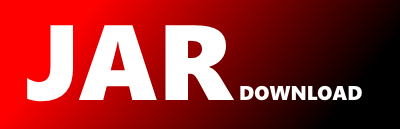
com.pulumi.azurenative.cdn.AFDOriginArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.cdn;
import com.pulumi.azurenative.cdn.enums.EnabledState;
import com.pulumi.azurenative.cdn.inputs.ResourceReferenceArgs;
import com.pulumi.azurenative.cdn.inputs.SharedPrivateLinkResourcePropertiesArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AFDOriginArgs extends com.pulumi.resources.ResourceArgs {
public static final AFDOriginArgs Empty = new AFDOriginArgs();
/**
* Resource reference to the Azure origin resource.
*
*/
@Import(name="azureOrigin")
private @Nullable Output azureOrigin;
/**
* @return Resource reference to the Azure origin resource.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy