() {
return this.allSettings == null ? Map.of() : this.allSettings;
}
/**
* @return The hint (e.g. keyVault secret resourceId) on how to fetch the app secret
*
*/
public Optional appPasswordHint() {
return Optional.ofNullable(this.appPasswordHint);
}
/**
* @return The CMK encryption status
*
*/
public String cmekEncryptionStatus() {
return this.cmekEncryptionStatus;
}
/**
* @return The CMK Url
*
*/
public Optional cmekKeyVaultUrl() {
return Optional.ofNullable(this.cmekKeyVaultUrl);
}
/**
* @return Collection of channels for which the bot is configured
*
*/
public List configuredChannels() {
return this.configuredChannels;
}
/**
* @return The description of the bot
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return The Application Insights key
*
*/
public Optional developerAppInsightKey() {
return Optional.ofNullable(this.developerAppInsightKey);
}
/**
* @return The Application Insights Api Key
*
*/
public Optional developerAppInsightsApiKey() {
return Optional.ofNullable(this.developerAppInsightsApiKey);
}
/**
* @return The Application Insights App Id
*
*/
public Optional developerAppInsightsApplicationId() {
return Optional.ofNullable(this.developerAppInsightsApplicationId);
}
/**
* @return Opt-out of local authentication and ensure only MSI and AAD can be used exclusively for authentication.
*
*/
public Optional disableLocalAuth() {
return Optional.ofNullable(this.disableLocalAuth);
}
/**
* @return The Name of the bot
*
*/
public String displayName() {
return this.displayName;
}
/**
* @return Collection of channels for which the bot is enabled
*
*/
public List enabledChannels() {
return this.enabledChannels;
}
/**
* @return The bot's endpoint
*
*/
public String endpoint() {
return this.endpoint;
}
/**
* @return The bot's endpoint version
*
*/
public String endpointVersion() {
return this.endpointVersion;
}
/**
* @return The Icon Url of the bot
*
*/
public Optional iconUrl() {
return Optional.ofNullable(this.iconUrl);
}
/**
* @return Whether Cmek is enabled
*
*/
public Optional isCmekEnabled() {
return Optional.ofNullable(this.isCmekEnabled);
}
/**
* @return Whether the bot is developerAppInsightsApiKey set
*
*/
public Boolean isDeveloperAppInsightsApiKeySet() {
return this.isDeveloperAppInsightsApiKeySet;
}
/**
* @return Whether the bot is streaming supported
*
*/
public Optional isStreamingSupported() {
return Optional.ofNullable(this.isStreamingSupported);
}
/**
* @return Collection of LUIS App Ids
*
*/
public List luisAppIds() {
return this.luisAppIds == null ? List.of() : this.luisAppIds;
}
/**
* @return The LUIS Key
*
*/
public Optional luisKey() {
return Optional.ofNullable(this.luisKey);
}
/**
* @return The bot's manifest url
*
*/
public Optional manifestUrl() {
return Optional.ofNullable(this.manifestUrl);
}
/**
* @return Token used to migrate non Azure bot to azure subscription
*
*/
public String migrationToken() {
return this.migrationToken;
}
/**
* @return Microsoft App Id for the bot
*
*/
public String msaAppId() {
return this.msaAppId;
}
/**
* @return Microsoft App Managed Identity Resource Id for the bot
*
*/
public Optional msaAppMSIResourceId() {
return Optional.ofNullable(this.msaAppMSIResourceId);
}
/**
* @return Microsoft App Tenant Id for the bot
*
*/
public Optional msaAppTenantId() {
return Optional.ofNullable(this.msaAppTenantId);
}
/**
* @return Microsoft App Type for the bot
*
*/
public Optional msaAppType() {
return Optional.ofNullable(this.msaAppType);
}
/**
* @return The hint to browser (e.g. protocol handler) on how to open the bot for authoring
*
*/
public Optional openWithHint() {
return Optional.ofNullable(this.openWithHint);
}
/**
* @return Contains resource parameters defined as key/value pairs.
*
*/
public Map parameters() {
return this.parameters == null ? Map.of() : this.parameters;
}
/**
* @return List of Private Endpoint Connections configured for the bot
*
*/
public List privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* @return Provisioning state of the resource
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Whether the bot is in an isolated network
*
*/
public Optional publicNetworkAccess() {
return Optional.ofNullable(this.publicNetworkAccess);
}
/**
* @return Publishing credentials of the resource
*
*/
public Optional publishingCredentials() {
return Optional.ofNullable(this.publishingCredentials);
}
/**
* @return The channel schema transformation version for the bot
*
*/
public Optional schemaTransformationVersion() {
return Optional.ofNullable(this.schemaTransformationVersion);
}
/**
* @return The storage resourceId for the bot
*
*/
public Optional storageResourceId() {
return Optional.ofNullable(this.storageResourceId);
}
/**
* @return The Tenant Id for the bot
*
*/
public Optional tenantId() {
return Optional.ofNullable(this.tenantId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(BotPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Map allSettings;
private @Nullable String appPasswordHint;
private String cmekEncryptionStatus;
private @Nullable String cmekKeyVaultUrl;
private List configuredChannels;
private @Nullable String description;
private @Nullable String developerAppInsightKey;
private @Nullable String developerAppInsightsApiKey;
private @Nullable String developerAppInsightsApplicationId;
private @Nullable Boolean disableLocalAuth;
private String displayName;
private List enabledChannels;
private String endpoint;
private String endpointVersion;
private @Nullable String iconUrl;
private @Nullable Boolean isCmekEnabled;
private Boolean isDeveloperAppInsightsApiKeySet;
private @Nullable Boolean isStreamingSupported;
private @Nullable List luisAppIds;
private @Nullable String luisKey;
private @Nullable String manifestUrl;
private String migrationToken;
private String msaAppId;
private @Nullable String msaAppMSIResourceId;
private @Nullable String msaAppTenantId;
private @Nullable String msaAppType;
private @Nullable String openWithHint;
private @Nullable Map parameters;
private List privateEndpointConnections;
private String provisioningState;
private @Nullable String publicNetworkAccess;
private @Nullable String publishingCredentials;
private @Nullable String schemaTransformationVersion;
private @Nullable String storageResourceId;
private @Nullable String tenantId;
public Builder() {}
public Builder(BotPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.allSettings = defaults.allSettings;
this.appPasswordHint = defaults.appPasswordHint;
this.cmekEncryptionStatus = defaults.cmekEncryptionStatus;
this.cmekKeyVaultUrl = defaults.cmekKeyVaultUrl;
this.configuredChannels = defaults.configuredChannels;
this.description = defaults.description;
this.developerAppInsightKey = defaults.developerAppInsightKey;
this.developerAppInsightsApiKey = defaults.developerAppInsightsApiKey;
this.developerAppInsightsApplicationId = defaults.developerAppInsightsApplicationId;
this.disableLocalAuth = defaults.disableLocalAuth;
this.displayName = defaults.displayName;
this.enabledChannels = defaults.enabledChannels;
this.endpoint = defaults.endpoint;
this.endpointVersion = defaults.endpointVersion;
this.iconUrl = defaults.iconUrl;
this.isCmekEnabled = defaults.isCmekEnabled;
this.isDeveloperAppInsightsApiKeySet = defaults.isDeveloperAppInsightsApiKeySet;
this.isStreamingSupported = defaults.isStreamingSupported;
this.luisAppIds = defaults.luisAppIds;
this.luisKey = defaults.luisKey;
this.manifestUrl = defaults.manifestUrl;
this.migrationToken = defaults.migrationToken;
this.msaAppId = defaults.msaAppId;
this.msaAppMSIResourceId = defaults.msaAppMSIResourceId;
this.msaAppTenantId = defaults.msaAppTenantId;
this.msaAppType = defaults.msaAppType;
this.openWithHint = defaults.openWithHint;
this.parameters = defaults.parameters;
this.privateEndpointConnections = defaults.privateEndpointConnections;
this.provisioningState = defaults.provisioningState;
this.publicNetworkAccess = defaults.publicNetworkAccess;
this.publishingCredentials = defaults.publishingCredentials;
this.schemaTransformationVersion = defaults.schemaTransformationVersion;
this.storageResourceId = defaults.storageResourceId;
this.tenantId = defaults.tenantId;
}
@CustomType.Setter
public Builder allSettings(@Nullable Map allSettings) {
this.allSettings = allSettings;
return this;
}
@CustomType.Setter
public Builder appPasswordHint(@Nullable String appPasswordHint) {
this.appPasswordHint = appPasswordHint;
return this;
}
@CustomType.Setter
public Builder cmekEncryptionStatus(String cmekEncryptionStatus) {
if (cmekEncryptionStatus == null) {
throw new MissingRequiredPropertyException("BotPropertiesResponse", "cmekEncryptionStatus");
}
this.cmekEncryptionStatus = cmekEncryptionStatus;
return this;
}
@CustomType.Setter
public Builder cmekKeyVaultUrl(@Nullable String cmekKeyVaultUrl) {
this.cmekKeyVaultUrl = cmekKeyVaultUrl;
return this;
}
@CustomType.Setter
public Builder configuredChannels(List configuredChannels) {
if (configuredChannels == null) {
throw new MissingRequiredPropertyException("BotPropertiesResponse", "configuredChannels");
}
this.configuredChannels = configuredChannels;
return this;
}
public Builder configuredChannels(String... configuredChannels) {
return configuredChannels(List.of(configuredChannels));
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder developerAppInsightKey(@Nullable String developerAppInsightKey) {
this.developerAppInsightKey = developerAppInsightKey;
return this;
}
@CustomType.Setter
public Builder developerAppInsightsApiKey(@Nullable String developerAppInsightsApiKey) {
this.developerAppInsightsApiKey = developerAppInsightsApiKey;
return this;
}
@CustomType.Setter
public Builder developerAppInsightsApplicationId(@Nullable String developerAppInsightsApplicationId) {
this.developerAppInsightsApplicationId = developerAppInsightsApplicationId;
return this;
}
@CustomType.Setter
public Builder disableLocalAuth(@Nullable Boolean disableLocalAuth) {
this.disableLocalAuth = disableLocalAuth;
return this;
}
@CustomType.Setter
public Builder displayName(String displayName) {
if (displayName == null) {
throw new MissingRequiredPropertyException("BotPropertiesResponse", "displayName");
}
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder enabledChannels(List enabledChannels) {
if (enabledChannels == null) {
throw new MissingRequiredPropertyException("BotPropertiesResponse", "enabledChannels");
}
this.enabledChannels = enabledChannels;
return this;
}
public Builder enabledChannels(String... enabledChannels) {
return enabledChannels(List.of(enabledChannels));
}
@CustomType.Setter
public Builder endpoint(String endpoint) {
if (endpoint == null) {
throw new MissingRequiredPropertyException("BotPropertiesResponse", "endpoint");
}
this.endpoint = endpoint;
return this;
}
@CustomType.Setter
public Builder endpointVersion(String endpointVersion) {
if (endpointVersion == null) {
throw new MissingRequiredPropertyException("BotPropertiesResponse", "endpointVersion");
}
this.endpointVersion = endpointVersion;
return this;
}
@CustomType.Setter
public Builder iconUrl(@Nullable String iconUrl) {
this.iconUrl = iconUrl;
return this;
}
@CustomType.Setter
public Builder isCmekEnabled(@Nullable Boolean isCmekEnabled) {
this.isCmekEnabled = isCmekEnabled;
return this;
}
@CustomType.Setter
public Builder isDeveloperAppInsightsApiKeySet(Boolean isDeveloperAppInsightsApiKeySet) {
if (isDeveloperAppInsightsApiKeySet == null) {
throw new MissingRequiredPropertyException("BotPropertiesResponse", "isDeveloperAppInsightsApiKeySet");
}
this.isDeveloperAppInsightsApiKeySet = isDeveloperAppInsightsApiKeySet;
return this;
}
@CustomType.Setter
public Builder isStreamingSupported(@Nullable Boolean isStreamingSupported) {
this.isStreamingSupported = isStreamingSupported;
return this;
}
@CustomType.Setter
public Builder luisAppIds(@Nullable List luisAppIds) {
this.luisAppIds = luisAppIds;
return this;
}
public Builder luisAppIds(String... luisAppIds) {
return luisAppIds(List.of(luisAppIds));
}
@CustomType.Setter
public Builder luisKey(@Nullable String luisKey) {
this.luisKey = luisKey;
return this;
}
@CustomType.Setter
public Builder manifestUrl(@Nullable String manifestUrl) {
this.manifestUrl = manifestUrl;
return this;
}
@CustomType.Setter
public Builder migrationToken(String migrationToken) {
if (migrationToken == null) {
throw new MissingRequiredPropertyException("BotPropertiesResponse", "migrationToken");
}
this.migrationToken = migrationToken;
return this;
}
@CustomType.Setter
public Builder msaAppId(String msaAppId) {
if (msaAppId == null) {
throw new MissingRequiredPropertyException("BotPropertiesResponse", "msaAppId");
}
this.msaAppId = msaAppId;
return this;
}
@CustomType.Setter
public Builder msaAppMSIResourceId(@Nullable String msaAppMSIResourceId) {
this.msaAppMSIResourceId = msaAppMSIResourceId;
return this;
}
@CustomType.Setter
public Builder msaAppTenantId(@Nullable String msaAppTenantId) {
this.msaAppTenantId = msaAppTenantId;
return this;
}
@CustomType.Setter
public Builder msaAppType(@Nullable String msaAppType) {
this.msaAppType = msaAppType;
return this;
}
@CustomType.Setter
public Builder openWithHint(@Nullable String openWithHint) {
this.openWithHint = openWithHint;
return this;
}
@CustomType.Setter
public Builder parameters(@Nullable Map parameters) {
this.parameters = parameters;
return this;
}
@CustomType.Setter
public Builder privateEndpointConnections(List privateEndpointConnections) {
if (privateEndpointConnections == null) {
throw new MissingRequiredPropertyException("BotPropertiesResponse", "privateEndpointConnections");
}
this.privateEndpointConnections = privateEndpointConnections;
return this;
}
public Builder privateEndpointConnections(PrivateEndpointConnectionResponse... privateEndpointConnections) {
return privateEndpointConnections(List.of(privateEndpointConnections));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("BotPropertiesResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder publicNetworkAccess(@Nullable String publicNetworkAccess) {
this.publicNetworkAccess = publicNetworkAccess;
return this;
}
@CustomType.Setter
public Builder publishingCredentials(@Nullable String publishingCredentials) {
this.publishingCredentials = publishingCredentials;
return this;
}
@CustomType.Setter
public Builder schemaTransformationVersion(@Nullable String schemaTransformationVersion) {
this.schemaTransformationVersion = schemaTransformationVersion;
return this;
}
@CustomType.Setter
public Builder storageResourceId(@Nullable String storageResourceId) {
this.storageResourceId = storageResourceId;
return this;
}
@CustomType.Setter
public Builder tenantId(@Nullable String tenantId) {
this.tenantId = tenantId;
return this;
}
public BotPropertiesResponse build() {
final var _resultValue = new BotPropertiesResponse();
_resultValue.allSettings = allSettings;
_resultValue.appPasswordHint = appPasswordHint;
_resultValue.cmekEncryptionStatus = cmekEncryptionStatus;
_resultValue.cmekKeyVaultUrl = cmekKeyVaultUrl;
_resultValue.configuredChannels = configuredChannels;
_resultValue.description = description;
_resultValue.developerAppInsightKey = developerAppInsightKey;
_resultValue.developerAppInsightsApiKey = developerAppInsightsApiKey;
_resultValue.developerAppInsightsApplicationId = developerAppInsightsApplicationId;
_resultValue.disableLocalAuth = disableLocalAuth;
_resultValue.displayName = displayName;
_resultValue.enabledChannels = enabledChannels;
_resultValue.endpoint = endpoint;
_resultValue.endpointVersion = endpointVersion;
_resultValue.iconUrl = iconUrl;
_resultValue.isCmekEnabled = isCmekEnabled;
_resultValue.isDeveloperAppInsightsApiKeySet = isDeveloperAppInsightsApiKeySet;
_resultValue.isStreamingSupported = isStreamingSupported;
_resultValue.luisAppIds = luisAppIds;
_resultValue.luisKey = luisKey;
_resultValue.manifestUrl = manifestUrl;
_resultValue.migrationToken = migrationToken;
_resultValue.msaAppId = msaAppId;
_resultValue.msaAppMSIResourceId = msaAppMSIResourceId;
_resultValue.msaAppTenantId = msaAppTenantId;
_resultValue.msaAppType = msaAppType;
_resultValue.openWithHint = openWithHint;
_resultValue.parameters = parameters;
_resultValue.privateEndpointConnections = privateEndpointConnections;
_resultValue.provisioningState = provisioningState;
_resultValue.publicNetworkAccess = publicNetworkAccess;
_resultValue.publishingCredentials = publishingCredentials;
_resultValue.schemaTransformationVersion = schemaTransformationVersion;
_resultValue.storageResourceId = storageResourceId;
_resultValue.tenantId = tenantId;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy